Hashing Passwords with the PHP 5.5 Password Hashing API
Core points
- PHP 5.5 Password Hash API simplifies password hashing with four functions:
password_hash()
for hashing passwords,password_verify()
for verifying passwords with their hash values,password_needs_rehash()
for checking if passwords need to be re-replaced Hash,password_get_info()
Used to return the name of the hash algorithm and various options used in the hashing process. - This API uses the bcrypt algorithm by default and automatically handles the generation of salt values without the need for developers to provide it. However, developers can still provide their own salt or cost values by passing a third parameter to the
password_hash()
function. - This API is considered very secure, but it is recommended to use it as part of a comprehensive security policy. Developers using PHP 5.3.7 or later can use a library called
password_compat
that emulates the API and automatically disables itself after the PHP version is upgraded to 5.5.
Using bcrypt is currently recognized as the best password hashing practice, but many developers are still using older and weaker algorithms like MD5 and SHA1. Some developers don't even use salt when hashing. The new hash API in PHP 5.5 is designed to attract attention to bcrypt while hiding its complexity. In this article, I will cover the basics of using the PHP new hash API. The new password hash API exposes four simple functions:
-
password_hash()
– Used to hash the password. -
password_verify()
– Used to verify passwords based on their hash value. -
password_needs_rehash()
– Used when rehashing the password. -
password_get_info()
– Returns the name of the hashing algorithm and the various options used in the hashing process.
password_hash()
Although the crypt()
function is safe, many people think it is too complex and error-prone. Then some developers use weak salt and weak algorithms to generate hashes, such as:
<?php $hash = md5($password . $salt); // 可行,但危险
However, the password_hash()
function simplifies our work and our code can be kept safe. When you need a hash password, just give it to the function and it will return a hash value that can be stored in the database.
<?php $hash = md5($password . $salt); // 可行,但危险
That's it! The first parameter is the password string to have, and the second parameter specifies the algorithm applied to generate the hash. The current default algorithm is bcrypt, but sometime in the future it may add a more powerful algorithm as the default algorithm and may generate larger strings. If you are using PASSWORD_DEFAULT
in your project, make sure to store the hash in a column with a capacity of more than 60 characters. Setting the column size to 255 may be a good choice. You can also use PASSWORD_BCRYPT
as the second parameter. In this case, the result is always 60 characters long. It is important here that you do not have to provide salt value or cost parameters. The new API will handle all of this for you. Salt is part of the hash, so you don't have to store it separately. If you want to provide your own salt (or cost), you can do it by passing a third parameter (an option array) to the function.
<?php $hash = password_hash($password, PASSWORD_DEFAULT);
In this way, you can always use the latest security measures. If PHP later decides to implement a more powerful hashing algorithm, your code can take advantage of it.
password_verify()
Now that you have learned how to generate hashes using the new API, let's see how to verify your password. Remember that you store the hash in the database, but what you get is a plain text password when the user logs in. The password_verify()
function takes a plain text password and a hash string as its two parameters. Returns true if the hash matches the specified password.
<?php $options = [ 'salt' => custom_function_for_salt(), //编写您自己的代码以生成合适的盐 'cost' => 12 // 默认成本为 10 ]; $hash = password_hash($password, PASSWORD_DEFAULT, $options);
Remember that salt is part of the hash password, which is why we don't specify it separately here.
password_needs_rehash()
What should I do if I need to modify the salt and cost parameters of the hash string? This is a concern because you may decide to improve safety by adding stronger salt or larger cost parameters. Additionally, PHP may change the default implementation of the hashing algorithm. In all these cases, you want to rehash the existing password. password_needs_rehash()
Helps check if a specified hash implements a specific algorithm and uses specific options (such as cost and salt) when created.
<?php if (password_verify($password, $hash)) { // 成功! } else { // 无效的凭据 }
Remember that when a user tries to log into your website, you need to do this because it's just the time when you can access your plain text password.
password_get_info()
password_get_info()
Accepts a hash and returns an associative array containing three elements:
-
algo
– A constant that identifies a specific algorithm -
algoName
– The name of the algorithm used -
options
– Various options used when generating hash
Conclusion
The new password hash API is easier to use than using the crypt()
function. If your website is currently running on PHP 5.5, then I highly recommend using the new hash API. Those using PHP 5.3.7 (or later) can use a library called password_compat
, which emulates the API and automatically disables itself after the PHP version is upgraded to 5.5.
PHP 5.5 Password Hash API FAQ (FAQ)
What is the PHP 5.5 Password Hash API and why is it important?
PHP 5.5 Password Hash API is a feature in PHP 5.5 and later that provides developers with an easy way to hash and verify passwords in a secure way. It is important because it helps protect sensitive user data. If the database is hacked, hash passwords are harder to crack than plain text passwords. The API uses the powerful hash function Bcrypt by default and automatically handles the generation of salt values, making it easier for developers to implement secure password processing.
password_hash
How does the function work?
