Using the Google Analytics API with PHP: Logging In
This series demonstrates using the Google Analytics API with PHP to access Google Analytics data. While Laravel and Homestead Improved are used in the example, the concepts apply to other frameworks and environments.
Key Concepts:
- The Google Analytics API requires a Google Developers Console project with the Google Analytics API enabled. API credentials (client ID, client secret, developer key) are essential.
- The API comprises several components: Management API (account, property, view configuration), Metadata API (dimension, metric lists), Core Reporting API (dashboard data), Real Time Reporting API (real-time data – beta), Embed API (dashboard embedding), and MCF Reporting API (multi-channel funnels). This tutorial focuses on the Management, Metadata, and Core Reporting APIs.
- The
Google_Client
class handles authentication and token retrieval. It requires configuration with client ID, client secret, developer key, redirect URI, and scopes. - API usage is subject to quotas (requests per day/second). Monitor usage via the Google Cloud Console.
Prerequisites:
- A Google Analytics account.
- Familiarity with the Google Analytics dashboard.
Application Overview:
This tutorial builds a simplified Google Analytics Explorer clone, focusing on core functionality and extensibility.
Google Analytics API Details:
The Google Analytics API's key components are:
- Management API: Accesses Google Analytics configuration data (accounts, properties, views, goals).
- Metadata API: Retrieves lists of dimensions and metrics, avoiding hardcoding.
- Core Reporting API: Accesses dashboard data; the primary API for most tasks.
- Real Time Reporting API (Beta): Accesses real-time data (currently in beta).
- Embed API: Allows embedding dashboards in websites using JavaScript.
- MCF Reporting API: Provides Multi-Channel Funnels data.
This tutorial uses the Management, Metadata, and Core Reporting APIs.
Basic API Usage:
- Create a project in the Google Developers Console.
- Enable the Google Analytics API.
- Obtain API credentials (client ID, client secret, developer key). Create a new client ID (Web Application type), specifying your website URL (e.g.,
localhost:8000
for development) and redirect URI. Generate a browser key (optional: specify HTTP referrers or leave blank for any origin).
API Limits and Quotas:
Be aware of API request limits (per day, per second). Refer to the official documentation for details.
Project Setup (Laravel Example):
- Add
"google/api-client": "dev-master"
tocomposer.json
and runcomposer update
. - Create
app/config/analytics.php
with API credentials:
1 2 3 4 5 6 |
|
- Create
app/src/GA_Service.php
for API interaction logic:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
|
-
Add
app/src
to theautoload
->classmap
incomposer.json
and runcomposer dump-autoload
. -
Modify
app/controllers/HomeController.php
(or your equivalent controller):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
|
- Update your routes in
app/routes.php
:
1 2 |
|
- Create a
login.blade.php
view:
1 |
|
This completes the basic setup. The next steps would involve adding functions to GA_Service.php
to actually retrieve data using the Google Analytics API. Remember to handle potential errors appropriately. The provided code is a foundation upon which to build a more complete application. Consult the Google Analytics API documentation for details on making specific data requests.
The above is the detailed content of Using the Google Analytics API with PHP: Logging In. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
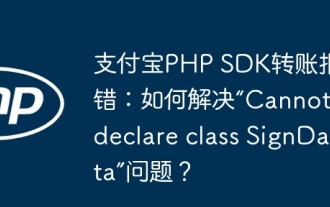
Alipay PHP...
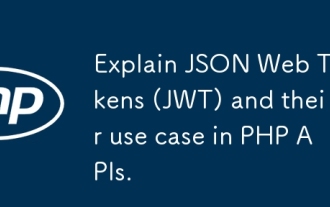
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
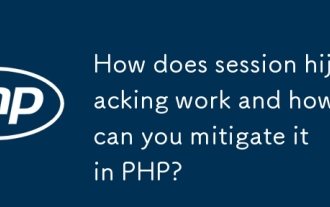
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
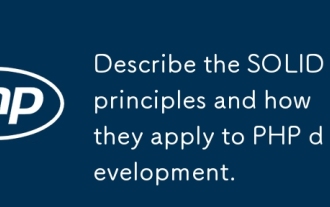
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
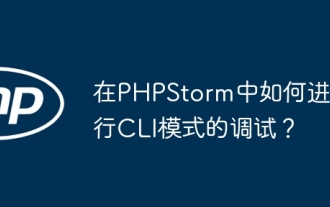
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
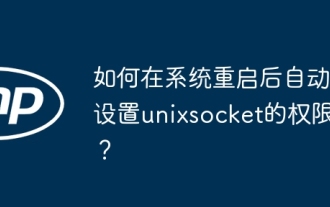
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
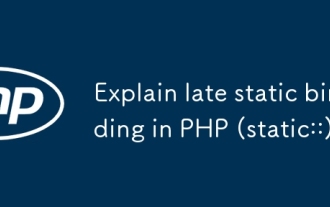
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
