How to Capture CSS3 Animation Events in JavaScript
Key Points
- JavaScript can manage CSS3 animations by adding event handlers to elements, enabling fine control of animations, such as playing them in sequence. There are three types of events that can be captured: "animationstart", "animationitration", and "animationend".
- Cross-browser compatibility of these animation events can be achieved by calling
addEventListener
for all prefixed and unprefixed names using custom functions. This allows the event handler to be assigned using a line of code. - The event object passed to the animation listener function provides the animation name and elapsed time since the start of the animation. This can be used to detect when a particular animation ends and execute other code, such as applying another CSS3 animation in a specific order.
CSS3 animations are smooth and easy to implement, but unlike JavaScript, it does not have frame-by-frame control. Fortunately, you can apply event handlers to any element to determine the animation state. This allows for fine control, such as playing different animations in sequence.
Consider this simple CSS3 animation:
#anim.enable { -webkit-animation: flash 1s ease 3; -moz-animation: flash 1s ease 3; -ms-animation: flash 1s ease 3; -o-animation: flash 1s ease 3; animation: flash 1s ease 3; } /* 动画 */ @-webkit-keyframes flash { 50% { opacity: 0; } } @-moz-keyframes flash { 50% { opacity: 0; } } @-ms-keyframes flash { 50% { opacity: 0; } } @-o-keyframes flash { 50% { opacity: 0; } } @keyframes flash { 50% { opacity: 0; } }
When the enable
class is applied to an element with ID anim
, an animation named flash
will run three times. Each iteration lasts for a second, during which the element fades out and then fades in.
Three types of events will be triggered when the animation occurs:
animationstart
var anim = document.getElementById("anim"); anim.addEventListener("animationstart", AnimationListener, false);
animationstart
Event is fired when the animation is first started.
animationiteration
anim.addEventListener("animationiteration", AnimationListener, false);
animationiteration
Events are fired at the beginning of each new animation iteration, i.e. each iteration except the first iteration.
animationend
anim.addEventListener("animationend", AnimationListener, false);
animationend
Event fires at the end of the animation.
Browser compatibility
At the time of writing, Firefox, Chrome, Safari, Opera, and IE10 support CSS3 animations and related event handlers. Additionally, Opera, IE10, and webkit browsers use prefixes and have made some case changes to ensure foolproof...
W3C Standard Firefox webkit Opera IE10
animationstart
animationstart
webkitAnimationStart
oanimationstart
MSAnimationStart
animationiteration
animationiteration
webkitAnimationIteration
oanimationiteration
MSAnimationIteration
animationend
animationend
webkitAnimationEnd
oanimationend
MSAnimationEnd
addEventListener
var pfx = ["webkit", "moz", "MS", "o", ""]; function PrefixedEvent(element, type, callback) { for (var p = 0; p < pfx.length; p++) { if (!pfx[p]) type = type.toLowerCase(); element.addEventListener(pfx[p] + type, callback, false); } }
#anim.enable { -webkit-animation: flash 1s ease 3; -moz-animation: flash 1s ease 3; -ms-animation: flash 1s ease 3; -o-animation: flash 1s ease 3; animation: flash 1s ease 3; } /* 动画 */ @-webkit-keyframes flash { 50% { opacity: 0; } } @-moz-keyframes flash { 50% { opacity: 0; } } @-ms-keyframes flash { 50% { opacity: 0; } } @-o-keyframes flash { 50% { opacity: 0; } } @keyframes flash { 50% { opacity: 0; } }
Event object
In the above code, the AnimationListener
function is called whenever an animation event occurs. An event object is passed as a single parameter. In addition to standard properties and methods, it also provides:
animationName
: CSS3 animation name (i.e.flash
)elapsedTime
: Time (in seconds) since the start of the animation.
Therefore, we can detect when the flash
animation ends, for example:
var anim = document.getElementById("anim"); anim.addEventListener("animationstart", AnimationListener, false);
For example, the code can remove an existing class or apply another CSS3 animation in a specific order.
View CSS3 animation event demonstration in JavaScript
The demo page displays a button. When you click it, the "enable" class is toggled, thus starting the flash
animation. When the animation event is triggered, the status is displayed in the console. After the animation is over, the "enable" class will be removed so that the button can be clicked again.
If you use animated event capture in any interesting project, please let me know.
(If you enjoy reading this article, you will love Learnable; this is where to learn new skills and skills from masters. Members can instantly access all SitePoint's e-books and interactive online courses, such as " HTML5 and CSS3 for the real world)
(The comments for this article are closed. Are there any questions about CSS? Why not ask on our forum?)
FAQs (FAQ) about CSS3 animations and JavaScript event handlers
What are CSS3 animations and JavaScript event handlers?
CSS3 animation is a feature of CSS3 (cascading stylesheets) that allows you to animate HTML elements without using JavaScript or Flash. It provides a way to create simple animations directly in CSS code without any additional resources. On the other hand, JavaScript event handlers are functions in JavaScript that trigger when specific events occur. These events can be any event such as a user clicking a button, page loading, or ending an animation. Use CSS3 animations and JavaScript event handlers to create dynamic interactive web content.
How to use JavaScript event handler with CSS3 animations?
To use the JavaScript event handler with CSS3 animations, you need to add an event listener to the HTML element you want to animate. This event listener will trigger the JavaScript function at the end of the animation. Here is a basic example:
anim.addEventListener("animationiteration", AnimationListener, false);
In this code, "animationend" is the event we are listening on, which fires when the CSS3 animation is complete.
