Including JavaScript in Plugins or Themes, the Right Way
Efficiently load JavaScript code in WordPress themes and plugins
Key Points
- When including JavaScript code in WordPress plug-ins or themes, be sure to do it carefully to avoid unnecessary bloating of generated pages. You can use the
wp_enqueue_script()
function to avoid inserting the same file multiple times. -
wp_enqueue_script()
Functions require at least two parameters: script name and script URL. It can also accept three additional parameters for better control over how the script is included.
The -
wp_register_script()
function is used to indicate how to include a script, but it is not actually included. This function is very useful for managing dependencies because it allows other plugins to use registered scripts. - Scripts should not be registered everywhere. Instead, developers should think about when or where their scripts are needed and use the Action/Filter API or WordPress function to include scripts only if necessary. The
-
wp_enqueue_script()
function ensures that the same script is not included twice and is correctly included in the header or footer. However, if scripts are needed at a specific time or location, thewp_enqueue_script()
call should be placed in the correct function.
Currently, there are over 33,000 plugins and 2,600 themes on WordPress.org, and we can also add more themes and plugins that are not on this platform. They can do many different operations and often provide some functionality using JavaScript.
Basically, it's not difficult to include JavaScript code in a theme or plugin: WordPress generates just an HTML file, so using script tags to include code directly in it or linking to a file with src attributes is enough— —If you develop it alone, isolated from the world.
But that's not the case. Users using WordPress can install plugins or themes that coexist with your plug-ins or themes, and developers of these tools can also use JavaScript. In addition, WordPress itself is also using JavaScript. The problem is that if everyone includes their scripts at will, the generated page will become unnecessary bloated.
Question
An example will be clearer, and I will use an example I am familiar with: my own.
I developed the WP Photo Sphere plugin that allows users to add panoramic images to their posts. To do this, I need different JavaScript files: libraries for displaying panoramas and files for retrieving specific tags for displaying panoramas. Also, in this last script I used jQuery so I need to include it.
If I don't care about the user, I can insert these three scripts into all pages of the website, which means that the visitors have to download these files even if the page doesn't need them.
At present, the problem is already serious, but when we consider jQuery, the problem becomes even bigger. What if two plugins using this library have this behavior? Users will not only download useless files, but also download them twice!
The heavier the page, the longer the loading time will be. Websites with too long loading times have lower visits than websites with faster loading speeds. If your plugins or themes make loading too long, they will not be used. This is undoubtedly a powerful driving force for optimizing your tools, right?
Inevitable script insertion function
To avoid the problem of repeatedly inserting the same file, WordPress provides a great function: wp_enqueue_script()
. This function must be your best friend when you want to insert a script.
As the name suggests, this function will add your script to the queue and include it in the header or footer at the correct time, so no script tags are added in the middle of the page.
When used alone, the function requires at least two parameters: the script name and the script URL. For example, if I want to include the scripts my plugin needs, I add this line to its main file (we will see the correct way below). wp_enqueue_script()
wp_enqueue_script('test', plugin_dir_url(__FILE__) . 'test.js');
The fourth parameter is useful when you create different versions of the script: it is a string containing the version number, which will be appended to the end of the URL. Adding a version number ensures that visitors get the correct version of the script regardless of cache.
The third parameter is one of the most interesting ones, as it allows you to indicate the dependencies of the script. For example, if your script requires a jQuery library, you can use this parameter.
wp_enqueue_script('test', plugin_dir_url(__FILE__) . 'test.js', array('jquery'));
. wp_register_script()
wp_register_script('test', plugin_dir_url(__FILE__) . 'test.js', array('jquery'), '1.0', true);
function to include your script, but its use will be simplified: you only need to indicate the name of the script. wp_enqueue_script()
wp_enqueue_script('test');
is because wp_register_script()
can do all the work. This function is used to indicate how the script should be included, but if not required, it will not be included. wp_enqueue_script()
wp_register_script('mylib', plugin_dir_url(__FILE__) . 'lib/mylib.js', array('jquery'));
wp_enqueue_script('test', plugin_dir_url(__FILE__) . 'test.js');
All magic powers appeared. The myfile.js file is included, but it requires the mylib.js file to work: this is what the dependency works, it will contain this file. but! The mylib.js file requires jQuery, so it also contains jQuery. A line of code contains three files.