The password_hash
function is part of the PHP 5.5 Password Hash API. It receives a plain text password and hash algorithm as input and returns a hash password. The function also automatically generates and applies a random salt value to the password before hashing. This salt value is contained in the returned hash, so it is not necessary to store it separately.
password_verify
What is the purpose of the function?
password_verify
Function is used to verify passwords based on hash passwords. It receives a plain text password and a hash password as input. This function extracts salt values and hashing algorithms from the hashed password, applies them to the plain text password, and then compares the results with the original hashed password. If it matches, the function returns true, indicating that the password is correct.
How is the security of the PHP 5.5 Password Hash API?
PHP 5.5 Password Hash API is considered very secure. It uses the Bcrypt hashing algorithm by default, which is a powerful hashing function. The API also automatically generates and applies a random salt value for each password, which helps prevent rainbow table attacks. However, like all security measures, it is not foolproof and should be used as part of a comprehensive security policy.
Can I use custom salt in the password_hash
function?
Yes, you can use custom salt in the password_hash
function, but this is not recommended. This function automatically generates a random salt value for each password, which is usually safer than custom salt. If you do choose to use a custom salt, it should be a random string of at least 22 characters.
password_hash
What are the cost parameters in the function?
password_hash
The cost parameters in the function determine the computational cost of the hash. Higher costs make hash safer, but also slower calculations. The default cost is 10, which is a good balance between security and performance for most applications.
How to check if the hash password needs to be rehashed?
You can use the password_needs_rehash
function to check if the hash password needs to be rehashed. This function receives hash password, hash algorithm, and optional cost as input. If the hash password is created with a different algorithm or cost, it returns true, indicating that it should be rehashed.
Can I use the PHP 5.5 password hash API with older versions of PHP?
PHP 5.5 Password Hash API is only available in PHP 5.5 and later. However, there is a compatibility library that provides the same functionality for PHP 5.3.7 and later.
What happens if I have the password using the PASSWORD_DEFAULT
constant hashing and then the default algorithm changes in future versions of PHP?
If you have the password using the PASSWORD_DEFAULT
constant and then the default algorithm in future versions of PHP changes, the password_hash
function will continue to work as expected. The hashed password contains information about the algorithm used, so the password_verify
function can still correctly verify the password.
Can I use the PHP 5.5 Password Hash API with non-ASCII passwords?
Yes, you can use the PHP 5.5 Password Hash API with non-ASCII passwords. The password_hash
and password_verify
functions use binary data, so they can handle passwords for any character. However, you should note that different systems may handle non-ASCII characters differently, so it is a good idea to normalize them before hashing the password.
The above is the detailed content of Hashing Passwords with the PHP 5.5 Password Hashing API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


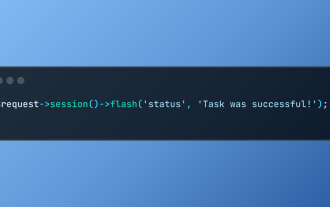
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
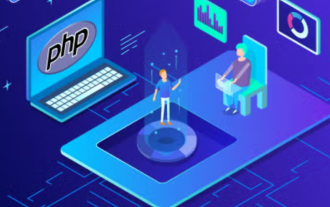
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
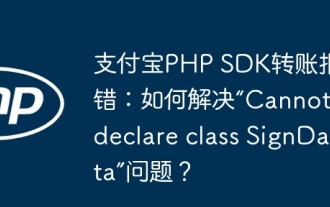
Alipay PHP...
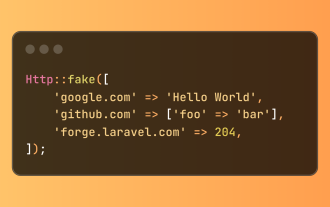
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
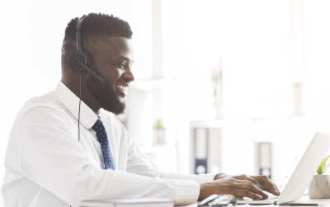
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
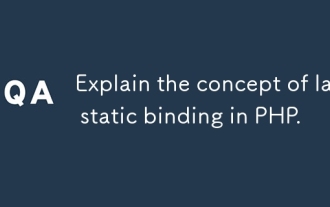
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
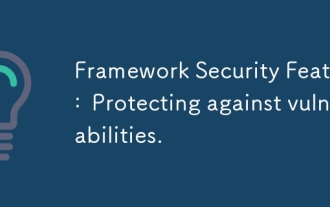
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
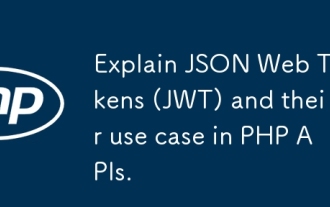
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
