PHP Master | Build a CRUD App with Yii in Minutes
Yii Framework: A Guide to Quickly Building Efficient CRUD Applications
Yii is a high-performance PHP framework known for its speed, security, and good support for Web 2.0 applications. It follows the principle of "convention over configuration", which means that much less code can be written than other frameworks (less code means fewer bugs) as long as it follows its specifications. In addition, Yii also provides many convenient features out of the box, such as scaffolding, data access objects, themes, access control, cache, and more. This article will introduce the basics of using Yii to create a CRUD system.
Key Points
- Yii is a high-performance framework suitable for Web 2.0 applications and provides many convenient features such as scaffolding, data access objects, themes, access control and caching. It follows the principle of conventions over configuration, reducing the amount of code, thereby reducing the possibility of bugs.
- Yii's command line tool
yiic
is used to create skeleton applications with appropriate directory structure. Yii follows the MVC and OOP principles, and the URL structure ishttp://localhost/yiitest/index.php?r=controllerID/actionID
. The controller and the method to be called are determined based on the ID in the URL. - Yii's web-based tool is used to generate models, controllers, and forms for CRUD operations, so as to quickly develop CRUD systems. For example, a simple system where users can perform CRUD operations on blog posts can be developed in minutes.
gii
Beginner
Suppose you have Apache, PHP (5.1 or later) and MySQL installed on your system, so the first step is to download the framework file. Visit Yii's official website and download the latest stable version (1.1.13 at the time of writing). Unzip the ZIP file to get the folder (the version identifier may vary depending on the version you downloaded), rename it to yii-1.1.13.e9e4a0
, and move it to your web-accessible root directory. In my system, this is yii
, so the path to the framework file will be C:\wamp\www
. In this article, I'll call it C:\wamp\www\yii
so that you can easily follow the action even if your settings are different. Next, we should check which features of Yii will be supported by our system. Visit <yiiroot></yiiroot>
in your browser to view the requirements details of the framework. Since we will use a MySQL database, you should enable the MYSQL PDO extension. http://localhost/yii/requirements/
Continue to move forward
Each web application has a directory structure, and Yii application also needs to maintain a hierarchical structure within the web root directory. To create a skeleton application using the appropriate directory structure, we can use Yii's command line tool yiic
. Navigate to the web root directory and type the following:
<yiiroot>/framework/yiic webapp yiitest
This will create a skeleton application called yiitest
with the minimum required files. In it you will find index.php
, which is used as an entry script; it accepts user requests and decides which controller should handle the request. Yii is based on MVC and OOP principles, so you should be familiar with these topics. If you are not familiar with MVC, please read the SitePoint series article "MVC Mode and PHP", which provides a great introduction. The Yii URL looks like http://localhost/yiitest/index.php?r=controllerID/actionID
. For example, in a blog system, the URL might be http://localhost/yiitest/index.php?r=post/create
. post
is the controller ID, create
is the operation ID. The entry script determines which controller and method to call based on the ID. The controller with ID post
must be named PostController
(ID removes the suffix "Controller" from the class name and converts the first letter to lowercase). The operation ID is the ID of the method in the controller that exists in a similar way; in PostController
, there will be a method called actionCreate()
. There may be multiple views associated with the controller, so we save the view file in the protected/views/*controllerID*
folder. We can create a view file named create.php
for our controller in the above directory and then just write the following code to present this view to the user:
public function actionCreate() { $this->render('create'); }
If necessary, other data can also be passed to the view. The operation is as follows:
$this->render('create', array('data' => $data_item));
In the view file, we can access the data through the $data
variable. The view can also access $this
, which points to the controller instance that renders it. Also, if you want a user-friendly URL, you can uncomment the following in protected/config/main.php
:
'urlManager'=>array( 'urlFormat'=>'path', 'rules'=>array( '<w>/<d>'=>'<controller>/view', '<w>/<w>/<d>'=>'<controller>/<action>', '<w>/<w>'=>'<controller>/<action>', ) )
Then the URL will look like http://localhost/yiitest/controllerID/actionID
.
Develop CRUD Application
Now that you have learned the important Yii conventions, it's time to start using CRUD. In this section, we will develop a simple system where users can perform CRUD operations (create, retrieve, update, and delete) on blog posts.
Step 1
Create a MySQL database yiitest
and create a table called posts
in it. For the purposes of this article, the table will have only 3 columns: id, title, and content.
