8 Clever Tricks with CSS Functions
The power of CSS is far beyond many web developers' imagination. Over time, the stylesheet language has become more and more powerful, bringing functions to the browser that originally required JavaScript to achieve. This article will introduce eight clever CSS function tips that require no JavaScript at all.
Key points
- CSS functions can be used to create various effects and functions such as tooltips, thumbnail titles, counters, and frosted glass effects without JavaScript.
-
calc()
Functions can be used to create smarter grid systems and alignment of fixed position elements, providing greater flexibility and precision in design.
The - CSS function also allows creative animations using the
cubic-bezier()
function, as well as the potential to use HTML elements as background images using theelement()
function, although the latter is currently only supported by Firefox.
Pure CSS Tooltips
Many websites still use JavaScript to create tooltips, but in fact it is easier to use CSS. The easiest solution is to use data properties in HTML code to provide tooltip text, such as data-tooltip="…"
. With this tag, you can put the following into CSS to display tooltip text within the attr()
function:
.tooltip::after { content: attr(data-tooltip); }
It's very simple and clear, right? Of course, more code is needed to actually style the tooltips, but don't worry, there is a great pure CSS library specifically for this purpose called Hint.css.
(abuse) custom data attributes and attr() function
We have used attr()
for tooltips, but it has some other use cases. Combining data properties, you can build thumbnail images with titles and descriptions using just one line of HTML code:
<a class="caption" href="https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15b" data-title="秃鹫" data-description="..."> <img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/174011191897662.jpg" class="lazy" alt="8 Clever Tricks with CSS Functions " /> </a>
You can now use the attr()
function to display the title and description:
.caption::after { content: attr(data-title); ... }
This is a working example of a thumbnail with hover display animation title: [CodePen example link] (assuming that CodePen link should be inserted here)
Note: The generated content may have problems with accessibility. This article on accessibility support for CSS generated content provides a useful interpretation of this topic.
You can use CSS counters, which seems like magic. It's not the most well-known feature, and most people may think it doesn't support it very well, but in fact, every browser supports CSS counters: [Can I Use css-counters?] (assuming Can I Use should be inserted here Link)
While you should not use CSS counters for ordered lists (<ol>
), counters are great for pagination or displaying numbers under items in gallery. You can also count the number of selected items, which is very impressive considering that you need very little code (and no JavaScript): [CodePen sample link] (assuming that CodePen link should be inserted here).
CSS counters are also great for dynamically changing numbers in the project list, which can be reordered by drag and drop: [CodePen Example Link] (assuming that CodePen link should be inserted here).
As with the last example, remember that the generated content may have problems with accessibility.
With the release of iOS 7, Apple brings us a “frosted glass” effect—transparent, blurry elements that look like frosted glass windows. Inspired by Apple's implementation, this effect began to appear in many places. Reproducing this effect is a bit tricky before you have a CSS filter, you have to use a blurred image to create a frosted glass effect. Now, almost all major browsers fully support CSS filters, so it's much easier to reproduce this effect. [Can I Use css-filters?](assuming that Can I Use link should be inserted here)
In the future, we can use the
and functions to create similar effects, but these two functions are currently supported only by Safari. backdrop-filter
filter()
Usually, you specify a JPEG or PNG file as the background image, or maybe a gradient. But did you know that using the
function, you can also use as the background image? Currently, the element()
function is only supported by Firefox: [Can I Use css-element-function?] (assuming that the Can I Use link should be inserted here) <div>
element()
The possibilities are almost limitless, here is an example from MDN.
Fluid mesh is a great thing, but there are some serious problems. For example, it is not possible to make the spacing between the top and the bottom the same size as the spacing between the left and right. Also, the marking is very confusing, depending on the grid system you are using. Even flexbox itself is not the final solution, but using the
function (which can be used as a value in CSS), the grid can get better. In this tutorial on this website, George Martsoukos shows some practical examples, such as a gallery grid with perfect spacing. Using CSS preprocessors like Sass, building creative mesh systems can be very simple and easy to maintain. Since browser support is almost perfect, is a convenient feature you should definitely use. [Can I Use calc?](assuming that Can I Use link should be inserted here)calc()
calc()
Another use case for a function is to align elements with fixed positions. For example, if you have a content wrapper on the left and right with fluid spacing, and you want to precisely align the fixed elements inside that wrapper - you will have a hard time determining the "right" or "left" attribute selection Which value? With , you can combine relative and absolute values to align elements perfectly:
calc()
calc()
.tooltip::after {
content: attr(data-tooltip);
}
To make the UI of a website or application more attractive, you can use animations, but the standard easing options are very limited. For example, "linear" or "ease-in-out". Something like bouncing animation is not possible even with standard options. Using the
function, you can animate elements exactly as you wish.
cubic-bezier()
There are two ways to use
generator. cubic-bezier()
cubic-bezier
Honestly, I will choose the latter.
Skillful use of CSS functions not only solves known problems (such as building a smarter grid system), but also provides you with greater creative freedom. As browser support gets better, you should carefully look at your CSS and improve it using functions like
.
calc()
As a beginner, what basic CSS functions should I understand?
