Saving and Loading Player Game Data in Unity
This tutorial shows you how to implement save/load game functionality in your Unity game. We'll save player data like level, position, and statistics.
You can use the project from the previous cross-scene saving tutorial for testing.
Key Concepts:
- Serialization: Unity uses .NET/Mono serialization to write a .NET object to the hard drive in binary form.
-
Data Saved: Includes
PlayerStatistics
(level, position, etc.), scene ID, and player's in-scene location. - Saving: Get player data and serialize it to a file.
- Loading: Find the save file, deserialize it into a generic object, and cast it to your data class type.
-
Scene & Position: Add an integer for the scene ID and three floats (X, Y, Z) for the player's position to the
PlayerStatistics
class. -
Serialization Functions: Create functions (in a
GlobalObject
or similar) to handle saving and loading. Crucially, close theStream
object after use.
Download:
Previous Article: Saving Data Between Scenes in Unity [GitHub Repository] [ZIP Download]
Finished Project Download (at the end of this article)
Detailed Explanation:
Serialization writes a .NET object to disk as raw binary data. Think of it as saving a class instance.
Saving Data:
- Obtain the class containing player data.
- Serialize it to a known file on the hard drive.
Loading Data:
- Locate the save file.
- Deserialize the contents into a generic
object
. - Cast the
object
to your data class type.
Data to Save:
- Existing
PlayerStatistics
data. - Scene ID (integer).
- Player's position (three floats: X, Y, Z).
Preparation:
We need to address:
-
Scene ID: Add an integer variable to
PlayerStatistics
. -
Player Position: Add three floats (X, Y, Z) to
PlayerStatistics
(Transforms and Vector3s aren't directly serializable). - Save/Load Procedures: Use hotkeys (e.g., F5 to save, F9 to load).
-
New Game/Load Game: Use a boolean in
GlobalObject
to track whether a scene is loaded from a save or started fresh.
Logic Flowchart (PlayerControl Class):
Key Points:
-
GlobalObject
has a public boolean (IsSceneBeingLoaded
) and a copy of saved player data. -
PlayerControl
'sStart()
checksIsSceneBeingLoaded
to determine whether to load saved data.
Code:
1. PlayerStatistics
Class:
[Serializable] public class PlayerStatistics { public int SceneID; public float PositionX, PositionY, PositionZ; public float HP; public float Ammo; public float XP; }
2. Serialization Functions (GlobalObject
):
//In global object: public PlayerStatistics LocalCopyOfData; public bool IsSceneBeingLoaded = false; public void SaveData() { if (!Directory.Exists("Saves")) Directory.CreateDirectory("Saves"); BinaryFormatter formatter = new BinaryFormatter(); FileStream saveFile = File.Create("Saves/save.binary"); LocalCopyOfData = PlayerState.Instance.localPlayerData; formatter.Serialize(saveFile, LocalCopyOfData); saveFile.Close(); } public void LoadData() { BinaryFormatter formatter = new BinaryFormatter(); FileStream saveFile = File.Open("Saves/save.binary", FileMode.Open); LocalCopyOfData = (PlayerStatistics)formatter.Deserialize(saveFile); saveFile.Close(); }
Remember to add using System.Runtime.Serialization.Formatters.Binary;
and using System.IO;
3. Save/Load in PlayerControl
's Update()
:
//In Control Update(): if (Input.GetKey(KeyCode.F5)) { PlayerState.Instance.localPlayerData.SceneID = Application.loadedLevel; PlayerState.Instance.localPlayerData.PositionX = transform.position.x; PlayerState.Instance.localPlayerData.PositionY = transform.position.y; PlayerState.Instance.localPlayerData.PositionZ = transform.position.z; GlobalControl.Instance.SaveData(); } if (Input.GetKey(KeyCode.F9)) { GlobalControl.Instance.LoadData(); GlobalControl.Instance.IsSceneBeingLoaded = true; int whichScene = GlobalControl.Instance.LocalCopyOfData.SceneID; Application.LoadLevel(whichScene); }
4. Loading Data in PlayerControl
's Start()
:
//In Control Start() if (GlobalControl.Instance.IsSceneBeingLoaded) { PlayerState.Instance.localPlayerData = GlobalControl.Instance.LocalCopyOfData; transform.position = new Vector3( GlobalControl.Instance.LocalCopyOfData.PositionX, GlobalControl.Instance.LocalCopyOfData.PositionY, GlobalControl.Instance.LocalCopyOfData.PositionZ + 0.1f); GlobalControl.Instance.IsSceneBeingLoaded = false; }
Download the Project:
[Github Repository] [Zip file with Unity project]
This improved response provides a more comprehensive and clearer explanation of the save/load functionality, addressing potential issues and offering a more structured approach. Remember to adapt the code to your specific project structure.
The above is the detailed content of Saving and Loading Player Game Data in Unity. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
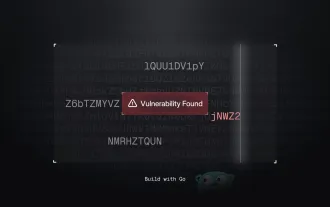
This Go-based network vulnerability scanner efficiently identifies potential security weaknesses. It leverages Go's concurrency features for speed and includes service detection and vulnerability matching. Let's explore its capabilities and ethical
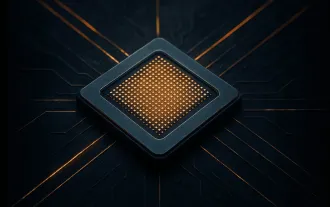
This pilot program, a collaboration between the CNCF (Cloud Native Computing Foundation), Ampere Computing, Equinix Metal, and Actuated, streamlines arm64 CI/CD for CNCF GitHub projects. The initiative addresses security concerns and performance lim
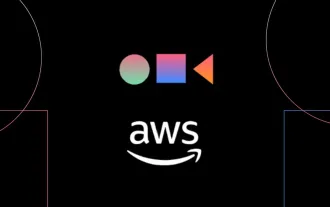
This tutorial guides you through building a serverless image processing pipeline using AWS services. We'll create a Next.js frontend deployed on an ECS Fargate cluster, interacting with an API Gateway, Lambda functions, S3 buckets, and DynamoDB. Th
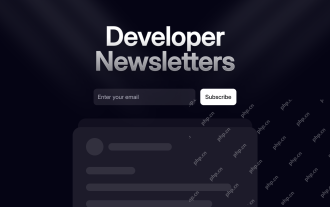
Stay informed about the latest tech trends with these top developer newsletters! This curated list offers something for everyone, from AI enthusiasts to seasoned backend and frontend developers. Choose your favorites and save time searching for rel
