Building a Chat Application with SignalR
This tutorial demonstrates building a simple chat application using SignalR, an open-source library for ASP.NET developers. SignalR provides real-time web functionality, similar to Socket.IO for Node.js. While Socket.IO might be preferred with JavaScript frameworks like Ember, SignalR offers extensive documentation and leverages the benefits of the ASP.NET ecosystem. This tutorial assumes familiarity with Ember.js.
Key Concepts:
- SignalR: A powerful library for adding real-time capabilities to ASP.NET applications. It simplifies the process of building interactive web applications.
- Ember.js Components: Reusable UI elements that encapsulate functionality and improve code organization. This tutorial utilizes components for the chat room, user list, chat area, and individual user items.
- Ember Controllers and Models: Manage application logic and data binding, respectively, providing a structured approach to handling chat data.
- SignalR Hubs: Server-side components that facilitate communication between the server and clients. This tutorial uses a "Lobby" hub to manage user connections and messages.
- CORS (Cross-Origin Resource Sharing): Addressing potential issues when the server and client reside on different domains. Proper CORS configuration is crucial for successful communication.
Getting Started with Ember CLI:
- Create a new Ember application:
ember new chatr
- Install dependencies:
ember install semantic-ui-ember
(Semantic UI provides a responsive layout framework). - Import Semantic UI: Add the following lines to
Brocfile.js
:
app.import('bower_components/semantic-ui/dist/semantic.css'); app.import('bower_components/semantic-ui/dist/semantic.js');
- Generate Ember routes and components:
ember g route chat ember g component chat-room ember g component chat-userlist ember g component chat-area ember g component chat-useritem ember g controller chat ember g model chat-room ember g model chat-user ember g model chat-message
- Integrate the
chat-room
component into thechat
route's template (app/templates/chat.hbs
):
{{#chat-room users=room.users messages=room.messages topic=room.topic onSendChat="sendChat"}}{{/chat-room}}
Server-Side Development with SignalR (using Visual Studio):
- Create a new Empty Web Application project in Visual Studio.
- Install the SignalR package:
Install-Package Microsoft.AspNet.Signalr
- Create the OWIN startup class (
App_Start/Startup.cs
):
public class Startup { public void Configuration(IAppBuilder app) { app.MapSignalR(); } }
- Create the Lobby Hub (
Lobby.cs
): This hub handles user connections, message broadcasting, and user management. (Implementation details omitted for brevity, but the original response provides the code.)
Client-Side Integration with Ember and SignalR:
- Install SignalR using Bower:
bower install signalr --save
- Import SignalR into your Ember application (in
Brocfile.js
):
app.import('bower_components/semantic-ui/dist/semantic.css'); app.import('bower_components/semantic-ui/dist/semantic.js');
- Include the SignalR hubs script in
app/index.html
:
ember g route chat ember g component chat-room ember g component chat-userlist ember g component chat-area ember g component chat-useritem ember g controller chat ember g model chat-room ember g model chat-user ember g model chat-message
-
Create an Ember initializer to manage the SignalR connection (
app/initializers/signalr.js
): (Implementation details omitted for brevity, but the original response provides the code.) This initializer uses aSignalRConnection
utility class to simplify SignalR interaction. -
Handle CORS: Install the
Microsoft.Owin.Cors
NuGet package and configure CORS middleware in yourStartup.cs
file to allow cross-domain requests from your Ember application. (Implementation details are in the original response.)
Conclusion:
This revised response provides a more concise and structured overview of the process, highlighting the key components and steps involved in building a real-time chat application using SignalR and Ember. The original response contains the detailed code snippets for each component, which are omitted here for brevity but are readily available in the original output. Remember to consult the original response for the complete code implementation.
The above is the detailed content of Building a Chat Application with SignalR. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
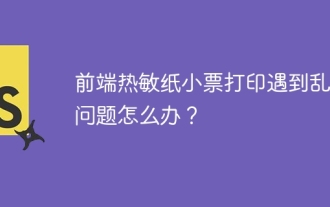
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
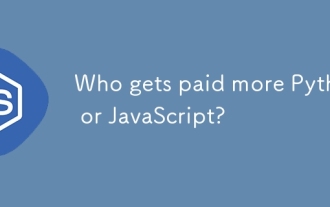
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
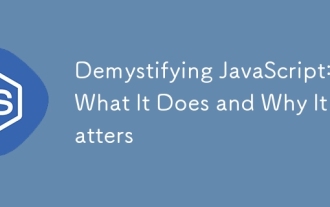
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
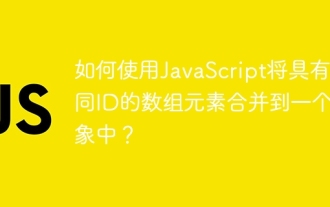
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
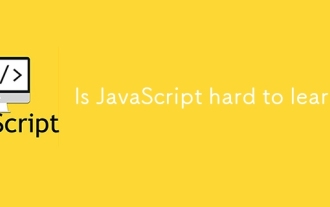
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
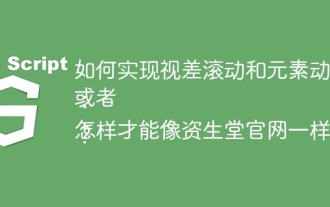
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
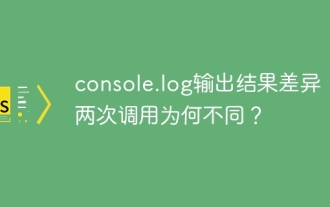
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
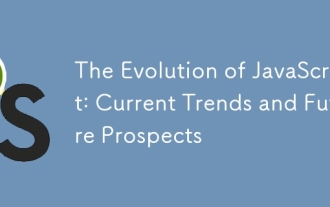
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
