PHP Master | Abstracting Shipping APIs
Core points
- Use abstraction layers to integrate multiple transport APIs (UPS, FedEx, USPS) into your e-commerce platform, providing a unified interface for a variety of transport operations.
- First set up the shipping provider account and obtain the necessary API keys and documentation to ensure compliance with the shipping provider's guidelines and procedures.
- Define and manage goods and parcels through object classes (Shipment and Package) standardized in the code, which simplifies the handling of different transport parameters and requirements.
- Implement the shipper plug-in to interact with a specific shipping API, so that rates and shipping tags are obtained without changing the core application code.
- Train errors calmly and protect abstraction layers to protect sensitive data, ensuring your e-commerce platform provides customers with reliable and secure shipping options.
Your new custom e-commerce store is almost done. The only thing left is figuring out how to calculate the customer's shipping fee. You don't want to use a standard flat rate for each address because you know you'll charge some customers too much and, more importantly, too low fees for others. Wouldn't it be great if shipping costs can be calculated based on the weight/size and destination of the item? Maybe you can even offer an accurate price quote for overnight delivery! You have a UPS account and you have checked their API, but it looks very complicated. If you hardcode your website to use APIs, you will have to do a lot of work if you need to change the shipper. Your cousin is a sales representative for FedEx and he swears he can get better rates for you with FedEx. Some of your customers only use PO boxes, so these items must be shipped via the post office. What should you do? You may have heard of database abstraction, a practice that allows you to use many different databases and a common set of commands. This is exactly what you can do here! To solve all of these problems, you can separate the transport task from the rest of the code and build an abstraction layer. Once done, it doesn't matter whether you ship your package via UPS, FedEx or USPS. The functions your core application will call will be exactly the same, which will make your life much easier!
UPS Getting Started
In this article, I will focus on using the UPS API, but by writing plugins for different shippers (such as FedEx or USPS), you can also access their services, and changes to core application code can be ignored Don't count. In order to get started with UPS, you need to register an online account at www.ups.com using your existing shipper number. Make sure to choose a username and password that you are willing to use for a while, as the API requires both for each call. Next, visit https://www.php.cn/link/ebd74b9b3bfd11deb539e4242d95078b and register to access the UPS API. Here you will get your API key and be able to download documentation for different API packages. (Note: There are known issues with this section of the UPS website, and Chrome sometimes returns blank pages. You may need to use a different browser.) Remember that when you use the UPS API (or any of the shipping APIs), you Agree to abide by their rules and procedures. Be sure to review and obey them, especially before using your code for production environments, follow their instructions. Next, download or clone the shipping abstraction layer package from github.com/alexfraundorf-com/ship on GitHub and upload it to a server running PHP 5.3 or later. Open the include/config.php file. You need to enter your UPS details here, and the field names should be self-explanatory. Please note that the UPS shipper address must match the address recorded in the UPS account, otherwise an error will occur.
Define goods and parcels
Now define a Shipment object. On instantiation, it will accept an array containing the receiver information, and if it is different from the shipper information in our configuration file, you can select a shipping address.
1 2 |
|
Next, we need some details about what we are shipping. Let's create a Package object that accepts optional arrays of weight, package size, and some basic options such as description, whether signature is required, and insurance amount. Then add the newly instantiated Package(s) to the Shipment object. Software that simulates life makes sense: each parcel belongs to a cargo, and each cargo must contain at least one parcel.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
|
(The following content is a simplification and rewriting of the "Behind the Curtain" chapter in the original text, avoiding duplication of redundant information and maintaining the integrity of key information)
Shipment object details: The Awsp/Ship/Shipment.php
object in Shipment
stores the receiver information (and optional shipper information) and includes the addPackage()
and getPackages()
methods to manage the package.
Package object details: The object in Awsp/Ship/Package.php
stores the package weight, size and optional parameters, automatically sorts the dimensions by length, width and height, and calculates the package size (length, perimeter) . Package
Shipman Plugin: Plugin (e.g. ShipUps
) implements the ShipperInterface
interface, providing a unified getRate()
(get freight) and createLabel()
(create tags) method.
Get shipping: Get shipping by calling $ups->getRate()
and use the try/catch
block to handle the error. The result is returned as a RateResponse
object, containing the status and details of each shipping option.
