Getting Started with Symfony2 Route Annotations
Core points
- Symfony2's SensioFrameworkExtraBundle allows developers to route configuration directly in the controller using annotations, providing a convenient alternative.
- Routing annotations in Symfony2 make routing configuration more modular, and each route is directly defined in its corresponding controller operations, making the code easier to understand and maintain.
- Annotations allow detailed routing configurations, including setting URL default parameters, adding requirements, enforcing HTTP methods or schemes, and enforcing hostnames.
- While using annotations may make controller operations more complicated, as they now include routing configurations, this can be mitigated by keeping routing simple and well documented.
The standard Symfony 2 distribution contains a practical bundle called SensioFrameworkExtraBundle, which implements many powerful features, especially the ability to use annotations directly in the controller.
This article aims to introduce a convenient alternative to controller configuration, and is not a mandatory way of annotation. The best approach depends on the specific scenario requirements.
Symfony 2 manages all routing for applications with powerful built-in components: routing components. Basically, the route maps the URL to the controller action. Since Symfony is designed to be modular, a file is specially set up for this: routing.yml
, located in app > config > routing.yml
.
To understand how to define routes using annotations, let's take a simple blog application as an example.
Step 1: Create a homepage route
We link the path to an operation of /
. HomeController
No annotation method
In: app/config/routing.yml
blog_front_homepage: path : / defaults: { _controller: BlogFrontBundle:Home:index }
: src/Blog/FrontBundle/Controller/HomeController.php
<?php namespace Blog\FrontBundle\Controller; class HomeController { public function indexAction() { //... 创建并返回一个 Response 对象 } }
, we declare a simple routing.yml
routing configuration with two parameters: the path and the controller operation to be located. The controller itself does not require any special settings. blog_front_homepage
Using annotations
In: app/config/routing.yml
blog_front: resource: "@BlogFrontBundle/Controller/" type: annotation prefix: /
: src/Blog/FrontBundle/Controller/HomeController.php
<?php namespace Blog\FrontBundle\Controller; use Sensio\Bundle\FrameworkExtraBundle\Configuration\Route; class HomeController { /** * @Route("/", name="blog_home_index") */ public function indexAction() { /* ... */ } }
Centre: routing.yml
resource
Specify the controller to affecttype
Define how to declare routesprefix
Define prefixes for all operations in the controller class (optional)
More important is how the controller is built. First, we must call the relevant class of SensioFrameworkExtraBundle: use SensioBundleFrameworkExtraBundleConfigurationRoute;
. Then we can implement the route and its parameters: here only the path and name (we will see all the operations that can be performed later): @Route("/", name="blog_homepage")
.
One might think: "We know how to overwrite the controller with the routing layer, so what's the point? Ultimately, more code is needed to get the same result." At least for the moment.
Step 2: Add article page route
No annotation method
In app/config/routing.yml
:
blog_front_homepage: path : / defaults: { _controller: BlogFrontBundle:Home:index }
In src/Blog/FrontBundle/Controller/HomeController.php
:
<?php namespace Blog\FrontBundle\Controller; class HomeController { public function indexAction() { //... 创建并返回一个 Response 对象 } }
Using annotations
In app/config/routing.yml
:
blog_front: resource: "@BlogFrontBundle/Controller/" type: annotation prefix: /
In src/Blog/FrontBundle/Controller/HomeController.php
:
<?php namespace Blog\FrontBundle\Controller; use Sensio\Bundle\FrameworkExtraBundle\Configuration\Route; class HomeController { /** * @Route("/", name="blog_home_index") */ public function indexAction() { /* ... */ } }
Note: routing.yml
No changes are required. Now you can see at a glance which operation is being called from routing mode.
If you want all operations in the controller to be prefixed, such as /admin
, you can remove the routing.yml
key from prefix
and add an extra @Route
to the top of the class Note:
In app/config/routing.yml
:
blog_front_homepage: path : / defaults: { _controller: BlogFrontBundle:Home:index } blog_front_article: path : /article/{slug} defaults: { _controller: BlogFrontBundle:Home:showArticle }
In src/Blog/AdminBundle/Controller/AdminController.php
:
<?php // namespace & uses... class HomeController { public function indexAction() { /* ... */ } public function showArticleAction($slug) { /* ... */ } }
Step 3: Additional routing configuration
Set URL default parameters
Chemism: defaults = { "key" = "value" }
.
blog_front: resource: "@BlogFrontBundle/Controller/" type: annotation prefix: /
The slug
placeholder is no longer required by adding defaults
to the {slug}
key. The URL /article
will match this route, and the value of the slug
parameter will be set to hello
. The URL /blog/world
will also match and set the value of the page
parameter to world
.