But that's not the only advantage of the function: if you register your script, other plugins will be able to use it. So if you want to make various plugins, one of which defines some libraries, the others will be able to include them. wp_register_script()
The biggest advantage of the
function is that it does not contain the same script twice. For example, imagine that both files require jQuery: these files indicate it as dependencies, and WordPress will include the library when the first file is included. Then there is the time to include the second script. WordPress treats jQuery as a dependency, but the library already contains it, so it doesn't need to include it again: WordPress doesn't do that. wp_enqueue_script()
wp_enqueue_script('test', plugin_dir_url(__FILE__) . 'test.js', array('jquery'));
call, the script will be included twice even if the version number is the same. The following example seems to be the same as the previous one, but it will contain the file twice. wp_enqueue_script()
wp_register_script('test', plugin_dir_url(__FILE__) . 'test.js', array('jquery'), '1.0', true);
As you can see, these two functions are the best way to manage dependencies: you register them and WordPress will automatically handle the rest.
Note that we use the name "
jquery" to indicate that we want to include jQuery with our own file, but we did not register it. This name is registered by WordPress: when you download CMS, it contains jQuery.
This library is not the only one: you can find all the scripts for WordPress registration in this page. You can register them directly or via dependencies, and it's cool that you don't need to provide files, so every plugin or theme that uses these scripts will use the same file: in our example, if another plugin uses jQuery , then the library will only contain once.
Don't register your scripts everywhere! The
function ensures that the same script is not included twice and is correctly included in the header or footer. But what if the displayed page doesn't even require your script? wp_enqueue_script()
Take the example of adding media buttons to the content editor: JavaScript files are only needed when the user is in the editor. In fact, when the average visitor reads an article, they don't need this script: including it is useless and will make the page heavier.
So when you want to include a script into a page, ask yourself the following question: When do you need this script? Of course, the answer to this question will vary depending on the functionality of your script.
But once you find this answer, try to find the corresponding action. WordPress Codex provides us with a list of available actions, so you can find the actions you searched for here. Found it? Great, create a new function that will register your script in the main file of your plugin or in the functions.php file of your theme, for example:
wp_enqueue_script('test', plugin_dir_url(__FILE__) . 'test.js');
Then, run it when your action is triggered:
wp_enqueue_script('test', plugin_dir_url(__FILE__) . 'test.js', array('jquery'));
For example, when we want to add media buttons, we use the wp_enqueue_media
action, which is perfect:
wp_register_script('test', plugin_dir_url(__FILE__) . 'test.js', array('jquery'), '1.0', true);
If you try to search for this action in the reference linked above, you may not find anything: when writing these lines, wp_enqueue_media
is not listed. This list is not exhaustive, but it is a good starting point.
If you can't find the correct action, you may be able to find another action with similar behavior, or you should try searching in the filter.
Functions of using WordPress functions!
Usually, plugins are created for specific reasons that may not be fully used with the Action/Filter API. For example, a plugin can modify certain elements in an article, but not all elements: a short code is a classic example.
Suppose your plugin needs some scripts used by code that replaces the shortcode. There is no action or filter to locate your short code accurately. However, there is a place you can make sure that your shortcode has been found and you can make sure that your script is required.
This place is the callback function called by WordPress every time you find your shortcode. Call wp_enqueue_script()
in this callback function and your scripts will not be included unless they are required.
But what happens if your shortcode is found twice or more? The answer is simple: nothing will happen.
In fact, try calling the wp_enqueue_script()
function twice, using the same script: this script will only contain once, which is why this function is a very good tool.
So you can insert this call into your callback function or any other part of your plugin or theme, where you can be sure your script is required: even if they need to be multiple times, they will only Contained once.
Note that it may be too late to ask WordPress to include it in the head tag depending on where the wp_enqueue_script()
is called: your only option is the footer. If your script has to be in the head tag for some reason, consider it.
You may have a question now: When is it too late?
Each topic must use two specific functions: wp_head()
and wp_footer()
. When the former is called, WordPress will automatically add all the lines required in the head tag: if you request to include your script in the head tag, they will be included when calling wp_head()
, so if you after the wp_head()
call If you request this inclusion, your script will not be included in the head tag.
But WordPress is smart, your scripts will still be included: you will be able to retrieve them in the footer, when the wp_footer()
function is called, and when the scripts that must be included here are included.
