jQuery/HTML5 Input Focus and Cursor Positions
This document provides code snippets and examples demonstrating how to manage cursor input focus and position using jQuery and HTML5. Feedback and suggestions are welcome.
jQuery Input Focus
Use the focus()
function to set focus to an input element:
// Set focus on input $('input[name=firstName]').focus();
See this in action on https://www.php.cn/link/3f74a886c7f841699690962c497d4f30
HTML5 Input Focus
HTML5 offers built-in auto-focus capabilities. While widely supported, note that it may not function consistently across all browsers (tested working in Chrome and Firefox, but not IE9 or older).
<input type="text" autofocus>
See this example on https://www.php.cn/link/8bd045c0275185605e58d7fec40ecae6
jQuery Set Cursor Position
This jQuery function sets the cursor position to a specific character index within an input field:
// Set cursor position $.fn.setCursorPosition = function(pos) { this.each(function(index, elem) { if (elem.setSelectionRange) { elem.setSelectionRange(pos, pos); } else if (elem.createTextRange) { var range = elem.createTextRange(); range.collapse(true); range.moveEnd('character', pos); range.moveStart('character', pos); range.select(); } }); return this; };
Example Usage: Sets the cursor position after the first character.
$('#username').setCursorPosition(1);
See this example on https://www.php.cn/link/5496f40877a2ded20411a2266e86f523
jQuery Select Text Range
This jQuery function automatically selects a specific range of text (a number of characters) within an input field:
// Select text range $.fn.selectRange = function(start, end) { return this.each(function() { if (this.setSelectionRange) { this.focus(); this.setSelectionRange(start, end); } else if (this.createTextRange) { var range = this.createTextRange(); range.collapse(true); range.moveEnd('character', end); range.moveStart('character', start); range.select(); } }); };
Example Usage: Selects the first 5 characters.
$('#username').selectRange(0, 5);
See this example on https://www.php.cn/link/c7410e5d6aa6b2f78ea7d9267b7908c2
Frequently Asked Questions (FAQs)
This section addresses common questions regarding jQuery/HTML5 input focus and cursor positioning. The answers provide concise code examples for clarity. (Note: The original FAQ section had some formatting inconsistencies and code blocks that weren't properly formatted as code. This version corrects those issues.)
Q: How can I set the cursor position at the end of the input field using jQuery?
var input = $('#inputField'); var len = input.val().length; input.focus(); input[0].setSelectionRange(len, len);
Q: How can I use jQuery to focus on an input field when a link is clicked?
$('#linkID').click(function() { $('#inputField').focus(); });
Q: What is the difference between the focus
and focusin
events in jQuery?
focus
is triggered when an element receives focus and doesn't bubble. focusin
is similar but bubbles up the DOM.
Q: How can I trigger the focus event manually in jQuery?
$('#inputField').focus(); // or $('#inputField').trigger('focus');
Q: How can I detect when an input field loses focus in jQuery?
// Set focus on input $('input[name=firstName]').focus();
Q: How can I prevent an input field from losing focus in jQuery?
Using preventDefault
within a focusout
handler is generally not recommended as it can lead to unexpected behavior. Consider alternative approaches to achieve your desired outcome.
Q: How can I set the focus on the next input field when the enter key is pressed in jQuery?
<input type="text" autofocus>
Q: How can I set the cursor position at a specific index in an input field using jQuery?
// Set cursor position $.fn.setCursorPosition = function(pos) { this.each(function(index, elem) { if (elem.setSelectionRange) { elem.setSelectionRange(pos, pos); } else if (elem.createTextRange) { var range = elem.createTextRange(); range.collapse(true); range.moveEnd('character', pos); range.moveStart('character', pos); range.select(); } }); return this; };
Q: How can I get the current cursor position in an input field using jQuery?
$('#username').setCursorPosition(1);
Q: How can I move the cursor to the start of an input field using jQuery?
// Select text range $.fn.selectRange = function(start, end) { return this.each(function() { if (this.setSelectionRange) { this.focus(); this.setSelectionRange(start, end); } else if (this.createTextRange) { var range = this.createTextRange(); range.collapse(true); range.moveEnd('character', end); range.moveStart('character', start); range.select(); } }); };
The above is the detailed content of jQuery/HTML5 Input Focus and Cursor Positions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


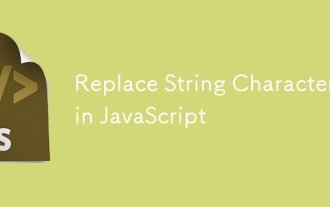
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
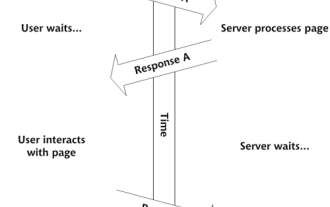
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
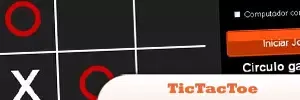
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
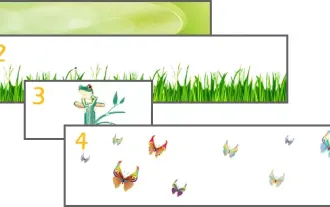
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
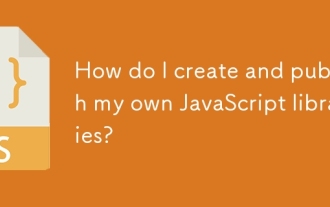
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
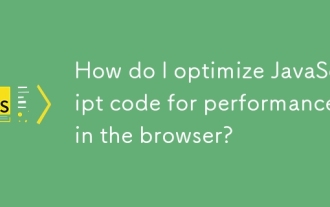
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
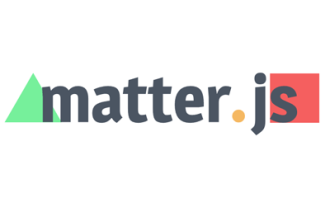
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
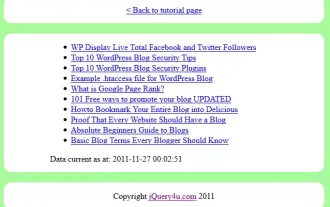
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
