Building a Library with RequireJS
Key Points
- RequireJS is an AMD module loader for browsers that asynchronously load scripts and CSS files, manage dependencies and build code structures. It also includes an optimization tool for production environments.
- When using RequireJS, the code needs to be wrapped in the module definition. Modules can be referenced in other modules, and all dependencies will be loaded before the module itself is loaded.
- RequireJS optimizer r.js can be configured to build all modules into a single file. This configuration can also make the module an independent global library, both as an AMD module and as a global export in the browser.
- RequireJS can be used to build libraries and applications that use them. This process involves defining and using AMD modules, configuring r.js optimizer, and configuring RequireJS in the browser to generate well-structured and organized code.
RequireJS is an AMD module loader for browsers that can load scripts and CSS files asynchronously. You no longer need to process the order of script files in a single file (such as index.html). Instead, you just wrap your code in a module definition, RequireJS will be responsible for dependency management, making your code structure clearer and more organized. It also has an optimization tool that compresses and connects files for production environments.
The official website provides detailed documentation on its API and there are many sample code bases to help you. However, it has a lot of configurations, and using RequireJS will be tricky at the beginning.
In this article, we will learn how to use RequireJS by building a library with AMD modules, optimizing it, and exporting it as a standalone module using the RequireJS optimizer. Later, we will build the application using RequireJS and use our library.
This tutorial assumes that you have a certain understanding of RequireJS. If you are looking for a get-start guide, check out: Understand RequireJS for efficient JavaScript module loading.
Installation RequireJS
RequireJS can be installed through bower:
bower install requirejs --save
Or you can get the file from github.
There is also a Grunt-based Yeoman generator for RequireJS projects.
Define AMD module
We wrap the code in define()
which will make it an AMD module.
File: mylib.js
define(['jquery'], function($) { // $现在是jquery。 return 'mylib'; });
That's it. Note that define()
accepts an optional first parameter, the dependency array, in this case ['jquery']
. It is the dependency list for this module. All modules in the array will be loaded before this module. When executing this module, the parameters are the corresponding module in the dependency array.
So in this case, jQuery will be loaded first, then passed it into the function as parameter $, and then we can use it safely in the module. Finally, our module returns a string. The return value is what is passed to the function parameter when this module is required.
Cite other modules
Let's see how it works by defining the second module and referring to our first module mylib.js.
File: main.js
bower install requirejs --save
You can refer to any number of dependencies in the dependency array, and all modules will be provided by function parameters in the same order. In this second module, we reference the jquery and mylib modules and simply return an object exposes certain variables. Users of this library will use this object as your library.
Configure RequireJS optimizer: r.js
You may be wondering, how does RequireJS know which file to load by just looking at the strings in the dependency array? In our example, we provide jquery and mylib as strings, RequireJS knows where these modules are. mylib is very simple, it is mylib.js, omitting .js.
Where is jquery? This is what RequireJS configuration is for. You can provide a wide range of configurations through RequireJS configuration. There are two ways to provide this configuration, since we are using the RequireJS optimizer and I will show you the r.js method. r.js is the RequireJS optimizer.
We will provide r.js with a configuration that optimizes all modules into a single file. The configuration we provide will enable r.js to build modules into independent global libraries, both as AMD modules and as global exports in the browser.
r.js can be run via the command line or as a Node module. There is also a Grunt task for running the optimizer grunt-requirejs.
With that being said, let's see what our configuration looks like:
File: tools/build.js
define(['jquery'], function($) { // $现在是jquery。 return 'mylib'; });
Configuration files are actually the core of RequireJS. Once you understand how these parameters work, you can use RequireJS like a professional.
You can perform different actions and adjust project builds using configuration files. To learn more about configuration and RequireJS, we recommend that you refer to the documentation and wiki. There is also a sample configuration file that demonstrates how to use the build system, so be sure to refer to it as well.
Finally, we actually ran the optimizer. As I said before, you can run it through the command line or through the Node and the Grunt task. See the r.js readme file for how to run the optimizer in different environments.
define(['jquery', 'mylib'], function($, mylib) { // $照常是jquery // mylib是字符串`mylib`,因为这是第一个模块的返回值 // return { version: '0.0.1, jQuery版本:' + $.fn.jquery, mylibString: mylib } });
This will generate the build file in dist/mylib.js
build.js
Next, let's see what these parameters actually mean.
baseUrl – The root path for all modules to look for.
paths – Path map relative to the module name of baseUrl.
