Beginners Guide to DOM Selection with jQuery
As a front-end developer or designer, you must use the Document Object Model (DOM) in your daily projects. In recent years, with the improvement of JavaScript technology, selecting elements and applying dynamic functions in the DOM has become increasingly common. Therefore, we need to master the knowledge of using DOM elements to avoid conflicts and optimize performance. jQuery is one of the most popular and commonly used JavaScript libraries, with advanced DOM selection and filtering capabilities. In this article, we will explore how to filter DOM elements by considering the actual scenario with the help of jQuery. Let's get started.
Key points
- jQuery is a commonly used JavaScript library that provides advanced DOM selection and filtering capabilities. For front-end developers, it is crucial to have a deep understanding of DOM elements to avoid conflicts and optimize performance.
- HTML element tags, IDs, class, and data attributes are used to select elements in the DOM tree. ID is a unique identifier, the class is used to style elements and to filter and select elements, and the data attribute defines private data for elements.
- jQuery allows selecting unique elements, selecting multiple elements with classes, selecting elements with multiple classes, selecting elements with data attributes, and selecting elements with multiple data attributes. It is crucial to prevent duplication of elements with the same ID and to distinguish style classes for selection and functional purposes.
Introduction to ID, class and data attributes
Usually, we use HTML element tags, IDs, classes, and data attributes to select elements in the DOM tree. Most developers are familiar with using HTML tags for selection. However, novices often misuse IDs, classes, and data attributes without knowing their exact meaning and necessity in various scenarios. So, before we get into the actual scenario, let's determine each of these three options first.
- ID – Used as a unique identifier in the document. Therefore, we should not use the same ID for multiple elements, even if this is possible. Typically, we use element ID to select elements using jQuery.
- Class – Used to style elements using CSS. However, classes are usually used to filter and select elements when an ID cannot be filtered.
- Data attributes – Used to define private data for elements. We can set multiple data attributes for a single element to store all the data required by the front-end function.
Practical scenarios using ID, class, and data attributes
In this section, we will cover some common use cases for using these filtering and selecting options, and how each option can be adapted to different scenarios. In fact, the possibilities of using these options in different scenarios are endless. Therefore, we will consider the scope of this article and focus on the most important content.
Scene 1 – Select the unique element in the document
This is the most basic and common scenario we use to select any element. Selecting form values for validation can be considered a good example of such scenarios. We use HTML element ID to select elements, as shown in the following jQuery code:
$("#elementID").val();
When using this method, it is important to prevent duplication of elements with the same ID.
Scene 2 – Select multiple elements using class
Usually, when we want to filter multiple elements in a document, we use class attributes. Unlike ID, we can use multiple elements with the same CSS class. Typically, these types of similar elements are generated by loops. Suppose we have a list of records containing user data, as shown in the HTML code below. We have a "Delete" button that users can delete records by clicking them.
<table> <tr><td>Mark</td><td><button type='button' class='user_data' value='Delete'></button></td></tr> <tr><td>John</td><td><button type='button' class='user_data' value='Delete'></button></td></tr> <tr><td>Mike</td><td><button type='button' class='user_data' value='Delete'></button></td></tr> </table>
Now, we have multiple instances of similar elements, so we cannot use ID to filter the exact records. Let's see how to filter rows using the given CSS class.
var rows = $(".user_data");
In the previous code, the rows variable will contain all three rows with the class user_data. Now let's see how to delete rows when clicking a button using the class selector.
$(".user_data").click(function(){ $(this).parent().parent().remove(); });
In this technique, we iterate through all matching elements and execute the function on the current project using the $(this) object pointing to the button being clicked. This is the most widely used method to deal with multiple elements. Now let's look at some common mistakes for newbies developers in such scenarios.
- Use ID instead of class
There are many cases where developers use the same ID in a loop to generate similar HTML code instead of classes. Once the same ID is used, it only affects the first element. The rest of the elements will not work as expected. So make sure to create a dynamic ID in the loop and use the class name for element selection.
- Use class names instead of $(this) object
Another mistake many novice developers make is the lack of understanding of context and current object usage. When iterating through multiple elements, we can use jQuery $(this) to reference the current element. Many developers use class names in loops and therefore cannot get the expected results. Therefore, never use class names in a loop, as shown in the following code:
$(".user_data").click(function(){ $(".user_data").parent().parent().remove(); });
This is a widely used method of selecting elements, so it is important to understand how to use this method effectively. The CSS class is used for style purposes. But here we use it for element selection purposes. Using a CSS class for selection purposes is not the best way to do it, sometimes it can lead to layout conflicts. For example, when working with a team, developers might use only CSS classes to provide dynamic functionality for jQuery. The designer may notice that these classes are not used for styling purposes, so it is possible to delete them without knowing their importance. Therefore, it is best to use some prefix for CSS classes that are only used for various functions and not style purposes.
