PHP Master | Data Structures for PHP Devs: Trees
This article introduces tree data structures in PHP, focusing on their hierarchical nature and efficiency in searching and sorting. It builds upon a previous article covering stacks and queues.
Key Concepts:
- Hierarchical Data: PHP tree structures represent data hierarchically, with parent-child relationships between nodes. This is ideal for representing organizational charts, file systems, or any data with inherent nesting.
- Tree Traversal: Visiting each node in a tree is called traversal. Common methods include pre-order, in-order, and post-order (depth-first searches), and level-order (breadth-first search).
- Implementation: A PHP tree is typically implemented using classes representing nodes, each containing a value and references to its children. Methods are added for insertion, deletion, and traversal.
- Tree Balancing: For efficient search, trees need balancing to ensure roughly equal subtree depths. Algorithms like AVL or Red-Black trees maintain this balance.
The Search Problem:
The article highlights the limitations of stacks and queues for value-based data retrieval. Searching a list requires traversing, on average, half the list. Trees offer a more efficient solution. The core operations for a tree-based "table" are: create, insert, delete, and retrieve, mirroring database CRUD operations.
Trees: A Superior Solution:
Trees combine the advantages of sequential and linked list implementations, offering efficient operations. Many database systems (MySQL's MyISAM, file systems (HFS , NTFS, btrfs) utilize trees for indexing.
The diagram illustrates a binary tree – a tree where each node has at most two children. This is a recursive structure.
Binary Tree Implementation:
A basic binary tree implementation in PHP is shown, using BinaryNode
and BinaryTree
classes. BinaryNode
holds a value and references to left and right children. BinaryTree
manages the root node.
Node Insertion:
A simple insertion algorithm is described using pseudocode. It uses a divide-and-conquer approach: new nodes are inserted to the left if smaller than the current node's value, and to the right if larger. Duplicates are rejected. The PHP code demonstrates a recursive implementation of this algorithm. Node deletion is mentioned but deferred to a future article.
Tree Traversal (In-Order):
The article explains in-order traversal, where the left subtree is processed, then the current node, then the right subtree. Modified BinaryNode
and BinaryTree
classes demonstrate in-order traversal using a recursive dump()
method.
Conclusion:
The article concludes by summarizing the introduction to binary trees, node insertion, and in-order traversal. Future articles will cover breadth-first search and other data structures.
Frequently Asked Questions (FAQs):
The FAQs section provides further explanation on various aspects of PHP tree data structures, including their significance, implementation details, relationship with SPL, usage in databases and machine learning, performance considerations, tree balancing, and visualization techniques.
The above is the detailed content of PHP Master | Data Structures for PHP Devs: Trees. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










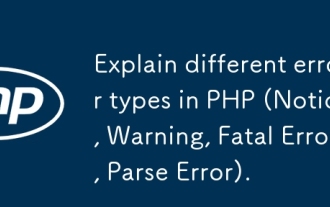
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
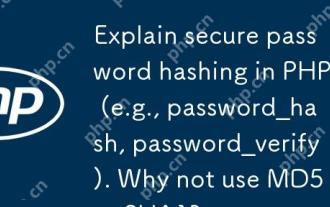
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
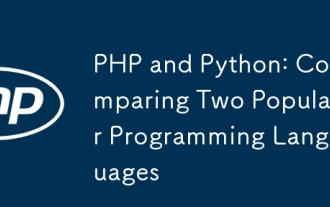
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
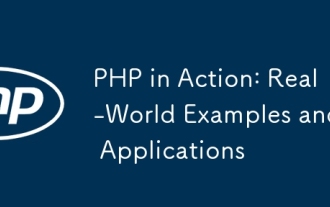
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
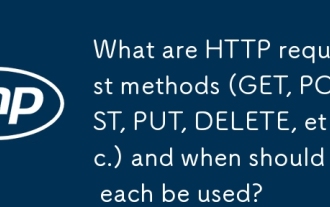
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
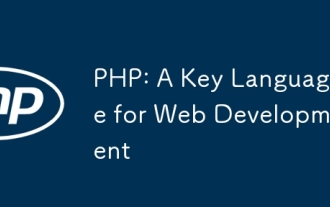
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
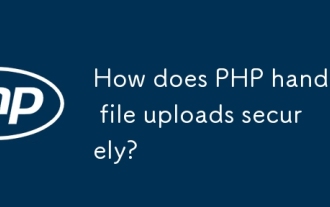
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
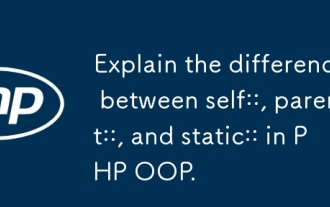
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