CREATE TABLE posts ( id INTEGER NOT NULL PRIMARY KEY AUTO_INCREMENT, title VARCHAR(100), content TEXT )
Open the application's configuration file (protected/config/main.php
) and uncomment the following:
'db'=>array( 'connectionString' => 'mysql:host=localhost;dbname=yiitest', 'emulatePrepare' => true, 'username' => 'root', 'password' => '', 'charset' => 'utf8', )
Replace testdrive
with our database name, i.e. yiitest
. Obviously, you should also provide the credentials you need for the Yii connection.
Step 2
In Yii, each database table should have a model class of the corresponding type CActiveRecord
. The advantage is that we don't have to process database tables directly. Instead, we can use model objects corresponding to different rows of the table. For example, the Post
class is a model of the posts
table. An object of this class represents a row from the posts
table and has attributes representing the column value. To quickly generate the model, we will use Yii's web-based tool gii
. This tool can be used to generate models, controllers, and forms for CRUD operations. To use gii
in your project, find the following in the application's configuration file and uncomment it and add a password.
<yiiroot>/framework/yiic webapp yiitest
Then use the following URL to access gii
: http://localhost/yiitest/index.php?r=gii
. If you are using a user-friendly URL, the URL is: http://localhost/yiitest/gii
. Click Model Builder. gii
You will be asked for the table name; enter "posts" for the table name and use the name "Post" for the model. Then click Generate to create the model.
Checkprotected/models
, where you will find the file Post.php
.
Step 3
Now click on CRUD generator. Enter the model name as "Post". The controller ID will be automatically populated as "post". This means that a new controller will be generated under the PostController.php
name. Click Generate. This process will generate the controller and several view files for CRUD operations.
Now you have a brand new CRUD application! Click the "Try Now" link to test it. To manage posts, you need to log in as admin/admin
. To create a new post you need to visit http://localhost/yiitest/post/create
, to update a specific post, just point your browser to http://localhost/yiitest/post/update/postID
. Again, you can list all posts and delete some or all of them.
Conclusion
Yii is very powerful in developing Web 2.0 projects. In fact, we just saw how easy it is to create a fully functional CRUD system in just a few minutes! Obviously, Yii can save you a lot of work because you don't have to start from scratch. Yii provides us with the foundation we can expand as needed.
(The subsequent FAQ part is too long, it is recommended to organize it into a separate document.)
The above is the detailed content of PHP Master | Build a CRUD App with Yii in Minutes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


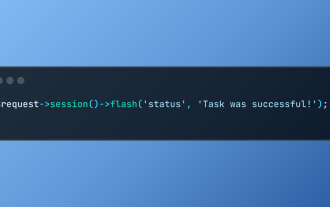
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
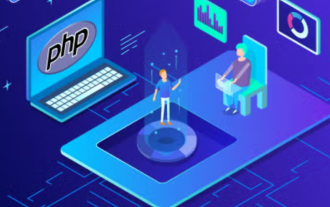
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
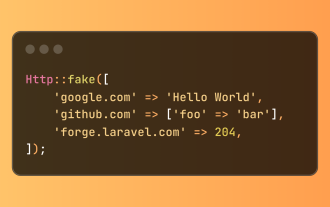
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
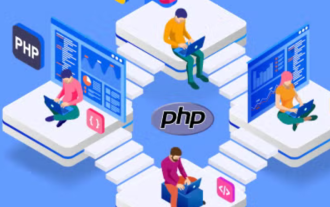
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
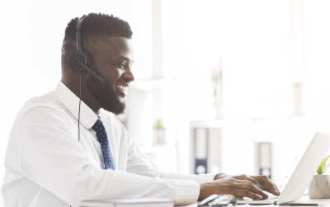
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
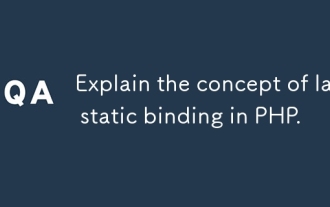
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
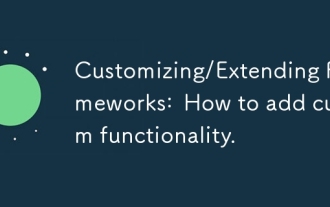
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
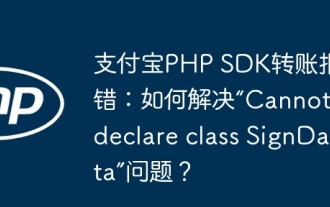
Alipay PHP...
