Mock your Test Dependencies with Mockery
While not everyone is doing this, testing your application is one of the most basic parts of being a developer. Unit testing is the most common test. With unit testing, you can check if a class is running exactly as you expected. Sometimes, you are using third-party services in your application, and it's hard to set up everything for unit testing. This is when the simulation comes into play.
Key Points
- Mocking is the process of creating a substitute for real objects in a unit test, which is especially useful when testing applications that rely heavily on dependency injection.
- Mockery is a library created by Pádraic Brady that can be used to mock objects in unit tests, providing an alternative to PHPUnit's default mocking capabilities.
- Mockery allows developers to define expectations for the number of method calls, parameters to be received, and values to be returned, making it a powerful tool for isolating dependencies in unit tests.
- While PHPUnit can already mock objects, Mockery provides greater flexibility and convenience for developers who want to ensure that their unit tests are not affected by other classes.
What is simulation?
Mocking an object is nothing more than creating a substitute object that replaces the real object in unit tests. If your application is heavily dependent on dependency injection, mocking is a viable way.
There may be several reasons for mocking objects:
- It is best to isolate the class when performing unit tests. You don't want another class or service to interfere with your unit tests.
- The object does not exist yet. You can create the test first and then build the final object.
- Mock objects are usually faster than preparing the entire database for testing.
You may be using PHPUnit when running unit tests. PHPUnit comes with some default simulation features as shown in the documentation. You can read more about simulations and the simulation capabilities of PHPUnit in this article written by Jeune Asuncion.
In this article, we will dive into the library Mockery created by Pádraic Brady. We will create a temperature class that will inject weather services that do not currently exist.
Settings
Let's start by setting up the project. We start with the composer.json file that contains the following content. This will ensure we have mockery and PHPUnit.
<code>{ "name": "sitepoint/weather", "license": "MIT", "type": "project", "require": { "php": ">=5.3.3" }, "autoload": { "psr-0": { "": "src/" } }, "require-dev": { "phpunit/phpunit": "4.1.*", "mockery/mockery": "0.9.*" } }</code>
We also create a PHPUnit configuration file called phpunit.xml
<phpunit> <testsuite name="SitePoint Weather"> <directory>./tests</directory> </testsuite> <listeners> <listener class="\Mockery\Adapter\Phpunit\TestListener" file="vendor/mockery/mockery/library/Mockery/Adapter/Phpunit/TestListener.php"/> </listeners> </phpunit>
It is important to define this listener. If there is no listener, if the methods once()
, twice()
and times()
are used incorrectly, no error will be raised. This will be described in detail later.
I also created 2 directories. The src directory is used to hold my classes, and the tests directory is used to store our tests. In the src directory, I created the path SitePointWeather.
We first create the WeatherServiceInterface. Weather services that do not exist will implement this interface. In this case we only provide one method which will give us the temperature of Celsius.
<code>{ "name": "sitepoint/weather", "license": "MIT", "type": "project", "require": { "php": ">=5.3.3" }, "autoload": { "psr-0": { "": "src/" } }, "require-dev": { "phpunit/phpunit": "4.1.*", "mockery/mockery": "0.9.*" } }</code>
Therefore, we have a service that provides us with Celsius temperature. I want to get Fahrenheit. To do this, I created a new class called TemperatureService. This service will inject weather services. In addition to this, we also define a method that converts Celsius to Fahrenheit.
<phpunit> <testsuite name="SitePoint Weather"> <directory>./tests</directory> </testsuite> <listeners> <listener class="\Mockery\Adapter\Phpunit\TestListener" file="vendor/mockery/mockery/library/Mockery/Adapter/Phpunit/TestListener.php"/> </listeners> </phpunit>
Create unit tests
We are ready to set up unit tests. We create a TemperatureServiceTest class in the tests directory. In this class we create the method testGetTempFahrenheit()
which will test our Fahrenheit method.
The first step to do in this method is to create a new TemperatureService object. Just as we do this, our constructor will request an object that implements the WeatherServiceInterface. Since we don't have such an object yet (we don't want it either), we will use Mockery to create a mock object for us. Let's see what the method looks like after completion.
namespace SitePoint\Weather; interface WeatherServiceInterface { /** * 返回摄氏温度 * * @return float */ public function getTempCelsius(); }
We first create the mock object. We tell Mockery which object (or interface) we want to mock. The second step is to describe the method that will be called on this mock object. In the shouldReceive()
method, we define the name of the method to be called.