Create a shipping tag: Call $ups->createLabel()
Create a shipping tag, and the result is returned as a LabelResponse
object, containing the status, total cost, tracking number, and base-64-encoded tag image.
Detailed explanation of RateResponse object: The Awsp/Ship/RateResponse.php
object in RateResponse
stores freight data in standardized format, including status, service option arrays, etc.
Detailed explanation of LabelResponse object: The Awsp/Ship/LabelResponse.php
object in LabelResponse
stores label data in standardized format, including status, total cost, label array, etc.
Detailed explanation of UPS Shipmenter Plugin: Awsp/Ship/Ups.php
Convert standardized Package
and Shipment
objects to formats that are understandable by the UPS API, communicate with the SOAP API, and convert the response to Standardized RateResponse
or LabelResponse
objects.
Summary: With the abstraction layer, you can easily use the UPS API or other shipper's API, simplifying interaction with different APIs and reducing maintenance costs. If you need to integrate USPS, it is recommended to use USPS approved vendors such as stamps.com instead of directly using the official USPS API.
(The original FAQs part has been streamlined, retaining core information and avoiding duplication)
FAQs (FAQs)
- What is the purpose of the abstract transportation API? Simplifies the process of integrating various transportation services into a single application, providing a unified interface and reducing complexity.
- How does the abstract transportation API benefit your business? Seamlessly integrate multiple shipping options to improve customer satisfaction, simplify operations, automate processes, and reduce errors.
- What are the challenges of the abstract transportation API? The structural, functional and documentation differences of different APIs require flexible and powerful abstraction layers and require continuous maintenance to cope with API updates.
- How to deal with errors in the abstract transport API? Implement a robust error handling mechanism, verify API responses, catch exceptions, and provide meaningful error messages.
- Can you use a third-party library to abstract the shipping API? Yes, but requires careful evaluation to ensure that specific needs are met and actively maintained.
- How to test abstraction layers? Write unit tests and integration tests and use mock APIs to test.
- How to deal with rate limiting in abstract transport API? Implement mechanisms to handle rate limits, such as retrying a request or reducing the request rate.
- How to protect abstract layers? Implement security measures such as encrypting sensitive data, using secure communication protocols, and periodically updates to fix security vulnerabilities.
- How to deal with version control in the abstract transport API? Design abstraction layers to handle different API versions, such as using version-specific endpoints or parameters.
- How to keep abstraction layers in sync with changes in the shipping API? Regularly monitor shipping API changes, subscribe to API updates, and quickly test and deploy updates.
The above is the detailed content of PHP Master | Abstracting Shipping APIs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
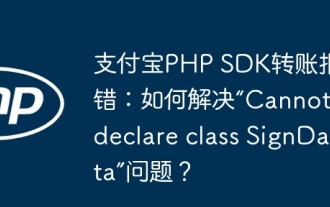
Alipay PHP...
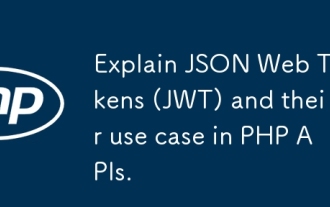
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
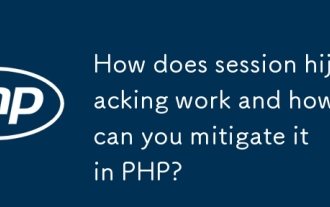
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
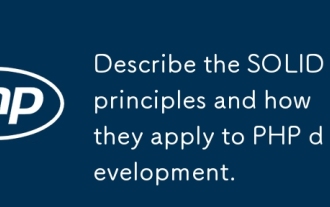
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
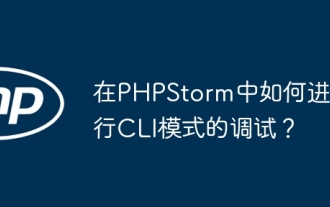
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
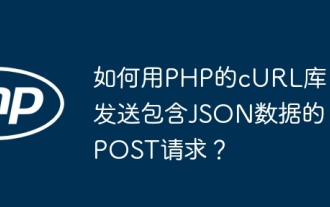
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
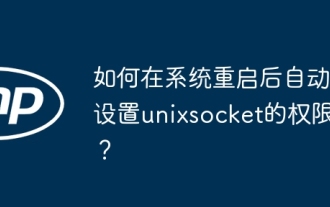
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
