Generating Random Color Values using JavaScript
This JavaScript code snippet generates a random RGB color, useful for adding dynamic color to transitions.
const randomRGBColor = () => `rgb(${Math.floor(Math.random() * 256)}, ${Math.floor(Math.random() * 256)}, ${Math.floor(Math.random() * 256)})`;
This concise function directly returns the rgb()
string.
Frequently Asked Questions (FAQs) about Generating Random Color Values in JavaScript
This section addresses common questions about generating random colors using various color models in JavaScript.
Q1: How do I create a random RGB color in JavaScript?
The RGB color model uses red, green, and blue values (0-255 each) to define a color. Here's a function:
function getRandomRGBColor() { const r = Math.floor(Math.random() * 256); const g = Math.floor(Math.random() * 256); const b = Math.floor(Math.random() * 256); return `rgb(${r}, ${g}, ${b})`; }
Q2: How can I generate a random hexadecimal color code?
Hexadecimal color codes use a # symbol followed by six hexadecimal digits (0-9, A-F). This function generates one:
function getRandomHexColor() { const hexChars = '0123456789ABCDEF'; let hexColor = '#'; for (let i = 0; i < 6; i++) { hexColor += hexChars[Math.floor(Math.random() * 16)]; } return hexColor; }
Q3: How to apply a generated random color to HTML elements?
After generating a color (using either getRandomRGBColor()
or getRandomHexColor()
), use JavaScript to set the style
property of your HTML element:
const myElement = document.getElementById('myElement'); myElement.style.backgroundColor = getRandomRGBColor();
Q4: Can I add opacity to my random color?
Yes, use RGBA (Red, Green, Blue, Alpha) or HSLA (Hue, Saturation, Lightness, Alpha). Here's an example with RGBA:
function getRandomRGBAColor() { const r = Math.floor(Math.random() * 256); const g = Math.floor(Math.random() * 256); const b = Math.floor(Math.random() * 256); const a = Math.random().toFixed(2); // Opacity (0.0 - 1.0) return `rgba(${r}, ${g}, ${b}, ${a})`; }
Q5: How about generating random colors using HSL?
HSL (Hue, Saturation, Lightness) is another color model. Hue is 0-360 degrees, saturation and lightness are 0-100%.
function getRandomHSLColor() { const h = Math.floor(Math.random() * 360); const s = Math.floor(Math.random() * 101); // 0-100% const l = Math.floor(Math.random() * 101); // 0-100% return `hsl(${h}, ${s}%, ${l}%)`; }
These functions provide flexible ways to generate random colors for various applications in your JavaScript projects. Remember to choose the color model (RGB, Hex, RGBA, HSL, HSLA) that best suits your needs.
The above is the detailed content of Generating Random Color Values using JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


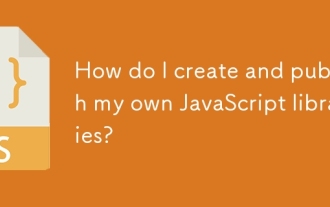
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
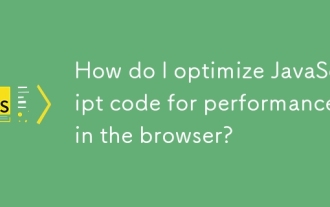
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
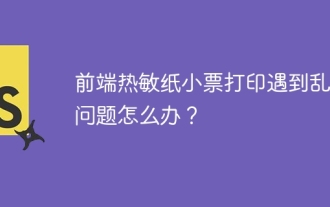
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
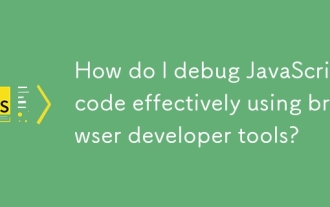
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
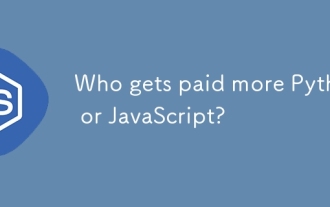
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
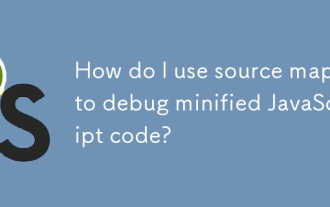
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
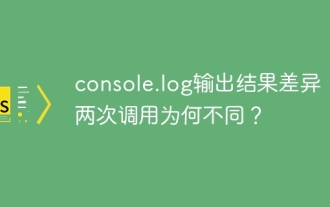
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
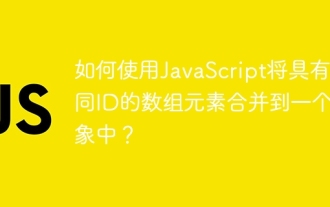
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
