jQuery refresh page on browser resize
Use JavaScript to refresh the basic code snippet of the browser's resized page.
// 浏览器大小调整时刷新页面 $(window).bind('resize', function(e) { console.log('窗口大小已调整..'); this.location.reload(false); /* false 从缓存获取页面 */ /* true 从服务器获取页面 */ });
If window.location.reload()
does not work in Firefox, try the following:
// 浏览器大小调整时刷新页面 $(window).bind('resize', function(e) { if (window.RT) clearTimeout(window.RT); window.RT = setTimeout(function() { this.location.reload(false); /* false 从缓存获取页面 */ }, 200); });
jQuery Page Refresh FAQ (FAQ)
How to refresh the page using jQuery when resizing the browser?
To use jQuery to refresh the page when your browser is resized, you can use the $(window).resize()
method. This method triggers a resize event or attaches a function that runs when a resize event occurs. Here is a simple code snippet that demonstrates this:
$(window).resize(function() { location.reload(); });
In this code, $(window).resize()
is an event that fires when the browser window is resized. The location.reload()
function is used to refresh the page.
location.reload()
What is the role of jQuery?
The location.reload()
function in jQuery is used to refresh or reload the page. It is equivalent to the refresh button in the browser. When this function is executed, it forces the browser to refresh the current page. The location.reload()
function is very useful when you want to reload the current document, which may be necessary in various situations, such as dynamically updating content or refreshing the page when resizing the browser.
Can I refresh the page without using jQuery?
Yes, you can refresh the page without using jQuery. JavaScript provides a native way to refresh pages, i.e. window.location.reload()
. This method works similarly to the location.reload()
function in jQuery. Here is an example:
window.location.reload();
When this line of code is executed, the current page will be refreshed.
How to use jQuery to automatically refresh the page after a specific time interval?
To automatically refresh the page after a specific time interval, you can use the setTimeout()
function with the location.reload()
function. Here is an example:
setTimeout(function(){ location.reload(); }, 5000);
In this code, the setTimeout()
function will wait 5000 milliseconds (or 5 seconds), and then it will execute the location.reload()
function to refresh the page.
Is there a way to refresh part of the page using only jQuery?
Yes, you can use jQuery to refresh only part of the page. This is usually done using AJAX (asynchronous JavaScript and XML). With AJAX, you can update a part of a web page without reloading the entire page. Here is a basic example:
$("#div1").load("demo_test.txt");
In this code, the load()
function is used to load data from the "demo_test.txt" file into the "div1" element.
How to use jQuery to prevent page refresh?
To use jQuery to prevent page refresh, you can use the event.preventDefault()
method. This method prevents the default operation of the event from happening. For example, if you have a form submission event that refreshes the page, you can prevent it from happening like this:
// 浏览器大小调整时刷新页面 $(window).bind('resize', function(e) { console.log('窗口大小已调整..'); this.location.reload(false); /* false 从缓存获取页面 */ /* true 从服务器获取页面 */ });
In this code, the event.preventDefault()
method prevents the form from being submitted, thus preventing page refresh.
Can I refresh the page when the button is clicked using jQuery?
Yes, you can refresh the page when you click a button using jQuery. You can do this by attaching the click event to a button and then refreshing the page with the location.reload()
function. Here is an example:
// 浏览器大小调整时刷新页面 $(window).bind('resize', function(e) { if (window.RT) clearTimeout(window.RT); window.RT = setTimeout(function() { this.location.reload(false); /* false 从缓存获取页面 */ }, 200); });
In this code, the page will be refreshed when the button with ID "myButton" is clicked.
How to use jQuery to refresh the page without using cache?
To use jQuery to refresh the page without using cache, you can use the location.reload(true)
function. The reload()
parameter in the true
function forces the browser to reload the page from the server, bypassing the cache. Here is an example:
$(window).resize(function() { location.reload(); });
When executing this line of code, the current page will be refreshed from the server, not from the cache.
Can I refresh the page when a specific event occurs using jQuery?
Yes, you can use jQuery to refresh the page when a specific event occurs. jQuery provides various event methods such as click()
, dblclick()
, keydown()
, keyup()
, mousedown()
, mouseup()
, hover()
, scroll()
, location.reload()
,
function when these events occur.
How to refresh a page using jQuery within a specific time interval? setInterval()
window.location.reload();
setInterval()
The above is the detailed content of jQuery refresh page on browser resize. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


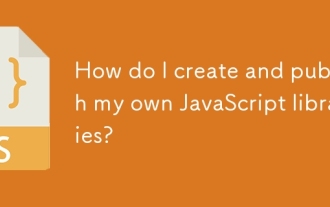
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
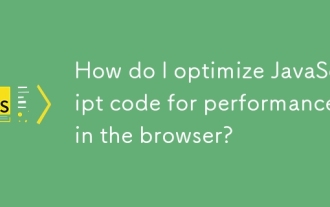
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
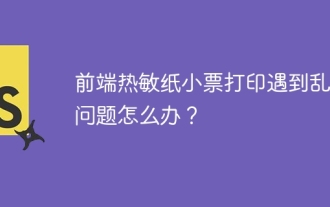
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
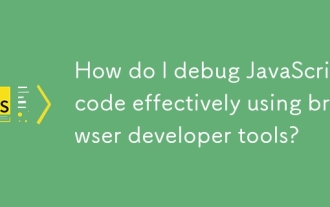
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
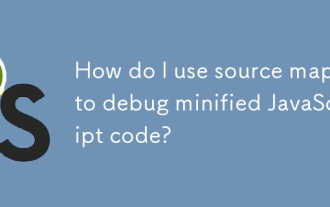
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
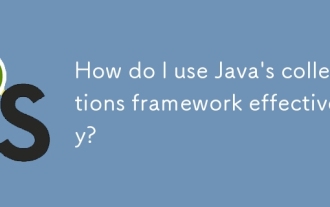
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
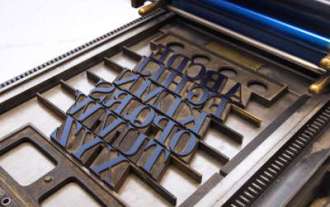
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