Can I use JavaScript event handlers with CSS3 transitions?
Yes, you can use JavaScript event handlers with CSS3 transitions just like you do with CSS3 animations. The event you need to listen for is "transitionend". This event is fired after the CSS transition is complete.
How to use JavaScript to control the time of CSS3 animations?
You can use JavaScript to control the time of CSS3 animation using the "animation-delay" property. This property sets the delay in the start of the animation. You can set this property in JavaScript like this:
#anim.enable { -webkit-animation: flash 1s ease 3; -moz-animation: flash 1s ease 3; -ms-animation: flash 1s ease 3; -o-animation: flash 1s ease 3; animation: flash 1s ease 3; } /* 动画 */ @-webkit-keyframes flash { 50% { opacity: 0; } } @-moz-keyframes flash { 50% { opacity: 0; } } @-ms-keyframes flash { 50% { opacity: 0; } } @-o-keyframes flash { 50% { opacity: 0; } } @keyframes flash { 50% { opacity: 0; } }
In this code, the animation will start after the page is loaded for 2 seconds.
How to repeat CSS3 animations using JavaScript?
You can repeat CSS3 animations using JavaScript using the "animation-iteration-count" property. This property specifies the number of times the animation should be played. You can set this property in JavaScript like this:
var anim = document.getElementById("anim"); anim.addEventListener("animationstart", AnimationListener, false);
In this code, the animation will be repeated indefinitely.
Can I change the speed of CSS3 animations using JavaScript?
Yes, you can change the speed of CSS3 animations using JavaScript. The "animation-duration" property controls the length of time it takes for the animation to complete a cycle. You can set this property in JavaScript like this:
anim.addEventListener("animationiteration", AnimationListener, false);
In this code, the animation will last for 2 seconds.
How to pause and restore CSS3 animations using JavaScript?
You can pause and restore CSS3 animations using JavaScript using the "animation-play-state" property. This property sets whether the animation is running or paused. You can set this property in JavaScript like this:
anim.addEventListener("animationend", AnimationListener, false);
In this code, the animation will be paused.
Can I change the orientation of CSS3 animations using JavaScript?
Yes, you can change the orientation of your CSS3 animation using JavaScript. The "animation-direction" property defines whether the animation should be played in reverse during an alternating cycle. You can set this property in JavaScript like this:
var pfx = ["webkit", "moz", "MS", "o", ""]; function PrefixedEvent(element, type, callback) { for (var p = 0; p < pfx.length; p++) { if (!pfx[p]) type = type.toLowerCase(); element.addEventListener(pfx[p] + type, callback, false); } }
In this code, the animation will play backwards.
How to use JavaScript to detect the end of a CSS3 animation?
You can use JavaScript to detect the end of a CSS3 animation by adding an event listener to the "animationend" event. This event is fired after the CSS3 animation is completed. Here's how to do it:
// 动画侦听器事件 PrefixedEvent(anim, "AnimationStart", AnimationListener); PrefixedEvent(anim, "AnimationIteration", AnimationListener); PrefixedEvent(anim, "AnimationEnd", AnimationListener);
Can I change the animation-timing-function of CSS3 animation using JavaScript?
Yes, you can change the "animation-timing-function" of CSS3 animations using JavaScript. This property specifies the velocity curve of the animation. You can set this property in JavaScript like this:
if (e.animationName == "flash" && e.type.toLowerCase().indexOf("animationend") >= 0) { ... }
In this code, the animation will start slowly and then accelerate.
The above is the detailed content of How to Capture CSS3 Animation Events in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




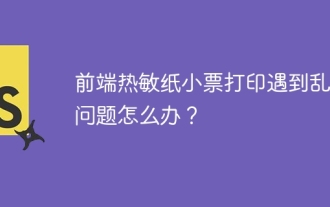
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
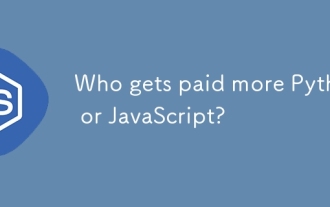
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
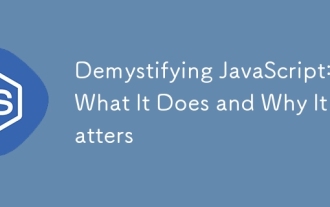
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
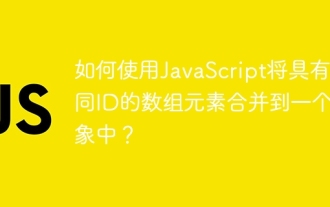
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
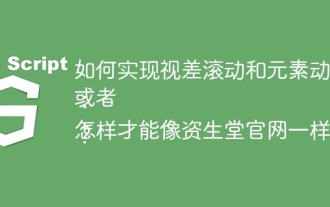
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
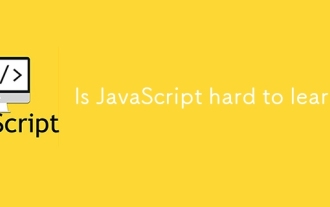
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
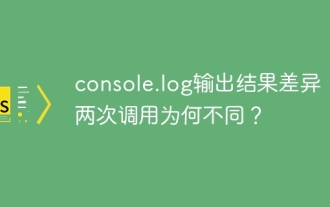
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
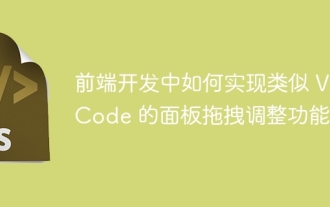
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