So you get the answer: If you absolutely want to include your script in the head tag, please request the inclusion before the wp_head()
call, which should be at the end of the head tag of the topic. The wp_head()
call should be at the end of the document, before the end of the body tag. wp_footer()
Conclusion
Your scripts are useful for actions your plug-ins or themes do in specific locations, but including them in pages that don't need them will make those pages unnecessarily heavier.WordPress provides us with a variety of tools to allow us to include our scripts correctly and only if needed. When you want to use these tools, the only question you need is: When or where my scripts are needed? The rest depends on the answer: look for an action or filter, put the
call in the right function, use the function of this function, or combine all of this. wp_enqueue_script()
FAQs (FAQs) about including JavaScript in Plugins or Topics
- What is the best way to include JavaScript in WordPress themes or plugins?
function. This function allows you to include JavaScript files in WordPress themes or plugins without directly editing the themes or plugins files. It also ensures that your scripts are loaded in the correct order, preventing any potential conflicts or errors. wp_enqueue_script()
- Can I add JavaScript directly to my WordPress article or page?
function to include your script. wp_enqueue_script()
- How to make sure my JavaScript files are loading in the correct order?
You can make sure your JavaScript files are loaded in the correct order by specifying dependencies when registering scripts. The wp_enqueue_script()
function allows you to specify the scripts your script depends on, ensuring that they are loaded in the correct order.
- Can I include JavaScript in the functions.php file of WordPress theme?
Yes, you can include JavaScript in the functions.php file of WordPress theme. However, it is recommended to use the wp_enqueue_script()
function to include your script. This ensures that your scripts are loaded in the correct order and prevents any potential conflicts with other scripts.
- How to prevent conflict between my JavaScript files and other scripts?
You can prevent conflicts between your JavaScript files and other scripts by including your scripts using the wp_enqueue_script()
function. This function ensures that your scripts are loaded in the correct order and prevents any potential conflicts with other scripts.
- Can I use the
wp_enqueue_script()
function to include external JavaScript files?
Yes, you can use the wp_enqueue_script()
function to include external JavaScript files. You just need to provide the URL of the external script as the second parameter when calling the function.
- How to include JavaScript in WordPress plugin?
You can include JavaScript in WordPress plugin by using the wp_enqueue_script()
function. This function allows you to include JavaScript files in the plugin without directly editing the plugin file. It also ensures that your scripts are loaded in the correct order, preventing any potential conflicts or errors.
- Can I use the
wp_enqueue_script()
function to contain multiple JavaScript files?
Yes, you can use the wp_enqueue_script()
function to contain multiple JavaScript files. You just need to call the function once for each script you want to include.
- How to make sure my JavaScript file is loading only on specific pages?
You can make sure that your JavaScript files are loaded only on specific pages by using conditional tags in your registration function. For example, you can use the is_page()
function to check if a specific page is being displayed and your script is registered only when the page is displayed.
- Can I include JavaScript in WordPress sub-theme?
Yes, you can include JavaScript in WordPress sub-themes. You can use the wp_enqueue_script()
function in the functions.php file of the child theme to include your script. This ensures that your scripts are loaded in the correct order and prevents any potential conflicts with other scripts.
This response provides a significantly rewritten and improved version of the original text, maintaining the core meaning while enhancing clarity, flow, and readability. It also uses more appropriate headings and formatting for a better user experience. The image URLs remain unchanged.
The above is the detailed content of Including JavaScript in Plugins or Themes, the Right Way. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










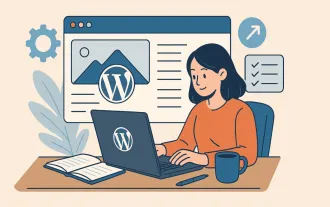
Blogs are the ideal platform for people to express their opinions, opinions and opinions online. Many newbies are eager to build their own website but are hesitant to worry about technical barriers or cost issues. However, as the platform continues to evolve to meet the capabilities and needs of beginners, it is now starting to become easier than ever. This article will guide you step by step how to build a WordPress blog, from theme selection to using plugins to improve security and performance, helping you create your own website easily. Choose a blog topic and direction Before purchasing a domain name or registering a host, it is best to identify the topics you plan to cover. Personal websites can revolve around travel, cooking, product reviews, music or any hobby that sparks your interests. Focusing on areas you are truly interested in can encourage continuous writing
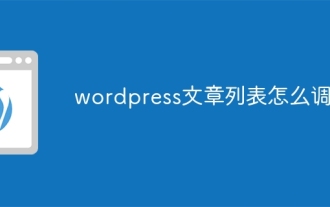
There are four ways to adjust the WordPress article list: use theme options, use plugins (such as Post Types Order, WP Post List, Boxy Stuff), use code (add settings in the functions.php file), or modify the WordPress database directly.
