Developing a Password Strength Plugin with jQuery
Passwords remain a cornerstone of online security, even with the rise of multi-factor authentication. This article guides you through creating a robust jQuery password strength plugin using the zxcvbn library. This plugin helps users generate stronger, more secure passwords.
Key Features:
- Leverages zxcvbn for realistic password strength assessment, considering factors like common words, patterns, and sequences.
- Provides clear feedback to users (very weak, weak, medium, strong, etc.) based on password strength.
- Offers customizable options for minimum/maximum password length and blacklisted words.
- Easily integrates with existing web forms.
- Compatible with modern browsers.
Introduction:
Even tech-savvy users struggle to judge password strength accurately. A password strength meter provides real-time feedback, guiding users towards stronger passwords and enhancing overall website security. Unlike simple length-based checks, zxcvbn offers a more sophisticated evaluation.
Password Strength Calculation: Beyond Simple Rules
Traditional methods often rely on basic criteria (length, uppercase/lowercase/numbers/symbols). This is insufficient. zxcvbn provides a more realistic assessment by considering a vast dataset of common words and patterns.
zxcvbn: A Realistic Approach
The zxcvbn library offers a superior method for evaluating password strength. It analyzes passwords against a comprehensive database, providing a score (0-4) and estimated cracking time. This score is far more accurate than simple rule-based checks.
The zxcvbn()
function takes the password and an optional blacklist (e.g., usernames) as input, returning an object with properties like:
-
entropy
: Password strength in bits. -
crack_time
: Estimated cracking time. -
crack_time_display
: User-friendly display of cracking time (e.g., "seconds," "years"). -
score
: Strength score (0-4). -
match_sequence
: Details of patterns identified.
Building the Password Strength Meter Plugin
We'll build a reusable jQuery plugin using jQuery Plugin Boilerplate. This approach prioritizes flexibility, allowing for easy integration into various website designs.
Plugin Requirements:
- Basic validation (empty fields, password/confirm password match).
- Customizable validation (minimum/maximum length, disallowed characters).
- Clear strength levels (very weak, weak, medium, strong).
- Optional advanced strength assessment using
crack_time_display
.
Implementation:
-
Include necessary files: jQuery, zxcvbn, and the jQuery plugin boilerplate.
-
Modify the plugin boilerplate: Rename the boilerplate file (e.g.,
jquery.password.strength.js
), update the plugin name (PasswordStrengthManager
), and define default options:
var pluginName = "PasswordStrengthManager", defaults = { password: "", confirm_pass: "", blackList: [], minChars: "", maxChars: "", advancedStrength: false };
-
Implement the plugin: The
init()
function performs validations and callszxcvbn()
. Aswitch
statement maps thezxcvbn()
score to strength levels. Advanced strength assessment usescrack_time_display
for finer granularity. -
Custom validators: Add functions (
minChars
,maxChars
,customValidators
) for additional validation rules. -
Enable multiple initializations: Modify the boilerplate's final section to allow the plugin to be re-initialized on keyup events.
-
Usage: Include the plugin in your HTML and call it on keyup events for password and confirm password fields.
Customization and Advanced Features:
- Website Design: Easily customize the plugin's appearance using CSS.
- Non-English Support: Translate messages for internationalization.
- Strength Criteria: Adjust minimum/maximum length and blacklist.
- Form Validation Integration: Seamlessly integrate with form validation libraries.
- Backend Integration (PHP): While the plugin operates client-side, server-side validation with PHP is recommended for enhanced security.
This comprehensive guide enables you to create a powerful and customizable password strength plugin, significantly improving your website's security posture. Remember to always test thoroughly across different browsers and devices.
The above is the detailed content of Developing a Password Strength Plugin with jQuery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










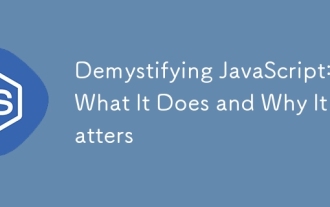
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
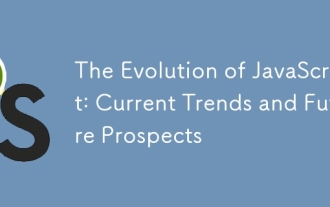
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
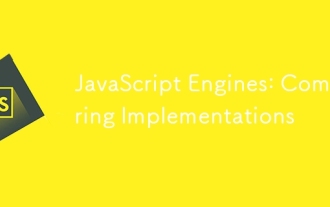
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
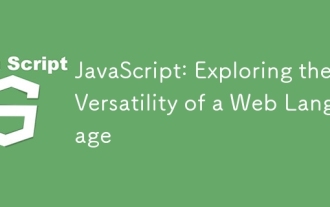
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
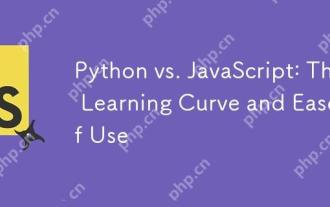
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
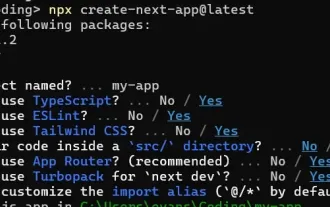
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
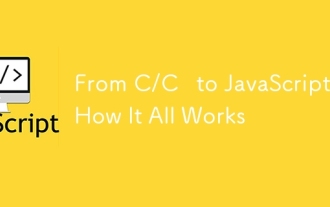
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
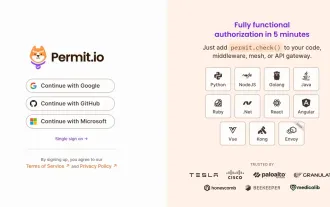
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