Scene 3 – Select elements using multiple classes
Some applications and websites rely heavily on JavaScript and provide highly dynamic client functionality. In this case, we may use a large number of classes and IDs to filter, select and apply functions. Suppose we have a list of user records that need to be filtered on the client according to the role. We can define roles as CSS classes to simplify the filtering process. Consider the following code snippet:
$("#elementID").val();
Suppose we want to acquire users with both developer and designer roles. In this case, we can use multiple CSS classes, as shown in the following code:
<table> <tr><td>Mark</td><td><button type='button' class='user_data' value='Delete'></button></td></tr> <tr><td>John</td><td><button type='button' class='user_data' value='Delete'></button></td></tr> <tr><td>Mike</td><td><button type='button' class='user_data' value='Delete'></button></td></tr> </table>
We can use any number of classes to match multiple classes on the same element one by one. Make sure not to use spaces between classes, as it will change the meaning and result of the selection. Consider the same line of code with spaces:
var rows = $(".user_data");
The code now looks for designers with parent elements called Developers. So, get used to the difference between these two implementations and use it wisely. Similarly, we can mix IDs and classes when using this technique.
Scene 4 – Select element using data attributes
HTML5 introduces custom data properties where we can define data related to HTML elements. These data attributes have a specific set of conventions and are considered private data. So now we can use the data attribute to store data related to any element instead of making multiple AJAX requests to the server. Let's see how to implement scenario 2 using custom data properties instead of CSS classes.
$(".user_data").click(function(){ $(this).parent().parent().remove(); });
As you can see, we can use data properties instead of CSS classes to get the same result. jQuery provides advanced attribute selectors to support custom data attributes. So let's consider some advanced selector options for custom properties. Suppose we store user emails as custom properties and we want to select users based on certain criteria in the email. Consider the following code snippet using data properties:
$(".user_data").click(function(){ $(".user_data").parent().parent().remove(); });
Select the element that contains "gmail" in the email
<ul> <li class='role-developer role-designer'>Mark</li> <li class='role-developer'>Simon</li> <li class='role-developer role-manager'>Kevin</li> </ul>
Select an element with the "uk" email address
var selected_users = $('.role-developer.role-designer');
Select an element for invalid emails without the "@" symbol
var selected_users = $('.role-developer .role-designer');
As you can see, data attributes are powerful in handling data related to HTML elements, and jQuery provides comprehensive support through its built-in functions. You can find the complete property selector reference at https://www.php.cn/link/2705e8fde87cd2883e9fc1f00335685f.
Scene 5 – Select elements using multiple data attributes
This is similar to what we discussed in Scene 3 and has some extensions. In advanced scenarios, using CSS classes to handle multiple data values can be complicated. So let's see how to use multiple data attributes for selection.
$("#elementID").val();
Here, we can check the existence of multiple data attributes and select through partial search values. Now you should be able to understand the difference between CSS classes and data attributes in element selection. Data attributes provide a way to organize the processing of elemental data. The techniques discussed here can be used in conjunction with other jQuery filters to meet any type of advanced situation, and it is important to use them wisely.
Summary
This article is intended to help beginners understand the basics of element IDs, classes, and custom data properties. We discuss each of them through actual scenarios with the help of jQuery built-in functions. In the process, we also identified some errors from newbies developers. Let's review the key points discussed in this article to build a useful DOM selection guide:
- Use IDs whenever possible for element selection, as it provides a unique identifier in the document.
- Use class or data attributes in multiple element scenarios instead of relying on element IDs.
- Ensure that you distinguish between style classes and classes for selection and functional purposes.
- Use data attributes in complex scenarios, because classes are not intended to provide data about elements.
I hope this article can provide you with a good introduction to element selection. If you have better guides and element selection techniques, feel free to share in the comments section.
FAQs about jQuery DOM selection (FAQ)
What is the Document Object Model (DOM) in jQuery?
The Document Object Model (DOM) in jQuery is the programming interface for HTML and XML documents. It represents the structure of a document and provides a way to manipulate content and structure. Using DOM, programmers can create, navigate, and modify elements and content in HTML and XML documents.
