PHP Master | Error Condition Testing with PHPUnit
Core points
- PHPUnit converts PHP native error handling into exceptions, which may change the flow of code execution during testing. This can cause problems when testing code using
trigger_error()
functions. - PHPUnit converts errors to exceptions, which causes the code to behave differently in development and testing than in production environments. This is because the execution process changes when an error is encountered.
- To accurately test the code using
trigger_error()
, you can use a custom error handler to capture error information for later analysis using assertions. This allows the code to continue execution while still allowing checking for the error condition raised. - Unit's default behavior is critical for accurate testing. In the event of a post-triggering logic, supplementing PHPUnit's functionality can facilitate accurate testing as close to the production environment as possible.
Suppose you are maintaining code that records error messages using PHP native trigger_error()
functions. Meanwhile, you are writing unit tests for that code using PHPUnit. If you refer to the PHPUnit manual, there is a section dedicated to how to test error conditions. It describes how PHPUnit implements its own error handler that converts errors, warnings, and notifications into exceptions, and catching these exceptions is how you should handle such error tests. However, depending on the appearance of your code, you may have problems with this approach with PHPUnit. This article will explain in detail what this issue is, how it affects your ability to test your code, and how to solve it.
What is the problem?
Errors and exceptions behave in a fundamentally different way. In particular, related to this article, if the error level constant passed to it does not indicate a fatal error, code execution can continue immediately after trigger_error()
. When an exception is thrown, execution will continue at the beginning of the catch
block corresponding to that exception class, which may or may not occur immediately after the point where the exception is thrown. Let's look at some examples of these behaviors. First of all, it's an error.
<?php error_reporting(E_ALL | E_STRICT); echo "Before warning\n"; trigger_error("Danger Will Robinson!", E_USER_WARNING); echo "After warning\n"; ?>
If you run the above code, you will get the following output:
<code>Before warning PHP Warning: Danger Will Robinson! in /home/matt/error_handler.php on line 4 After warning</code>
From this we can see that the trigger_error()
statement after the echo
is executed. Now, exception.
<?php try { echo "Before exception\n"; throw new Exception("Danger Will Robinson!"); echo "After exception\n"; } catch (Exception $e) { echo "In catch block\n"; } ?>
Output:
<code>Before exception In catch block</code>
In contrast to the error example, the code after the exception is thrown is not executed. Because PHPUnit converts an error to an exception, the error behaves the same as the exception in unit tests. During testing, any code that is executed after an error is triggered will not be executed. Give another example:
<?php function foo($param) { if (is_string($param)) { trigger_error(__FUNCTION__ . " no longer supports strings, pass an array", E_USER_NOTICE); } // do useful stuff with $param ... } ?>
With the error-to-exception conversion, it is impossible to test whether $param
is handled usefully because when the error is converted to an exception, the code will never be executed.
Side effects of PHPUnit behavior
This error-to-exception conversion will cause the code to behave differently in development and testing than in production environments. Here is an example:
<?php error_reporting(E_ALL | E_STRICT); echo "Before warning\n"; trigger_error("Danger Will Robinson!", E_USER_WARNING); echo "After warning\n"; ?>
Output:
<code>Before warning PHP Warning: Danger Will Robinson! in /home/matt/error_handler.php on line 4 After warning</code>
The first var_dump()
call (during this time, a custom error handler that converts the error to an exception is taking effect) outputs NULL. The second var_dump()
call (during which PHP's default error handler is taking effect) outputs information about the triggered error. Note that this is not because the first var_dump()
call output NULL is caused by the use of a custom error handler, but because the error handler throws an exception. If the error handler shown in this example does not do this, the output of the first var_dump()
call will be the same as the second.
Solution
We need a solution that allows continuing to execute the code being tested while still allowing us to check if an error condition was raised. As shown in the above example, allowing code execution to continue can be done using a custom error handler that does not convert errors to exceptions. What this error handler should do is capture the error message so that it can be analyzed later using assertions. This is what it looks like:
<?php try { echo "Before exception\n"; throw new Exception("Danger Will Robinson!"); echo "After exception\n"; } catch (Exception $e) { echo "In catch block\n"; } ?>
setUp()
(run before each test method) handles setting up an error handler, which is just another method in the same class that stores information about each error in an array. Other methods (such as assertError()
) are then used by test methods (such as testDoStuff()
) to perform assertions on this error message and output relevant debug information, such as what is the error triggered compared to the expected error. Other useful assertion types include logical inversion (i.e., assertions do not trigger a specific error), errors that check messages match a regular expression, or number of errors that are triggered.
Conclusion
If you don't care if the logic behind the test triggering an error is still executing, the default behavior of PHPUnit is perfectly suitable for your needs. However, it is important that you understand what the behavior means. If you do care about the execution of such logic, it is also important that you know how to complement PHPUnit's capabilities in order to facilitate accurate testing of your code as close to the production environment as possible.
Pictures from Fotolia
(The following is FAQ, the format and expression have been adjusted according to the original content, and some issues have been merged or simplified)
FAQs (FAQ) on using PHPUnit to test error conditions
Q1: Why is PHPUnit not showing any errors in the console?
