Revealing the Inner Workings of JavaScript's 'this' Keyword
Mastering JavaScript often means understanding its nuances, and the this
keyword is a prime example. While JavaScript is relatively easy to learn, the behavior of this
can trip up even experienced programmers. This article clarifies the complexities of this
in JavaScript.
Key Concepts:
-
Contextual Binding:
this
in JavaScript isn't statically defined; its value depends entirely on how the function is called (the execution context), not where it's declared. It points to the "owner" of the currently executing function. -
Variable Behavior: The behavior of
this
varies across different contexts: global scope, simple function calls, object methods, and constructor functions. -
Context Control: JavaScript offers methods like
call()
,apply()
, andbind()
to explicitly manage the value ofthis
, providing fine-grained control over its behavior. -
Common Pitfall: Misunderstanding
this
is a frequent source of errors for both beginners and experts.
Understanding this
:
this
is a special keyword automatically present within every function. It acts as a reference, but its target is dynamic and determined at runtime based on the function's invocation context. To fully understand this
, we need to consider:
-
Creation: When a JavaScript function executes, a new execution context is created. This context includes information about the function's invocation, including the value of
this
, which is bound to the object that called the function (if applicable). -
Reference: The value of
this
is determined by the call site—where the function is invoked, not where it's defined. The context changes with each new function call.
Example:
var car = { brand: "Nissan", getBrand: function(){ console.log(this.brand); } }; car.getBrand(); // Output: Nissan
Here, this.brand
inside getBrand()
refers to car.brand
because getBrand()
is called as a method of the car
object.
Invocation Contexts:
Let's explore how this
behaves in various contexts:
-
Global Scope: In the global scope (outside any function),
this
usually refers to the global object (e.g.,window
in browsers). -
Simple Function Call: A direct function call without an object context. In non-strict mode,
this
defaults to the global object. In strict mode ("use strict";
),this
isundefined
. -
Object Method: When a function is called as a method of an object,
this
is bound to that object. -
Constructor Function: When a function is invoked with
new
, it becomes a constructor.this
is bound to the newly created object instance.
Manipulating this
:
The call()
, apply()
, and bind()
methods provide ways to explicitly set the value of this
:
-
call()
: Invokes a function with a specifiedthis
value and arguments passed individually. -
apply()
: Similar tocall()
, but accepts arguments as an array. -
bind()
: Creates a new function with a permanently boundthis
value.
Summary:
The this
keyword in JavaScript is powerful but can be confusing. Understanding its contextual nature and utilizing methods like call()
, apply()
, and bind()
are crucial for writing robust and error-free JavaScript code. Further exploration of edge cases and common pitfalls will be covered in a future article.
Frequently Asked Questions (FAQs):
The FAQs section from the original text is already a comprehensive summary of common this
-related questions and answers, so it's best to retain it as is.
The above is the detailed content of Revealing the Inner Workings of JavaScript's 'this' Keyword. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
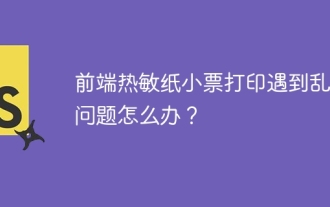
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
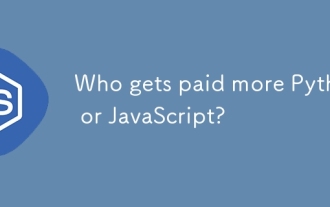
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
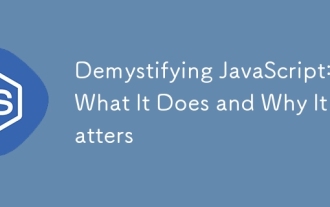
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
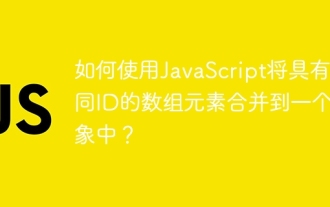
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
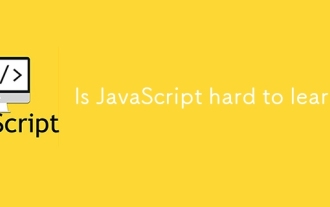
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
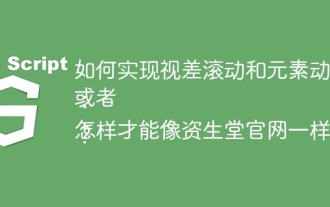
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
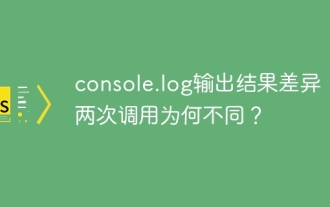
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
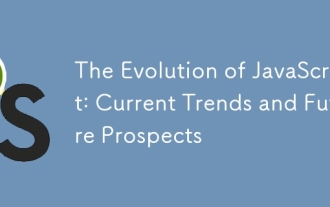
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