We define the number of times this method will be called. We can use once()
, twice()
and times(X)
. In this case, we expect it to be called only once. If not called or excessive calls are called, the unit test will fail.
Finally, we define the value to be returned in the andReturn()
method. In this case, we return 25. Mockery also has return methods such as andReturnNull()
, andReturnSelf()
and andReturnUndefined()
. If that's what you expect, Mockery can throw exceptions as well.
We now have mock objects that can create our TemperatureService object and test it as usual. 25 degrees Celsius is 77 degrees Fahrenheit, so we check if we receive 77 from our getTempFahrenheit()
method.
If you run vendor/bin/phpunit tests/
in your root directory, you will get a green light from PHPUnit, indicating that everything is perfect.
Advanced Usage
The above example is quite simple. No parameters, just a simple call. Let's make things more complicated.
Suppose our weather service also has a way to get the temperature at the exact hours. We add the following method to our current WeatherServiceInterface.
namespace SitePoint\Weather; class TemperatureService { /** * @var WeatherServiceInterace $weatherService 保存天气服务 */ private $weatherService; /** * 构造函数。 * * @param WeatherServiceInterface $weatherService */ public function __construct(WeatherServiceInterface $weatherService) { $this->weatherService = $weatherService; } /** * 获取当前华氏温度 * * @return float */ public function getTempFahrenheit() { return ($this->weatherService->getTempCelsius() * 1.8000) + 32; } }
We want to know what the average temperature is between 0:00 and 6:00 pm. To do this, we create a new method in TemperatureService to calculate the average temperature. To do this, we retrieve 7 temperatures from WeatherService and calculate the average.
<code>{ "name": "sitepoint/weather", "license": "MIT", "type": "project", "require": { "php": ">=5.3.3" }, "autoload": { "psr-0": { "": "src/" } }, "require-dev": { "phpunit/phpunit": "4.1.*", "mockery/mockery": "0.9.*" } }</code>
Let's take a look at our testing method.
<phpunit> <testsuite name="SitePoint Weather"> <directory>./tests</directory> </testsuite> <listeners> <listener class="\Mockery\Adapter\Phpunit\TestListener" file="vendor/mockery/mockery/library/Mockery/Adapter/Phpunit/TestListener.php"/> </listeners> </phpunit>
We simulate the interface again and define the method to be called. Next, we define the number of times this method will be called. We used once()
in the previous example, and now we use times(7)
to indicate that we expect this method to be called 7 times. If the method is not called exactly 7 times, the test will fail. If you do not define the listener in the phpunit.xml configuration file, you will not receive notifications about this.
Next, we define the with()
method. In the with
method, you can define the parameters you expect. In this case, we expect 7 different hours.
Finally, we have the andReturn()
method. In this case, we indicate 7 return values. If you define a fewer return values, the last available return value will be repeated each time.
Of course, Mockery can do more. For the complete guide and documentation, I recommend you check out the Github page.
If you are interested in the code of the project above, you can check out this Github page.
Conclusion
Using PHPUnit, you can already mock objects. However, you can also use Mockery as explained in the example above. If you are unit testing your class and you don't want any other class to affect your tests, mockery can help you easily. If you really want to do functional testing, it's better to see if you can integrate real testing. Are you currently using PHPUnit simulation and considering switching to Mockery? Do you want to see more and bigger Mockery examples in subsequent posts? Please let me know in the comments below.
FAQs about Mockery and Test Dependencies (FAQ)
What is Mockery and why is it important in PHP testing?
Mockery is a powerful and flexible PHP mock object framework for unit testing. It is designed as a direct alternative to PHPUnit's mock object functionality. Mockery allows developers to isolate the tested code and create test stand-ins that simulate the behavior of complex objects. This is crucial in unit testing because it ensures that the code being tested does not depend on any external factors or state.
How to install and set up Mockery in my PHP project?