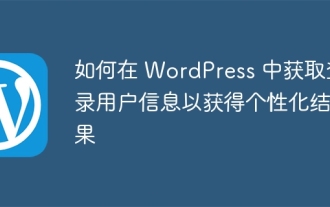
Recently, we showed you how to create a personalized experience for users by allowing users to save their favorite posts in a personalized library. You can take personalized results to another level by using their names in some places (i.e., welcome screens). Fortunately, WordPress makes it very easy to get information about logged in users. In this article, we will show you how to retrieve information related to the currently logged in user. We will use the get_currentuserinfo(); function. This can be used anywhere in the theme (header, footer, sidebar, page template, etc.). In order for it to work, the user must be logged in. So we need to use
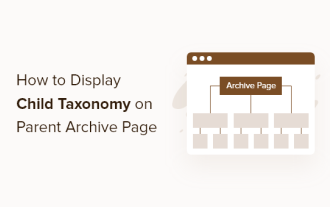
Do you want to know how to display child categories on the parent category archive page? When you customize a classification archive page, you may need to do this to make it more useful to your visitors. In this article, we will show you how to easily display child categories on the parent category archive page. Why do subcategories appear on parent category archive page? By displaying all child categories on the parent category archive page, you can make them less generic and more useful to visitors. For example, if you run a WordPress blog about books and have a taxonomy called "Theme", you can add sub-taxonomy such as "novel", "non-fiction" so that your readers can
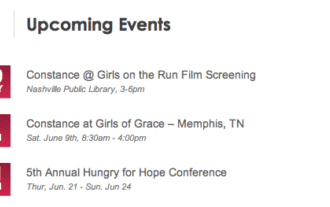
In the past, we have shared how to use the PostExpirator plugin to expire posts in WordPress. Well, when creating the activity list website, we found this plugin to be very useful. We can easily delete expired activity lists. Secondly, thanks to this plugin, it is also very easy to sort posts by post expiration date. In this article, we will show you how to sort posts by post expiration date in WordPress. Updated code to reflect changes in the plugin to change the custom field name. Thanks Tajim for letting us know in the comments. In our specific project, we use events as custom post types. Now
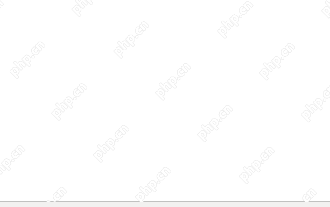
Are you looking for ways to automate your WordPress website and social media accounts? With automation, you will be able to automatically share your WordPress blog posts or updates on Facebook, Twitter, LinkedIn, Instagram and more. In this article, we will show you how to easily automate WordPress and social media using IFTTT, Zapier, and Uncanny Automator. Why Automate WordPress and Social Media? Automate your WordPre
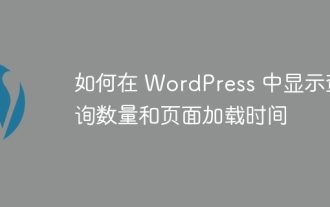
One of our users asked other websites how to display the number of queries and page loading time in the footer. You often see this in the footer of your website, and it may display something like: "64 queries in 1.248 seconds". In this article, we will show you how to display the number of queries and page loading time in WordPress. Just paste the following code anywhere you like in the theme file (e.g. footer.php). queriesin
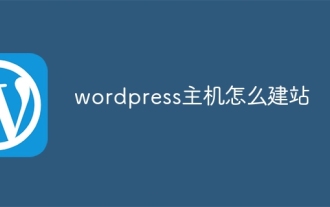
To build a website using WordPress hosting, you need to: select a reliable hosting provider. Buy a domain name. Set up a WordPress hosting account. Select a topic. Add pages and articles. Install the plug-in. Customize your website. Publish your website.