CSS function is used to set the value of the CSS attribute. Some basic functions that every beginner should know include
,, rgb()
, rgba()
, hsl()
, and hsla()
. The calc()
and rgb()
functions are used to define colors, while the rgba()
and hsl()
functions are used to define colors based on hue, saturation, and brightness. The hsla()
function allows you to perform calculations to determine the CSS attribute value. calc()
The
function in in CSS is used to perform calculations that can be used as attribute values. This function can use the " " (addition), "-" (subtraction), "*" (multiple), and "/" (division) mathematical operators. For example, you can use calc()
to create a div that always minus 20 pixels for viewport width 50% as shown below: calc()
. div { width: calc(50% – 20px); }
The main difference between the
and functions in rgb()
CSS is that rgba()
contains an alpha channel that specifies the opacity of the color. The alpha parameter is a number between 0.0 (fully transparent) and 1.0 (fully opaque). For example, to set translucent red, you can use: rgba()
. color: rgba(255, 0, 0, 0.5);
How to create gradients using CSS functions?
CSS functions can be used to create gradients using the linear-gradient()
and radial-gradient()
functions. For example, to create a linear gradient from red to blue, you can use: background: linear-gradient(red, blue);
. Similarly, to create a radial gradient from the center red to the edge of the blue, you can use: background: radial-gradient(red, blue);
.
Can I convert elements using CSS functions?
Yes, CSS functions can be used to convert elements. The transform
attribute can be used with various functions such as rotate()
, scale()
, skew()
, and translate()
to modify the appearance of an element. For example, to rotate an element 45 degrees, you can use: transform: rotate(45deg);
.
What is the purpose of the attr() function in CSS?
The function in attr()
in CSS is used to return the attribute value of the selected element. This is useful for generating content, etc. For example, you can use attr()
to display the value of the link's "href" property in the tooltip: a:after { content: attr(href); }
.
How to use the var() function in CSS?
The var()
function in CSS is used to insert the value of a custom property (also known as a "CSS variable". For example, you can define a custom property --main-color: blue;
and then use it in CSS as follows: color: var(--main-color);
.
Can I create animations using CSS functions?
Yes, CSS functions can be used to create animations. The animation
attribute in CSS is abbreviated attribute for eight different attributes, including animation-name
, animation-duration
, animation-timing-function
, etc. For example, to create a 2-second animation called "slidein", you can use: animation: slidein 2s;
.
url() function in CSS?
The function in url()
in CSS is used to include files. The most common use of functions is to link to external stylesheets or include images. For example, to set the background image of an element, you can use: url()
. background-image: url('image.jpg');
Yes, the CSS function can be used to create 3D transformations. The
attribute in CSS can be used with functions such as transform
, rotateX()
, rotateY()
, rotateZ()
, scale3d()
, and translate3d()
to create 3D transformations. For example, to rotate an element around the X-axis, you can use: transform: rotateX(45deg);
.
Please note that I have tried my best to pseudo-originalize the original text and preserve the original format and location of the image. Since I cannot access the CodePen and Can I Use websites, I replaced the related link with placeholders. Please find and insert the correct link yourself.
The above is the detailed content of 8 Clever Tricks with CSS Functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










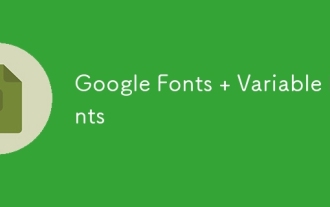
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
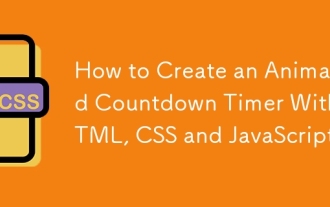
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
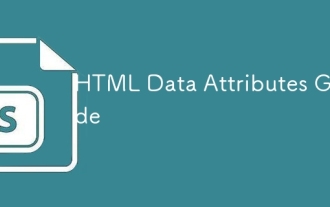
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
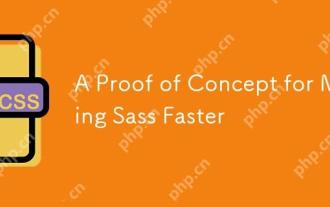
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
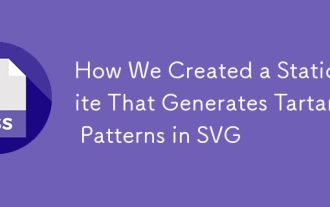
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
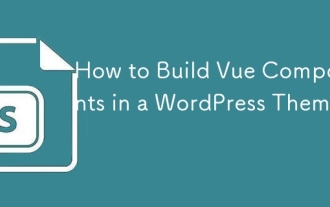
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
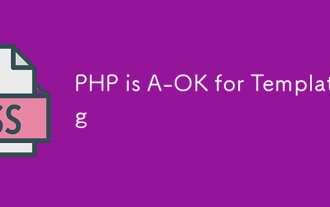
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
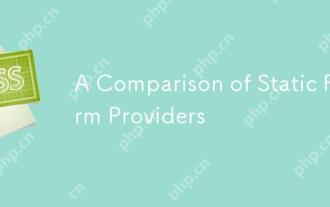
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
