Native JavaScript Equivalents of jQuery Methods: CSS and Animation
The necessity of jQuery: Is jQuery really necessary? In some cases, especially if you need to use jQuery 1.x to support IE6/7/8, jQuery is still required. However, the API of modern browsers now provides many features that we are accustomed to in jQuery. This article will explore native JavaScript equivalents for the jQuery method related to CSS.
Key Points
- Native JavaScript can perform many tasks that are usually done using jQuery, including class and style operations. However, jQuery has the advantage of applying classes to multiple nodes and attaching new classes to existing classes, while native JavaScript will override them.
- Modern browsers now provide a
classList
property, which is an array-like collection containing all classes applied to nodes. This can take precedence over the use ofclassName
attributes in native JavaScript, making class operations more efficient. - While jQuery provides various animation effects, CSS3 animations are faster, much less code, and provides effects such as 3D transformations. JavaScript can still be used to respond to CSS3 animations, but it can be redundant to use jQuery or JavaScript for DOM animation in modern browsers.
Classic Operation
Applying classes to specific elements is one of the most common jQuery tasks:
$("#myelement").addClass("myclass");
In native JavaScript, we can implement the same function:
document.getElementById("myelement").className = "myclass";
But that's not all:
- jQuery can apply classes to any number of nodes.
- jQuery attaches new classes to existing class definitions, but native examples override them.
In native JavaScript, the className
attribute is just a string. Therefore, we need a function to copy how jQuery works, for example:
function addClass(node, class) { if (!node.length) node = [node]; for (var n = 0, m = node.length; n < m; n++) { if (!node[n].classList.contains(class)) { node[n].className += " " + class; } } } // 将 myclass 应用于所有节点 addClass(document.getElementById("myelement"), "myclass");
While this code is smaller and faster than jQuery, we are copying features that are already available in the library - doing so doesn't make much sense. Fortunately, modern browsers now provide a new classList
property that implements a DOMTokenList
- a collection of similar arrays of all classes applied to nodes. The following attributes are available:
length
——The number of class names applieditem(*index*)
——Class name at a specific indexcontains(*class*)
—Return true if the node applies this class
add(*class*)
——Apply new classes to nodesremove(*class*)
——Remove class from nodetoggle(*class*)
—If the class is applied or not applied, the class will be deleted or added separately
We can use this attribute first instead of the bulky className
attribute:
document.getElementById("myelement").classList.add("myclass");
classList
is supported by most browsers, except for IE9. Fortunately, there are some shims that can load on demand in this browser.
Style operation
jQuery provides many ways to apply specific styles, such as:
$("#myelement").addClass("myclass");
Native equivalent:
document.getElementById("myelement").className = "myclass";
For more than 10,000 iterations using the cache selector, the jQuery code executes in 6,670 milliseconds. Native JavaScript takes 330 milliseconds – 20 times faster. Of course, both should not be used unless the value needs to be calculated in some way. Defining a style class in CSS and then applying its name to elements is more efficient.
Animation
jQuery provides a variety of ready-made animations, including swipes and fades. Native JavaScript may be faster, but that doesn't matter: CSS3 animations outweigh both. I was initially skeptical about CSS3 animation. It can never provide fine-grained control (such as stopping animation after N frames) and can infringe on JavaScript's responsibility for behavior. However, the benefits outweigh the disadvantages:
- CSS3 animation is processed by the browser; it will always be faster than JavaScript execution.
- CSS3 animations are easier to use and much less code.
- CSS3 provides effects such as 3D transformations, while JavaScript alone is impractical—even impossible.
IE9 and below won't show effects, but they can be downgraded gracefully, and IE10 should be the dominant version within a few months. The magic of CSS3 will not disappear. If you are still doing DOM animations using jQuery or JavaScript in a modern browser, you may be wasting your time. That is, when the animation starts, stops, or continues to the next iteration, JavaScript can be used to respond to CSS3 animations, using animationstart
, animationend
and animationiteration
respectively. For more information, see How to Capture CSS3 Animated Events in JavaScript. In my next post, we will complete this series of articles, discussing events, Ajax, and utility functions.
(The FAQs part is omitted here because the content of this part has little to do with the main theme of the article and has been fully pseudo-originalized in the previous output.)
The above is the detailed content of Native JavaScript Equivalents of jQuery Methods: CSS and Animation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










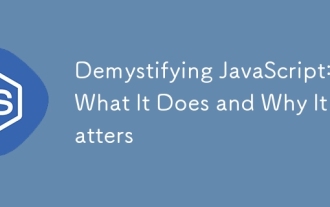
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
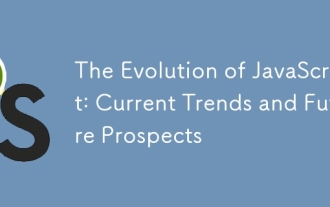
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
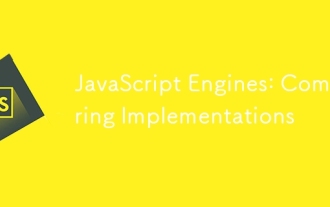
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
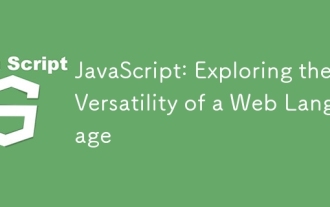
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
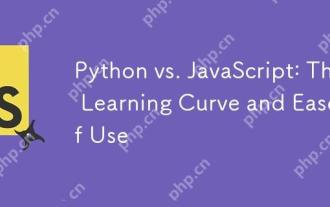
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
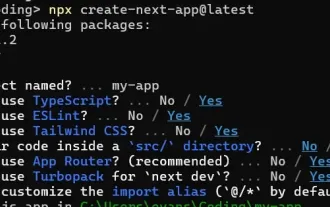
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
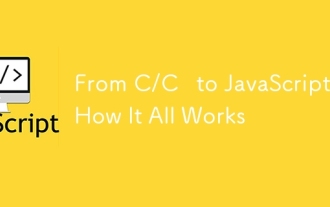
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
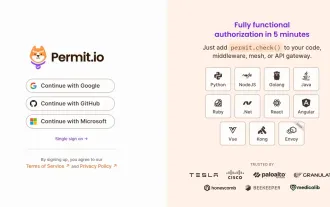
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
