Getting Started with Assetic
Or, you could create a file called stylesheets.php and embed it inline:
<style> <?php include('/assets/stylesheets.php'); </style>
Alternatively you could generate .css and .js files and just reference those as normal. You can use the AssetWriter for this:
use Assetic\AssetWriter; $scripts.js = new AssetCollection(array( new GlobAsset('/assets/js/libs/*'), new FileAsset('/assets/js/app.js'), ), array( new JSMinFilter(), )); // Set target path, relative to the path passed to the // AssetWriter constructor as an argument shortly $scripts->setTargetPath('scripts.js'); $am->set('scripts.js', $scripts.js); // see above for instantiation of $styles $styles->setTargetPath('stylesheets.css'); $am->set('styles', $styles); $writer = new AssetWriter('/assets/build'); $writer->writeManagerAssets($am);
You could create a command-line script to do this as part of your workflow, or use a tool such as Guard to “watch” the filesystem and re-run it whenever one of the relevant files has changed.
Caching
Assetic ships with a simple file-based caching mechanism to ensure that filters aren’t run unnecessarily. Here’s an example of caching the output of the YUI Compressor:
use Assetic\Asset\AssetCache; use Assetic\Asset\FileAsset; use Assetic\Cache\FilesystemCache; use Assetic\Filter\Yui; $yui = new Yui\JsCompressorFilter('/path/to/yuicompressor.jar'); $js = new AssetCache( new FileAsset('/path/to/some.js', array($yui)), new FilesystemCache('/path/to/cache') ); // the YUI compressor will only run on the first call $js->dump(); $js->dump(); $js->dump();
Summary
In this article I’ve introduced Assetic – a PHP package for managing assets. I’ve shown how you can use it to manage dependencies, run compilation processes, minify / pack / compress / optimise assets, and concatenate files to minimise the number of HTTP requests. Be sure to check out the documentation for details of all the available filters; or, you could even look at implementing FilterInterface / extending BaseFilter with a view to defining your own. For packages which complement it, refer to the suggested packages either when you first install it, or by inspecting the suggests section of its composer.json file.
Frequently Asked Questions (FAQs) about Assetic
What is Assetic and how does it work?
Assetic is a powerful asset management framework for PHP. It provides a systematic and efficient way to manage web assets such as CSS, JavaScript, and image files. Assetic works by allowing you to filter, combine, and compress these assets, which can significantly improve the performance of your website. It also supports a variety of filters, including CSS minification, JS minification, and LESS compilation, among others.
How can I install Assetic?
Assetic can be installed using Composer, a tool for dependency management in PHP. You can install Composer by following the instructions on its official website. Once Composer is installed, you can install Assetic by running the command “composer require kriswallsmith/assetic”.
How can I use Assetic with Symfony?
Assetic is often used with Symfony, a PHP web application framework. To use Assetic with Symfony, you need to install the Assetic bundle. Once installed, you can use Assetic to manage your web assets in your Symfony application. You can define asset collections in your Symfony configuration and use the Assetic controller to serve these assets.
What are the benefits of using Assetic?
Assetic offers several benefits. It allows you to manage your web assets in a systematic and efficient way, which can significantly improve the performance of your website. It also supports a variety of filters, which can help you to optimize your assets. Furthermore, Assetic is flexible and can be used with a variety of web application frameworks, including Symfony.
Can I use Assetic without Symfony?
Yes, Assetic can be used without Symfony. While Assetic is often used with Symfony, it is a standalone library and can be used with any PHP application. To use Assetic without Symfony, you need to install it using Composer and then use it to manage your web assets.
How can I use filters in Assetic?
Filters in Assetic allow you to transform your assets in various ways. For example, you can use a CSS minification filter to reduce the size of your CSS files, or a LESS compilation filter to compile your LESS files into CSS. To use a filter in Assetic, you need to define it in your configuration and then apply it to your assets.
What is asset collection in Assetic?
An asset collection in Assetic is a group of assets that are managed together. You can define an asset collection in your configuration and then use the Assetic controller to serve these assets. Asset collections can be used to combine and compress multiple assets into a single file, which can significantly improve the performance of your website.
How can I debug assets in Assetic?
Assetic provides a debug mode that can be used to troubleshoot issues with your assets. When debug mode is enabled, Assetic will serve each asset individually, rather than combining them into a single file. This can make it easier to identify and fix issues with your assets.
Can I use Assetic with other web application frameworks?
Yes, Assetic is a standalone library and can be used with any PHP application. While it is often used with Symfony, it can also be used with other web application frameworks. To use Assetic with another framework, you need to install it using Composer and then use it to manage your web assets.
How can I optimize my assets using Assetic?
Assetic provides several ways to optimize your assets. You can use filters to transform your assets in various ways, such as minifying CSS and JS files or compiling LESS files into CSS. You can also use asset collections to combine and compress multiple assets into a single file. These features can significantly improve the performance of your website.
The above is the detailed content of Getting Started with Assetic. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


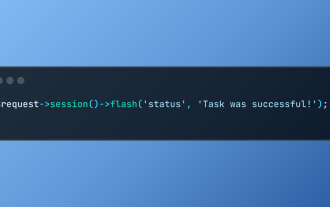
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
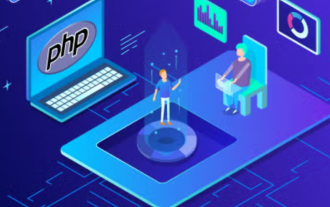
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
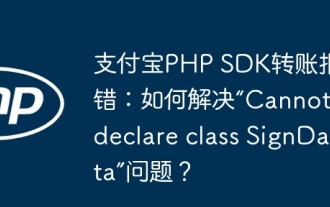
Alipay PHP...
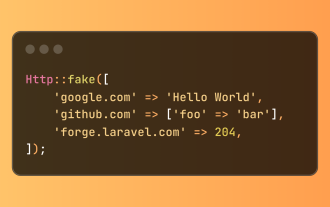
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
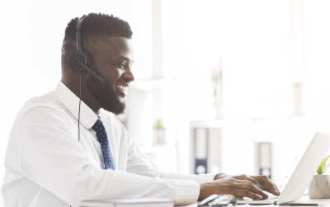
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
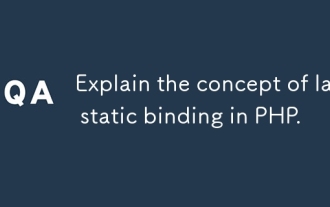
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
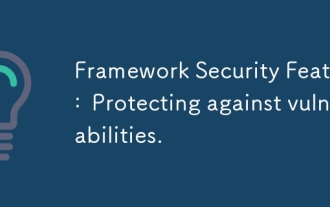
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
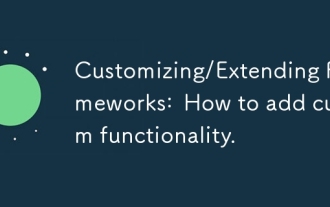
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