How to simplify DOM operations in jQuery?
jQuery simplifies DOM operations by providing an easy-to-use API to handle events, create animations, and develop Ajax applications. jQuery also provides a simple syntax to navigate and manipulate DOM. It abstracts the many complexities of handling raw JavaScript and DOM APIs, making it easier for developers to use HTML documents.
What are the different types of DOM selectors in jQuery?
There are several types of DOM selectors in jQuery, including element selectors, ID selectors, class selectors, attribute selectors, and pseudo-class selectors. Element selector selects elements based on their element name. The ID selector selects elements based on its ID attribute. The class selector selects elements based on its class attributes. The attribute selector selects elements based on attributes or attribute values. The pseudo-class selector selects elements based on a specific state.
How to select an element by its ID in jQuery?
To select an element by its ID in jQuery, use the pound sign (#) followed by the element's ID. For example, to select an element with ID "myElement", you will use $("#myElement").
How to select elements by their class in jQuery?
To select elements by their class in jQuery, use dot notation (.) followed by the class name. For example, to select an element with class "myClass", you will use $(".myClass").
What are property selectors in jQuery and how do they work?
The attribute selector in jQuery selects elements based on its attribute or attribute value. They are written in square brackets ([]). For example, to select all elements with the "data-example" attribute, you will use $("[data-example]").
How to select multiple elements in jQuery?
You can select multiple elements in jQuery by separating each selector with commas. For example, to select all elements with class "myClass" and all elements with ID "myID", you will use $(".myClass, #myID").
What is a pseudo-class selector in jQuery?
The pseudo-class selector in jQuery selects elements based on a specific state. For example, the ":checked" pseudo-class selector will select all selected check boxes or radio buttons.
How to use jQuery to operate DOM?
jQuery provides several methods for manipulating DOM, such as .append(), .prepend(), .after(), .before(), .remove(), and .empty(). These methods allow you to insert, replace, and delete elements in the DOM.
How does jQuery handle events in DOM?
jQuery provides methods for handling events in the DOM, such as .click(), .dblclick(), .hover(), .mousedown(), .mouseup(), .mousemove(), .mouseout(), . mouseover(), .mouseenter(), .mouseleave(), .change(), .focus(), .blur(), .keydown(), .keypress(), .keyup(), .submit(), . load(), .resize(), .scroll(), and .unload(). These methods allow you to define what happens when these events occur on elements in the DOM.
The above is the detailed content of Beginners Guide to DOM Selection with jQuery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
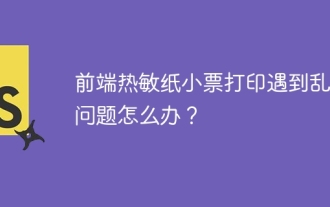
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
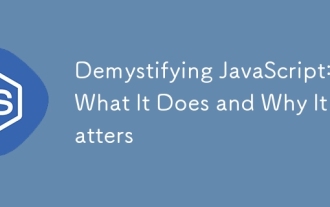
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
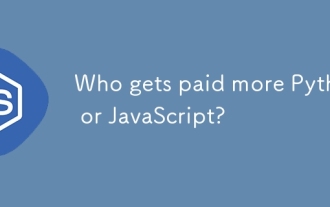
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
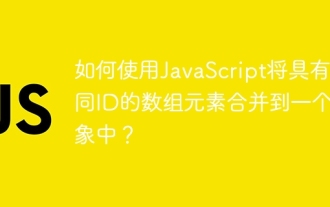
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
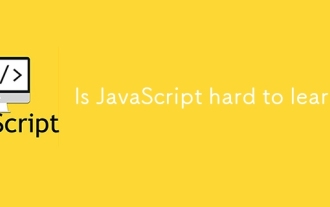
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
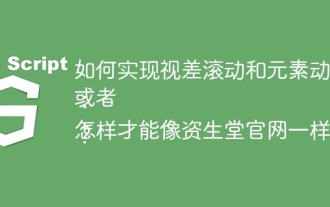
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
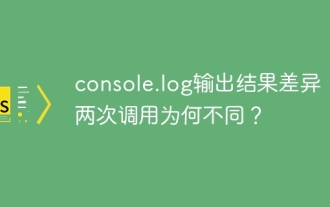
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
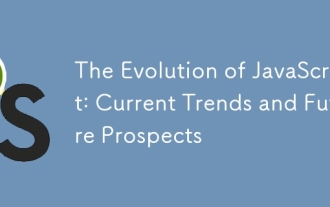
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