Add requirements
Chemism: requirements = { "key" = "value" }
.
<?php // namespace & uses... class HomeController { /** * @Route("/", name="blog_home_index") */ public function indexAction() { /* ... */ } /** * @Route("/article/{slug}", name="blog_home_show_article") */ public function showArticleAction($slug) { /* ... */ } }
We can use regular expressions to define requirements for the slug
key, and clearly define the value of {slug}
must consist of only alphabetical characters. In the following example, we do the exact same thing with the number:
blog_front: ... blog_admin: resource: "@BlogAdminBundle/Controller/" type: annotation
Enforce HTTP method
Chemism: methods = { "request method" }
.
blog_front_homepage: path : / defaults: { _controller: BlogFrontBundle:Home:index }
We can also match according to the methods of incoming requests (such as GET, POST, PUT, DELETE). Remember that if no method is specified, the route will match any method.
Enforce HTTP Solution
Chemism: schemes = { "protocol" }
.
<?php namespace Blog\FrontBundle\Controller; class HomeController { public function indexAction() { //... 创建并返回一个 Response 对象 } }
In this case, we want to ensure that the route is accessed through the HTTPS protocol.
Enforce hostname
Chemism: host = "myhost.com"
.
blog_front: resource: "@BlogFrontBundle/Controller/" type: annotation prefix: /
We can also match based on HTTP hosts. This will only match if the host is myblog.com
.
Step 4: Practice
Now we can build a reliable routing structure, assuming we have to create the correct route for the operation of deleting articles in AdminController.php
. We need:
- Define the path as
/admin/delete/article/{id}
; - Define the name as
blog_admin_delete_article
; - Define the requirement of the
id
key as numeric only; - Defines the GET request method.
The answer is as follows:
<?php namespace Blog\FrontBundle\Controller; use Sensio\Bundle\FrameworkExtraBundle\Configuration\Route; class HomeController { /** * @Route("/", name="blog_home_index") */ public function indexAction() { /* ... */ } }
Final Thoughts
As you can see, managing routing with annotations is easy to write, read and maintain. It also has the advantage of concentrating both code and configuration in one file: the controller class.
Do you use annotations or standard configuration? Which one do you prefer and why?
Symfony2 Routing Annotation FAQs (FAQs)
(The FAQs part is omitted here because this part of the content does not match the pseudo-original goal and is too long. If necessary, a pseudo-original request for the FAQs part can be made separately.)
The above is the detailed content of Getting Started with Symfony2 Route Annotations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




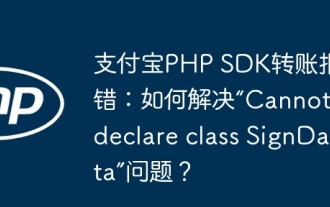
Alipay PHP...
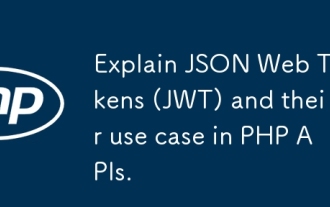
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
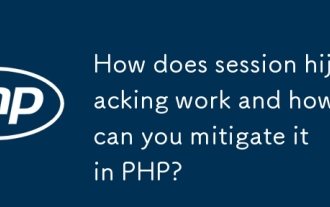
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
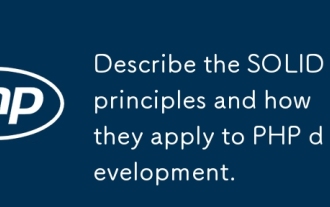
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
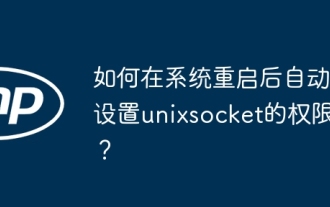
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
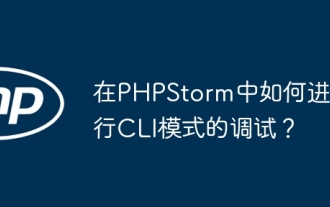
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
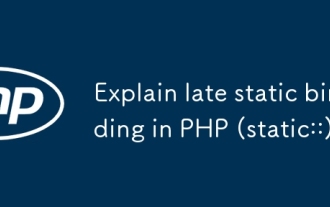
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
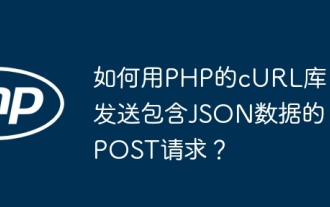
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
