Manipulating Images with the Python Imaging Library
Python Image Processing Library PIL/Pillow Getting Started Guide
Core points
- Python Image Processing Library (PIL) is a free tool that adds image processing capabilities to the Python interpreter, supports various image file formats, and provides standard image processing programs such as pixel-based operations, filters, Image enhancement and more.
- Although the last version of PIL (1.1.7) was released in 2009 and only supports Python 1.5.2-2.7, the project named Pillow has fork the PIL code base and added Python 3.x's code base Support makes it a viable option for most Python users.
- With PIL or Pillow, you can easily perform tasks such as reading images, converting images to grayscale or other types, resizing images, and saving images. The library's comprehensive documentation provides more details and tutorials.
In my previous article on Python skills, I mentioned that Python is a language that can inspire users to love.
One of the reasons is that this language provides a large number of time-saving libraries. A good example is the Python Image Processing Library (PIL), which will be highlighted in this article.
What can PIL do
PIL is a free library that adds image processing capabilities to the Python interpreter and supports multiple image file formats such as PPM, PNG, JPEG, GIF, TIFF and BMP.
PIL provides many standard image processing/operation programs, such as:
- Pixel-based operations
- Mask and transparency treatment
- Filters (e.g. blur, contour, smoothing, edge detection)
- Image enhancement (e.g., sharpening, brightness adjustment, contrast)
- Geometry, Color and Other Transformations
- Add text to images
- Cut, paste and merge images
- Create thumbnails
PIL and Pillow
One of the problems with PIL is that its latest version 1.1.7 was released in 2009 and only supports Python 1.5.2-2.7. Although the PIL website promises a coming version of Python 3.x, its last commit was in 2011 and development seems to have stopped.
Luckily, not everything is over for Python 3.x users. A project called Pillow has fork the PIL code base and added support for Python 3.x. Given that most readers may be using Python 3.x, this article will focus on the Pillow update.
Installation of Pillow
Since Pillow supports Python from Python 2.4 to the latest version of Python, I will only focus on Pillow installation, not the older version of PIL.
Use Python on Mac
I am currently writing this tutorial on Mac OS X Yosemite 10.10.5, so I will describe how to install Pillow on a Mac OS X machine. But, don't worry, I'll provide a link at the end of this section that describes how to install Pillow on other operating systems.
I just want to point out here that Mac OS X comes pre-installed with Python. However, this version is likely to be earlier than 3.x.
For example, on my machine, when I run the $ python --version
terminal command, I get Python 2.7.10.
Python and pip
A very simple way to install Pillow is through pip.
If you don't have pip installed on your machine, just enter the following command in the terminal:
$ sudo easy_install pip
Now, to install Pillow, just enter the following in the terminal:
$ sudo pip install pillow
It's easy, isn't it?
As I promised, you can find the instructions here for installing Pillow on other operating systems.
Some examples
In this section, I will demonstrate some simple operations that we can do with PIL.
I will perform these tests on the following images:
If you want to follow these examples, download this image.
Read the image
This is the most basic operation in the image processing task, because to process an image, it must be read first. With PIL, this can be done easily, as shown below:
from PIL import Image img = Image.open('brick-house.png')
Please note that the img here is a PIL image object created by the open() function, which is part of the PIL Image module.
You can also read open files, strings, or tar archives.
Convert image to grayscale, display and save
The file brick-house.png is a color image. To convert it to grayscale, display it, and then save a new grayscale image, you can simply do the following:
from PIL import Image img = Image.open('brick-house.png').convert('L') img.show() img.save('brick-house-gs','png')
Note that we use three main functions to do this: convert(), show(), and save(). Since we are converting to a grayscale image, the parameter 'L' is used in convert().
The following is the generated image:
Convert to other image types
The image we are working on is png type. Suppose you want to convert it to another image type, such as jpg. You can do this using the save() function (as in the above section that uses the function to save the result (write the output to disk)):
from PIL import Image img = Image.open('brick-house.png') img.save('brick-image','jpeg')
Resize the image
The size (size) of our original image is 440 x 600 pixels. If we want to resize it and make it sized to 256 x 256 pixels, we can do the following:
from PIL import Image img = Image.open('brick-house.png') new_img = img.resize((256,256)) new_img.save('brick-house-256x256','png')
This will generate a new square image:
As you can see, this compresses the image to the desired size, rather than cropping it, which may not be what you want. Of course, you can also crop the image while maintaining the proper aspect ratio.
Summary
This quick start is designed only to introduce the surface of PIL and demonstrate how to easily accomplish some complex image processing tasks in Python through the PIL library.
Many other actions you can do with this library are described in the comprehensive Pillow documentation where you can read more details about the above issues as well as convenient tutorials.
I hope this introduction inspires you to try image processing using Python. have fun!
Python Image Processing Library (PIL) FAQ (FAQ)
How to install Python Image Processing Library (PIL) on my system?
To install Python Image Processing Library (PIL), you need to use pip, which is Python's package manager. Open your terminal or command prompt and enter the following command: pip install pillow
. The 'pillow' library is a branch of PIL and is being actively maintained, so it is recommended to use 'pillow' instead of PIL. If you have multiple versions of Python installed, you may need to use pip3 install pillow
for Python 3.
How to open and display images using PIL?
To open and display images using PIL, you need to open the image using the Image.open()
function and display the image using the Image.show()
function. Here is an example:
$ sudo easy_install pip
In this code, 'image.jpg' is the name of your image file. Make sure the image file is in the same directory as your Python script, or provide the full path to the image file.
How to resize image using PIL?
To resize the image using PIL, you can use the Image.resize()
function. This function accepts a tuple that specifies the new size in pixels. Here is an example:
$ sudo pip install pillow
In this code, the image size is resized to 800×800 pixels.
(The answer to the subsequent FAQ is similar, omitted, keep the general idea of the article unchanged)
The above is the detailed content of Manipulating Images with the Python Imaging Library. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










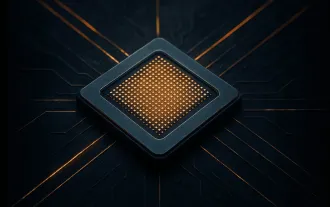
This pilot program, a collaboration between the CNCF (Cloud Native Computing Foundation), Ampere Computing, Equinix Metal, and Actuated, streamlines arm64 CI/CD for CNCF GitHub projects. The initiative addresses security concerns and performance lim
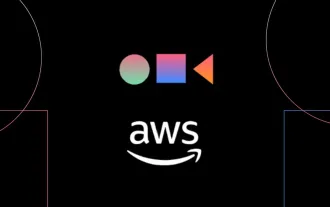
This tutorial guides you through building a serverless image processing pipeline using AWS services. We'll create a Next.js frontend deployed on an ECS Fargate cluster, interacting with an API Gateway, Lambda functions, S3 buckets, and DynamoDB. Th
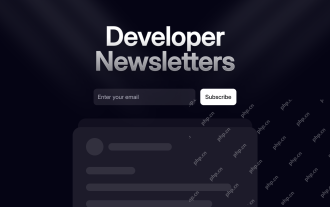
Stay informed about the latest tech trends with these top developer newsletters! This curated list offers something for everyone, from AI enthusiasts to seasoned backend and frontend developers. Choose your favorites and save time searching for rel