To install Mockery, you need to have Composer, a PHP dependency manager. You can install Mockery by running the command composer require --dev mockery/mockery
. After installation, you can set up Mockery in the test file by calling Mockery::close()
in the test teardown method to clean up the mock objects.
How to create mock objects using Mockery?
Creating mock objects in Mockery is simple. You can use the mock()
method to create a mock object. For example, $mock = Mockery::mock('MyClass');
will create a mock object for MyClass.
How to define expectations in Mockery?
In Mockery, you define expectations by linking methods to mock objects. For example, $mock->shouldReceive('myMethod')->once()->andReturn('mocked value');
This code tells Mockery that expects "myMethod" to be called once and should return "mocked value".
What is the difference between simulation and stub in Mockery?
In Mockery, a mock is the object on which we can set the desired one, while a stub is a mock object that has a pre-programmed response. When response is the only important thing, stubs are usually used, and when testing the interaction itself, mocks are used.
How to use Mockery to test private methods?
It is not recommended to directly test private methods because it violates the encapsulation principle. However, if you want, you can use the shouldAllowMockingProtectedMethods()
method in Mockery to allow the mocked protected and private methods.
How to handle constructor parameters in Mockery?
If the class you want to simulate has constructors with arguments, you can pass them as an array to the mock()
method. For example, $mock = Mockery::mock('MyClass', [$arg1, $arg2]);
will pass $arg1 and $arg2 to the constructor of MyClass.
How to use Mockery to simulate static methods?
Mockery provides a method to simulate static methods using the alias:
prefix. For example, $mock = Mockery::mock('alias:MyClass');
will create a mock object that can be used to set the desired setting of the static method of MyClass.
How to verify that all expectations are met in Mockery?
You can use the Mockery::close()
method in the test disassembly method to verify that all expectations have been met. If any expectations are not met, Mockery will throw an exception.
How to handle exceptions in Mockery?
You can use the andThrow()
method to set up the mock object to throw an exception. For example, $mock->shouldReceive('myMethod')->andThrow(new Exception);
will throw an exception when "myMethod" is called.
The above is the detailed content of Mock your Test Dependencies with Mockery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


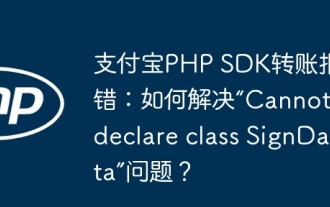
Alipay PHP...
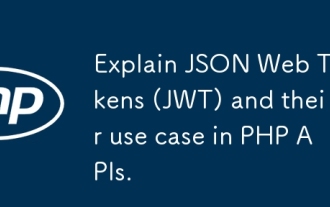
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
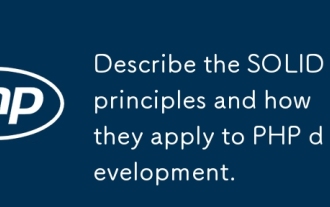
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
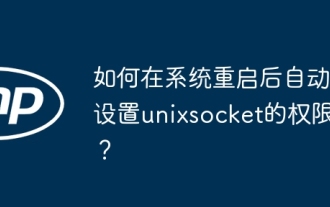
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
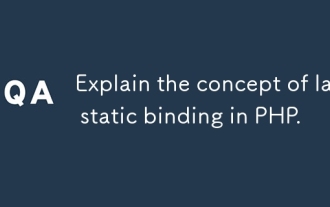
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
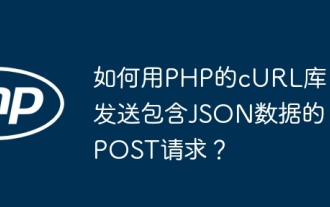
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
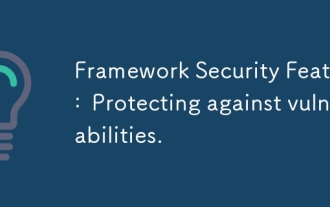
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
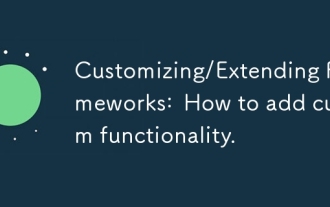
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