In our example, "mylib" maps to "../main", which is relative to baseUrl, so when we reference "mylib", it loads the file "../lib/../mylib/main .js”. Note that we append baseUrl, then paths settings, then module name followed by .js suffix. There you can specify how the module is mapped to files such as jquery and mylib.
include – The module we want to include in the optimization process. The required dependencies for the included module are implicitly included. In our example, the main module depends on mylib and jquery, which will be included as well, so there is no need to include it explicitly. We also include almond, which will be mentioned later.
exclude – Module we want to exclude from the optimization process. In our case, we ruled out jquery. The user who builds the library will provide a jQuery library. We'll see this later.
out – The name of the optimized output file.
wrap – wraps the build package in the start and end text specified by wrap. The optimized output file is as follows: wrap.start The module wrapped.end contained in it. wrap.start and wrap.end are the file names whose contents are contained in the output.
almond
The built library does not contain the require.js file, but uses almond. almond is a small AMD API implementation that will replace require.js.
Pack our library
In the r.js configuration, we wrap our library using wrap.start and wrap.end files. We also include almonds in the library, which will make our library independent, so they can be used via browser global variables or as an AMD module via requirejs.
File: wrap.start
bower install requirejs --save
The modules we include main, mylib and almond are located in the middle of wrap.start and wrap.end.
File: wrap.end
define(['jquery'], function($) { // $现在是jquery。 return 'mylib'; });
If the user uses the AMD loader, the built file will request "jquery" as an AMD dependency. If the consumer only uses browser global variables, the library will get the $global variable and use it for the jQuery dependency.
Using the library with RequireJS
Our library is done, now let's actually use it by building a requirejs application.
File: app.js
define(['jquery', 'mylib'], function($, mylib) { // $照常是jquery // mylib是字符串`mylib`,因为这是第一个模块的返回值 // return { version: '0.0.1, jQuery版本:' + $.fn.jquery, mylibString: mylib } });
Nothing special here, it's just another module that references jQuery and mylib. When a module is defined with define
, it is not executed immediately, that is, its callback function (passed after an array of dependencies) is not executed immediately. This means that our application will not be launched simply by defining this module. Now let's see how to configure RequireJS and actually execute this module, our application.
Configure RequireJS for browser
We will configure RequireJS in a file and execute our app module. However, there are different ways to do this.
File: common.js
{ "baseUrl": "../lib", "paths": { "mylib": "../main" }, "include": ["../tools/almond", "main"], "exclude": ["jquery"], "out": "../dist/mylib.js", "wrap": { "startFile": "wrap.start", "endFile": "wrap.end" } }
baseUrl and paths configurations are the same as before. The additional configuration value here is:
shim: Configure dependencies and exports of traditional "browser global" scripts that do not use define()
to declare dependencies and set module values. For example, Backbone is not an AMD module, but it is a browser global variable that exports Backbone to the global namespace we have specified in exports. In our example, the module also relies on jQuery and Underscore, so we specify it using deps. The script in the deps array is loaded before loading Backbone, and after loading, the exports value will be used as the module value.
Please note that you can also use r.js in this application project, which will require a separate configuration. But don't be confused about it. I won't go into details on how to do it, but this is similar to what we did with the library. See the sample build configuration for more information.
require and define
We will load the module using require and execute it immediately. Sometimes define and require may confuse which one to use. define defines a module, but does not execute it, requires define a module and executes it - that is, it loads and executes a dependent module before it executes itself. Typically, you will have a require as the main entry module, which will depend on other modules defined via define.
Loading script
Usually, you will include all script files in index.html. Now that we use RequireJS, we just need to include RequireJS and specify our data-main, which is the entry point for our application. There are many ways to set configuration options or detach the main module used in index.html. You can find more information here.
bower install requirejs --save
Conclusion
In this article, we built a library and an application that uses the library using RequireJS. We learned how to configure the r.js optimizer and how to configure RequireJS in the browser. Finally, we learned how to define and use AMD modules using RequireJS. This makes our code well-structured and organized.
In the first half of this tutorial (configuration optimizer), I used this example-libglobal repository, the second half is not complicated, so you should be able to do it yourself now.
The official RequireJS website is the final documentation, but be sure to check out the sample repository on github and the sample projects in that repository that demonstrate the use of the RequireJS application.
FAQs (FAQs) about building libraries with RequireJS
What is the main purpose of RequireJS in JavaScript development?
RequireJS is a JavaScript file and module loader. It is optimized for browser usage, but can also be used in other JavaScript environments. The main purpose of RequireJS is to encourage the use of modular programming in JavaScript. It helps developers manage dependencies between JavaScript files and modularize their code. This leads to better code organization, maintainability, and reusability. It also improves the speed and quality of the code.
