How to Asynchronously Upload Files Using HTML5 and Ajax
Core points
- Uploading files asynchronously using HTML5 and Ajax can be done in the background, allowing users to perform other tasks on the page during uploading. This process involves creating HTML forms to upload files to PHP pages, and JavaScript ensures that only JPG images with less than 300,000 bytes are uploaded.
- The JavaScript upload function requires an XMLHttpRequest2 object (available in Firefox and Chrome). The .open() method of XMLHttpRequest is set to POST the data to the PHP page, set the HTTP header to the name of the file, and pass the File object to the .send() method.
- PHP file checks the X_FILENAME HTTP header to distinguish between Ajax requests and standard form POST. If the file name is set, PHP will retrieve the published data and output it to a new file in the "uploads" folder. Standard HTML multipart/form-data POST is handled using the usual PHP $_FILE function.
In previous articles, we learned how to use HTML5 to drag and drop files and how to open files using HTML5 and JavaScript. Now we have a valid set of files that can upload each file to the server. This process takes place asynchronously in the background, so users can complete other tasks on the page as the process progresses.
HTML
Let's check our HTML form again:
<fieldset><legend>HTML文件上传</legend><div> <label for="fileselect">要上传的文件:</label> <div>或在此处拖放文件</div></div><div>上传文件</div></fieldset>
We upload the file to the PHP page upload.php. When the user clicks "Upload File", the page will process Ajax upload requests and standard form POST. Our JavaScript will ensure that only JPG images are uploaded with less than 300,000 bytes (the value specified in MAX_FILE_SIZE).
JavaScript
First, we need to add a line to the FileSelectHandler() function called when selecting or dragging and dropping one or more files. In our File loop, we will call another function - UploadFile():
// 文件选择 function FileSelectHandler(e) { // 取消事件和悬停样式 FileDragHover(e); // 获取FileList对象 var files = e.target.files || e.dataTransfer.files; // 处理所有File对象 for (var i = 0, f; f = files[i]; i++) { ParseFile(f); UploadFile(f); } }
File upload requires an XMLHttpRequest2 object, which is currently available in Firefox and Chrome. Before making the Ajax call, we make sure that the .upload() method is available and we have a JPG with a file size smaller than the MAX_FILE_SIZE form value:
// 上传JPEG文件 function UploadFile(file) { var xhr = new XMLHttpRequest(); if (xhr.upload && file.type == "image/jpeg" && file.size < $id("MAX_FILE_SIZE").value) { // 开始上传 xhr.open("POST", $id("upload").action, true); xhr.setRequestHeader("X_FILENAME", file.name); xhr.send(file); } }
The .open() method of XMLHttpRequest is set to POST the data to upload.php (the action property of our upload form). Additionally, we set the HTTP header to the name of the file and pass the File object to the .send() method.
PHP
Our PHP file upload.php now checks the X_FILENAME HTTP header to distinguish between Ajax requests and standard form POST:
<?php $fn = (isset($_SERVER['HTTP_X_FILENAME']) ? $_SERVER['HTTP_X_FILENAME'] : false); ?>
If the file name is set, PHP can retrieve published data and output it to a new file in the "uploads" folder. Surprisingly, this can be achieved with a single line of code:
if ($fn) { // AJAX调用 file_put_contents( 'uploads/' . $fn, file_get_contents('php://input') ); echo "$fn 上传成功"; exit(); }
The standard HTML multipart/form-data POST can be handled using the usual PHP $_FILE function:
<fieldset><legend>HTML文件上传</legend><div> <label for="fileselect">要上传的文件:</label> <div>或在此处拖放文件</div></div><div>上传文件</div></fieldset>
You can view the demo page, but note that it is hosted on servers without PHP support and uploads do not happen. So download the file to check the code and install it on your own PHP server.
The above code works, but the user does not know if the file upload has started, completed or failed. You need to read the last issue of this series: How to create a file upload progress bar in HTML5 and JavaScript…
FAQ (FAQ) About HTML5 AJAX File Upload
(The FAQ part is omitted here because this part has nothing to do with pseudo-original goals and is longer. It is OK to retain FAQ, but the language needs to be reorganized to make it consistent with the overall style of the article.)
The above is the detailed content of How to Asynchronously Upload Files Using HTML5 and Ajax. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


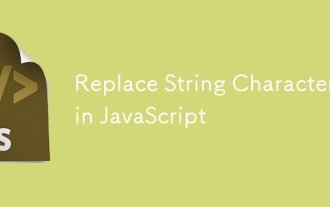
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
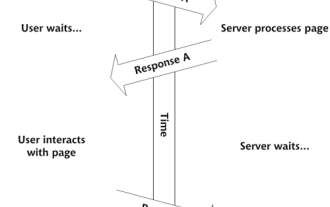
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
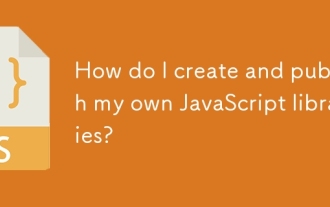
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
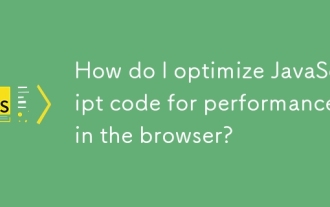
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
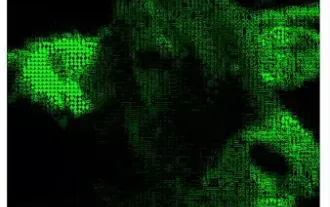
Bring matrix movie effects to your page! This is a cool jQuery plugin based on the famous movie "The Matrix". The plugin simulates the classic green character effects in the movie, and just select a picture and the plugin will convert it into a matrix-style picture filled with numeric characters. Come and try it, it's very interesting! How it works The plugin loads the image onto the canvas and reads the pixel and color values: data = ctx.getImageData(x, y, settings.grainSize, settings.grainSize).data The plugin cleverly reads the rectangular area of the picture and uses jQuery to calculate the average color of each area. Then, use
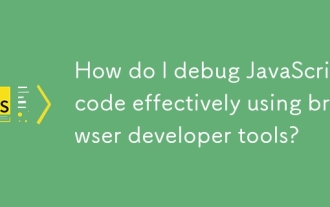
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
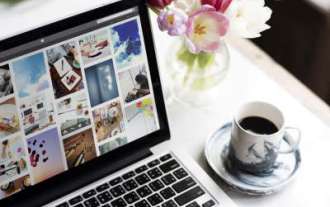
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
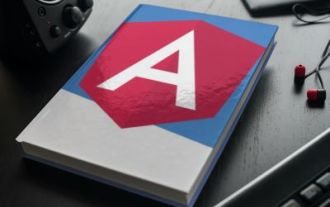
Data sets are extremely essential in building API models and various business processes. This is why importing and exporting CSV is an often-needed functionality.In this tutorial, you will learn how to download and import a CSV file within an Angular