PHPUnit is designed in a way that allows for effective testing of errors and exceptions. If you don't see any errors in the console, PHPUnit may be capturing them and treating them as failed tests. To view the details of these errors, you can use the --debug
option when running the test. This will provide more detailed output, including any errors or exceptions caught during testing.
Q2: How to assert that an exception was thrown in PHPUnit?
PHPUnit provides a set of assertions specifically used to handle exceptions. The most commonly used is expectException()
, which you can use to specify the type of exception you expect to throw. If the specified exception is thrown during the test, the test will pass. If not, the test will fail. This allows you to write tests that specifically check for correct handling of error conditions.
Q3: How does error reporting work in PHP?
PHP's Error Reporting feature allows you to control which errors are reported and how to deal with them. By default, all errors are reported and displayed. However, you can change these settings using the error_reporting()
function and the display_errors
ini directive. This allows you to hide certain types of errors, or log errors instead of displaying them.
Q4: How to test exceptions in PHPUnit?
Same as Q2.
Q5: How to write tests for PHPUnit?
Writing tests for PHPUnit involves creating a new test case class that extends the PHPUnitFrameworkTestCase class. Each test is a public method in this class, starting with the word "test". Within each test method, you can use PHPUnit's assertion to check if your code is running as expected. For example, you can use the assertEquals()
method to check whether the function returns the expected result.
Q6: How to handle errors in PHPUnit?
PHPUnit provides a set of assertions specifically used to handle errors. The most commonly used is expectError()
, which you can use to specify the type of error you expect to trigger. If the specified error is triggered during the test, the test passes. If not, the test will fail. This allows you to write tests that specifically check for correct handling of error conditions.
Q7: How to debug tests in PHPUnit?
PHPUnit provides several options for debugging tests. The --debug
option provides more detailed output, including any errors or exceptions caught during testing. The --stop-on-error
, --stop-on-failure
and --stop-on-risky
options can be used to stop the test running when an error of some type is encountered. This can make it easier to identify and fix problems.
Q8: How to test error conditions in PHPUnit?
PHPUnit provides several ways to test error conditions. The expectError()
method allows you to specify the type of error you expect to trigger. The expectWarning()
method allows you to specify the type of warning you expect to trigger. If the specified error or warning is triggered during the test, the test will pass. If not, the test will fail.
Q9: How to handle warnings in PHPUnit?
PHPUnit provides a set of assertions specifically used to handle warnings. The most commonly used is expectWarning()
, which you can use to specify the type of warning you expect to trigger. If the specified warning is triggered during the test, the test passes. If not, the test will fail. This allows you to write tests that specifically check the correct handling of warning conditions.
Q10: How to use data providers in PHPUnit?
Data provider is a powerful feature of PHPUnit that allows you to run tests multiple times with different datasets. To use the data provider, you can create a method that returns an array of arrays. Each internal array is a set of parameters for the test. You then comment your test method using @dataProvider
followed by the name of your data provider method. PHPUnit will then run the test once for each set of parameters and pass the parameters to the test method.
The above is the detailed content of PHP Master | Error Condition Testing with PHPUnit. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
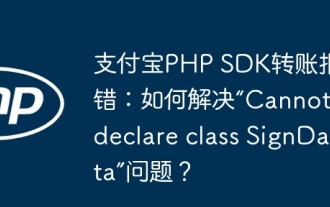
Alipay PHP...
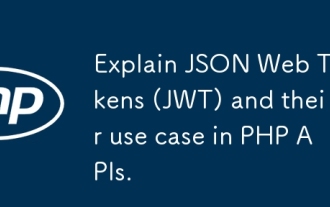
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
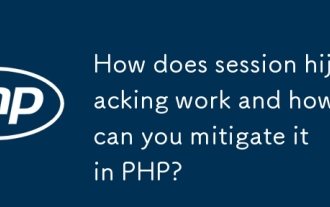
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
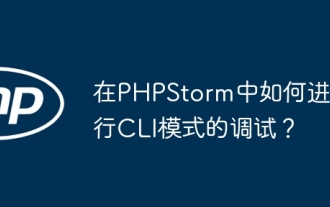
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
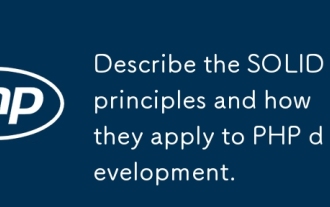
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
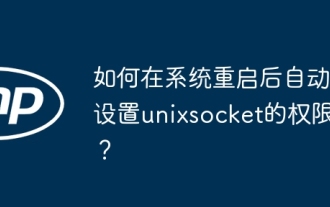
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
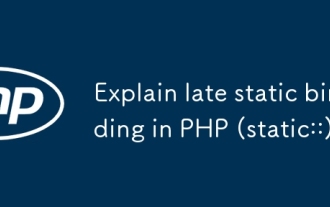
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
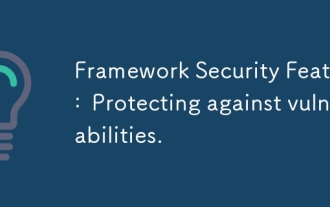
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
