


PHP Master | Extending Twig Templates: Inheritance, Filters, Functions
Leveraging Twig's Extensibility for Enhanced PHP Templating
In MVC architectures, template engines streamline dynamic content population in views. Twig excels among PHP template engines due to its robust extensibility features, particularly template inheritance, filters, and functions. This article explores these extensions for cleaner, more maintainable code.
Key Advantages of Twig's Extensibility:
- Template Inheritance: Creates reusable parent templates with defined blocks, extended and customized in child templates. This centralizes references, improving consistency.
- Filters: Modify variables within templates. Utilize built-in or custom filters for tasks like conditional styling (e.g., highlighting categories in a data grid).
- Functions: Inject dynamic content. Similar to filters, custom functions handle specific needs, such as generating form fields with required attributes, ensuring accuracy and reducing errors.
Addressing Limitations of Traditional Template Libraries:
Many template libraries lack extensibility, leading to code duplication. For instance, managing CSS and JavaScript inclusions often involves separate header and footer templates, or embedding script references directly into individual page templates. This approach becomes cumbersome and error-prone as complexity increases. Consider a scenario requiring Google Maps initialization on a single page; including the map script in every page's header is inefficient.
Twig's Solution: Template Inheritance
Twig's inheritance elegantly solves this. A parent template defines blocks:
<!DOCTYPE html> <html> <head> {% block head %} <link rel="stylesheet" href="style.css"> <🎜> {% endblock %} </head> <body> <div id="grid">{% block content %}{% endblock %}</div> <div id="footer">{% block footer %}© 2013 example.com{% endblock %}</div> </body> </html>
Child templates extend this parent, overriding specific blocks:
{% extends "parent.html" %} {% block head %} {{ parent() }} <🎜> {% endblock %} {% block content %} <h1 id="My-Page">My Page</h1> {% endblock %}
The parent()
function retains the parent's block content, allowing additive modifications.
Twig Filters: Data Transformation
Filters transform variables. A built-in example is trim()
for removing whitespace:
{{ " Variable Content " | trim }}
Custom filters extend this functionality. For instance, a filter to style categories in a product grid:
$filter = new \Twig\TwigFilter('highlight', function ($key) { switch (trim($key)) { case 'book_category': return '<span class="book">Book</span>'; case 'cd_category': return '<span class="cd">CD</span>'; // ... more cases default: return $key; } }); $twig->addFilter($filter);
Used in the template:
{% for product in products %} <div> {{ product.category | highlight }}: {{ product.product }} </div> {% endfor %}
Twig Functions: Dynamic Content Generation
Functions add dynamic content. Similar to filters, custom functions enhance template flexibility. Consider a function for generating form fields:
$function = new \Twig\TwigFunction('form_text', function ($name, $id, $value = "", $class = "form_text") { return '<input type="text" name="' . $name . '" id="' . $id . '" value="' . $value . '" class="' . $class . '">'; }); $twig->addFunction($function);
Template usage:
{{ form_text('fname', 'fname', '', 'chosen') }}
Conclusion:
Twig's inheritance, filters, and functions provide a powerful mechanism for creating extensible and maintainable templates. This approach promotes code reuse, reduces errors, and enhances overall development efficiency. The combination of these features makes Twig a top choice for PHP templating.
Frequently Asked Questions (FAQs):
The provided FAQs section in the original input is already comprehensive and well-structured. No changes are needed.
The above is the detailed content of PHP Master | Extending Twig Templates: Inheritance, Filters, Functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


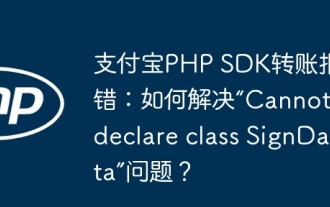
Alipay PHP...
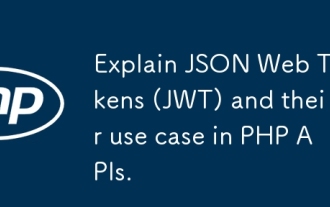
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
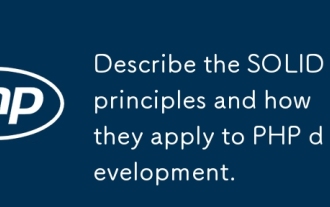
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
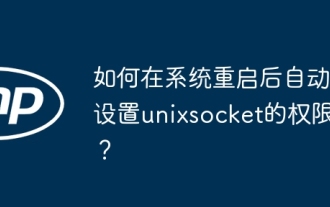
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
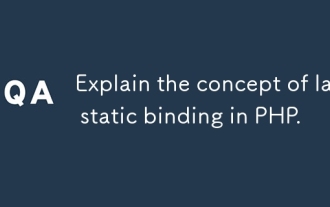
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
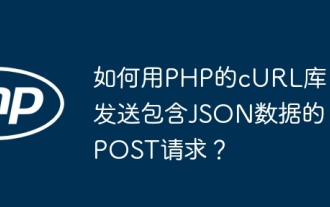
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
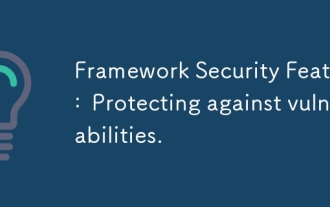
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
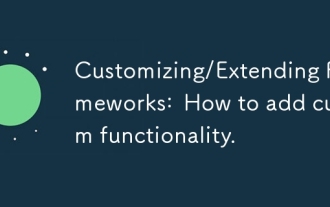
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
