10 Tips to Make Your Node.js Web App Faster
Node.js, inherently fast due to its asynchronous and event-driven architecture, still requires optimization for peak modern web performance. This article outlines ten crucial strategies to significantly boost your Node.js application's speed. Let's explore each technique.
Key Takeaways:
- Leverage Node.js's asynchronous capabilities for parallel function execution, minimizing middleware and maximizing speed.
- Consistently utilize asynchronous APIs to prevent main thread blockage and performance degradation.
- Implement caching for static data and employ gzip compression to reduce response sizes.
- Optimize JavaScript by minifying and concatenating files, and consider using Nginx as a reverse proxy to offload server strain.
-
Parallel Processing: When fetching data requiring multiple internal API calls (e.g., user profile, activity, subscriptions, notifications for a dashboard), avoid sequential execution. Node.js excels at parallel asynchronous operations. Use a library like
async.js
to concurrently execute these independent functions, dramatically reducing processing time. Example usingasync.js
:
function runInParallel() { async.parallel([ getUserProfile, getRecentActivity, getSubscriptions, getNotifications ], function(err, results) { // Callback executed upon completion of all functions }); }
-
Embrace Asynchronicity: Node.js's single-threaded nature makes synchronous code a performance bottleneck. Always favor asynchronous file system operations (e.g.,
fs.readFile
overfs.readFileSync
) and carefully vet third-party libraries for potential synchronous calls. -
Caching Strategies: Cache frequently accessed, static data to minimize database hits. Redis, for example, provides an excellent key-value store for this purpose. Cache data and implement a mechanism to refresh the cache periodically.
-
Gzip Compression: Enable gzip compression to significantly reduce the size of responses sent to the client. Express.js offers middleware for easy implementation.
-
Client-Side Rendering: For single-page applications (SPAs), consider client-side rendering using frameworks like Angular, React, or Vue.js. Serving JSON data instead of full HTML pages reduces bandwidth consumption and improves speed.
-
Session Data Management: Avoid storing excessive data in sessions (often stored in memory). Limit session data to essential information (e.g., user ID) and retrieve full objects as needed. Consider alternative session storage like MongoDB or Redis for scalability.
-
Database Query Optimization: Optimize database queries to retrieve only necessary fields. Avoid fetching unnecessary data, especially in large datasets.
-
Leverage V8 Functions: Utilize built-in V8 JavaScript functions (e.g.,
map
,reduce
,forEach
) for efficient collection manipulation. -
Nginx Reverse Proxy: Use Nginx as a reverse proxy to serve static assets, offloading this task from your Node.js server and improving its responsiveness. Nginx can also handle gzip compression.
-
Minify and Concatenate JavaScript/CSS: Minify and concatenate JavaScript and CSS files to reduce the number of HTTP requests and improve loading times. Build tools like Webpack or Parcel can automate this process.
Conclusion: Implementing these optimizations can significantly enhance your Node.js application's performance. Remember to profile your application to identify specific bottlenecks and tailor your optimization strategy accordingly.
Frequently Asked Questions (FAQ): (Similar to the original, but rephrased for conciseness and clarity)
- How to improve Node.js app performance? Use the Cluster module for multi-process handling, gzip compression, and a reverse proxy like Nginx.
- Using the Cluster module? The Cluster module creates child processes sharing a port, handling more requests concurrently.
- What is gzip compression? Gzip reduces response body size, speeding up data transfer.
- How does Nginx improve performance? Nginx serves static files, freeing Node.js to handle other tasks.
- Other performance strategies? Use CDNs, data caching, and process managers like PM2.
- Using a CDN? CDNs distribute content geographically, reducing latency.
- How does caching help? Caching reduces database queries by storing frequently accessed data.
- What is PM2? PM2 keeps your app running consistently, even after crashes.
- Monitoring Node.js performance? Use tools like the Node.js Performance Hooks API and the Node.js Inspector.
- Common performance issues? Event loop blocking, excessive database queries, and memory leaks. Avoid these by writing non-blocking code, caching, and using debugging tools.
The above is the detailed content of 10 Tips to Make Your Node.js Web App Faster. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


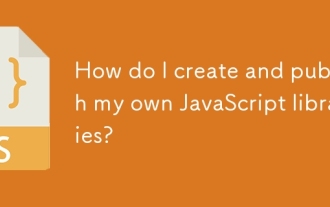
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
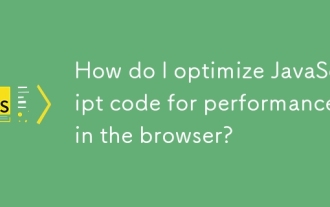
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
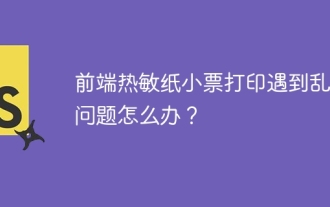
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
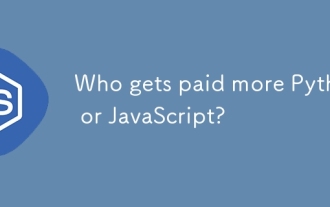
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
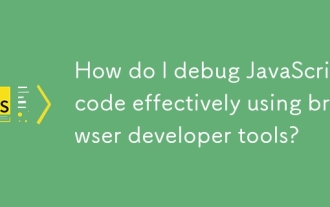
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
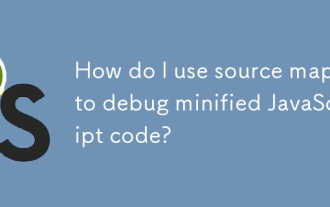
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
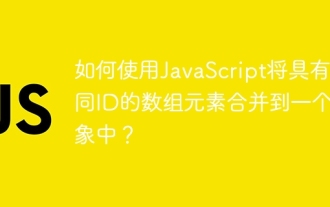
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
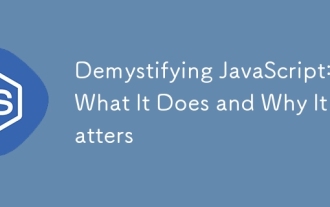
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