How does RequireJS handle JavaScript file dependencies?
RequireJS uses the Asynchronous Module Definition (AMD) API to handle JavaScript modules. These modules can be loaded asynchronously, meaning they do not block other scripts from running when loading. When you define a module using RequireJS, you specify its dependencies. RequireJS then ensures that these dependencies are loaded before the module itself.
How to define modules using RequireJS?
To define a module in RequireJS, you can use the define()
function. This function takes two parameters: a dependency array and a factory function. A dependency is the path to the file on which the module depends. Factory functions are where you write module code. This function is called once all dependencies are loaded.
How to use modules defined with RequireJS in your code?
To use a module defined with RequireJS, you can use the require()
function. This function accepts two parameters: a dependency array and a callback function. Dependencies are the path to the module you want to use. The callback function is where you use the module. This function is called once all modules are loaded.
Can I use RequireJS with other JavaScript libraries such as jQuery?
Yes, you can use RequireJS with other JavaScript libraries such as jQuery. RequireJS has a built-in feature for loading traditional non-modular scripts that do not use define()
to declare dependencies and set module values, called "shim". With shim, you can specify dependencies and exports for scripts that do not use define()
to declare dependencies and set module values.
How to optimize my code with RequireJS?
RequireJS comes with an optimization tool called r.js. This tool combines and compresses your JavaScript files and their dependencies into a single file. This reduces the number of HTTP requests and file size, which can greatly improve the loading time of the web page.
What is the difference between define() and require() in RequireJS?
Thedefine()
function is used to define a module, while the require()
function is used to load the module. Both functions accept an array of dependencies and a function as parameters. However, the function passed to define()
is used to create module values, while the function passed to require()
is used to run the code after the module is loaded.
Can I use RequireJS in Node.js?
Yes, you can use RequireJS in Node.js. However, Node.js has its own module system, so you probably don't need RequireJS. If you want to use the same code in both your browser and Node.js, or if you prefer the AMD API, RequireJS may be a good choice.
How to deal with errors in RequireJS?
RequireJS provides a onError
callback to handle errors. This callback is called when an error occurs while loading the module. You can use this callback to log or recover from the error.
Can I load CSS files using RequireJS?
Yes, you can use the require-css plugin to load CSS files using RequireJS. This plugin allows you to load and wait for CSS files just like you would with JavaScript modules.
The above is the detailed content of Building a Library with RequireJS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










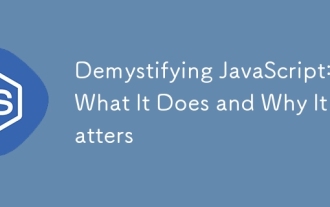
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
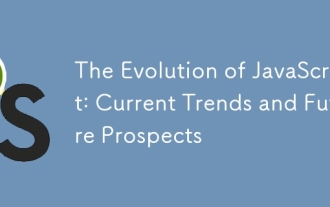
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
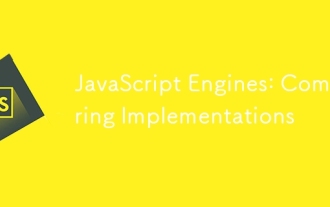
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
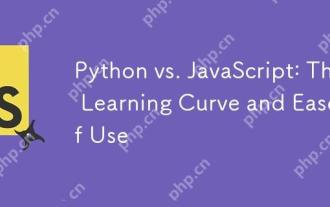
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
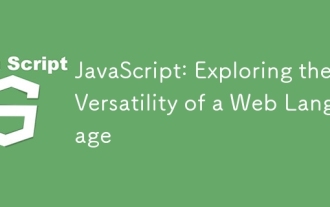
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
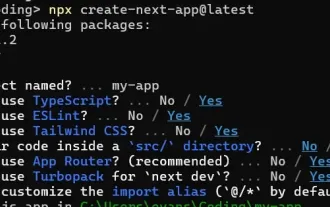
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
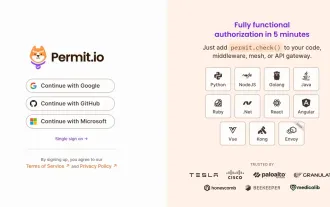
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
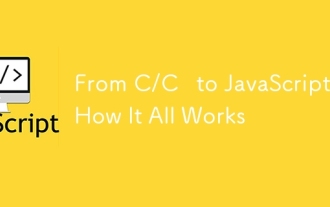
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
