PHP Master | 5 Inspiring (and Useful) PHP Snippets
The Internet is full of various articles of "X PHP code snippets" type, why do you need to write another article? The reason is simple: most of the code snippets in the article are lackluster. Generating random strings or returning $_SERVER["REMOTE_ADDR"]
to get fragments like client IP addresses is really not fun and practical. This article will share five practical and interesting snippets of PHP code and introduce the inspiration behind them. Hopefully these creative code snippets will inspire you to write better and more creative code in your daily programming.
Key Points
- This article introduces five practical PHP code snippets, including using the built-in
fputcsv()
function to generate CSV data, using the PSR-0 standard to automatically load the class, using theunpack()
function to parse fixed-length data, and using a simple PHP class Do HTML templates and usefile_get_contents
as an alternative to cURL to issue HTTP GET and POST requests. - The author emphasizes the importance of using PHP built-in functions and creating custom solutions to perform common tasks such as generating CSV data or automatically loading classes, which can improve coding efficiency and creativity.
- The author also discussed the benefits and potential risks of using PHP code snippets, suggesting that while they can greatly increase productivity and improve code readability, they should be used with caution, tested in a safe environment, and from trusted Sources to avoid potential problems or security risks.
1. Generate CSV
We often see code like this, trying to convert multidimensional array data to CSV:
<?php $csv = ""; foreach ($data as $row) { $csv .= join(",", $row) . "\n"; } echo $csv; ?>
The problem is that each element is not escaped correctly, and the inclusion of quotes or commas in a single value can cause an error in subsequent parsing of CSV data. It is best to use the built-in fputcsv()
function; its implementation is written in C code, so it executes faster and will handle the necessary references/escapings for you. The following code encapsulates the logic of constructing CSV output from a data array. It contains optional parameters that allow the use of the title column, and whether to refresh the CSV directly to the browser or return the output as a string. The clever thing about it is to use the stream with fputcsv()
, because the function requires an open file handle to operate.
<?php function toCSV(array $data, array $colHeaders = array(), $asString = false) { $stream = ($asString) ? fopen("php://temp/maxmemory", "w+") : fopen("php://output", "w"); if (!empty($colHeaders)) { fputcsv($stream, $colHeaders); } foreach ($data as $record) { fputcsv($stream, $record); } if ($asString) { rewind($stream); $returnVal = stream_get_contents($stream); fclose($stream); return $returnVal; } else { fclose($stream); } } ?>
With the toCSV()
function, generating CSV becomes simple and reliable.
2. Automatically load class
Autoload class files is common, but you may not like those bloated, heavyweight autoloaders that various PHP frameworks offer, or you may just like to write your own solutions. Fortunately, you can write your own minimal loader and still comply with the PSR-0 standard adopted by the PHP Standards Working Group, which I first demonstrated on my own blog. This standard does not describe the support functions (registration method, configuration options, etc.) that PSR-0 compatible autoloaders must provide. If it can automatically find the class definition in <vendor name>(<namespace>)
mode, then it is PSR-0 compatible. Also, it does not specify the parent directory of <vendor name>
. The extra "fill" implemented by most autoloaders is convenient if you need to specify the location through code, but it is not necessary if you are just using directories already in PHP's include path.
<?php $csv = ""; foreach ($data as $row) { $csv .= join(",", $row) . "\n"; } echo $csv; ?>
, which is the namespace name, which may be an empty string. These parts must be identified because underscores do not have any special meaning in the namespace parts, so blind replacement of underscores and backslashes is incorrect. $match[2]
$match[1]
In today's modern world filled with XML and JSON, you might think that fixed-length formats are extinct...but you're wrong. There are still a large amount of fixed-length data, such as certain log entries, MARC 21 (bibliography information), NACHA (financial information), etc. To be honest, I still have a special liking for fixed-length data.
In languages such as C, fixed-length data is relatively easy to process because once loaded into memory, it will be perfectly aligned with the access data structure. But for some people, processing fixed-length data in dynamic languages such as PHP can be a struggle; the loose type of the language makes this memory access impossible. Therefore, we often see code like this:
You may feel uncomfortable. That's OK, I don't want to use code like this in my application either! It's lengthy and indexes are prone to errors. Fortunately, there is a better alternative:
<?php function toCSV(array $data, array $colHeaders = array(), $asString = false) { $stream = ($asString) ? fopen("php://temp/maxmemory", "w+") : fopen("php://output", "w"); if (!empty($colHeaders)) { fputcsv($stream, $colHeaders); } foreach ($data as $record) { fputcsv($stream, $record); } if ($asString) { rewind($stream); $returnVal = stream_get_contents($stream); fclose($stream); return $returnVal; } else { fclose($stream); } } ?>
unpack()
The documentation in the
unpack()
Using
unpack()
<?php spl_autoload_register(function ($classname) { $classname = ltrim($classname, "\"); preg_match('/^(.+)?([^\]+)$/U', $classname, $match); $classname = str_replace("\", "/", $match[1]) . str_replace(["\", "_"], "/", $match[2]) . ".php"; include_once $classname; }); ?>
. A6date
$header["date"]
In the PHP community, there has not been much consensus on templateization. We all agree that it is desirable to separate HTML and PHP, but there is a conflict over the applicability of using template libraries such as Smarty or Twig. Some people point out that PHP itself is a template engine and opposes the speed, syntax, etc. of the library. Others claim to benefit greatly from using DSL provided by the template system. One tradeoff is to template your HTML with very small classes written in PHP to keep your code concise. It is not a mature template engine; it is a neat auxiliary class that acts as a "bucket" that collects key/value data pairs that you can access in the include files specified as templates. First, you create an instance of the example's In the template file, you can access the full range of PHP functions to format values, filter values, etc. as needed. 5. Use file_get_contents as an alternative to cURL cURL is a powerful library for communicating over various protocols. It's really very powerful and sometimes there's no other way to do it. If you explicitly need the functionality exposed by cURL to accomplish your tasks, then use cURL! However, most of the daily cURL usage in PHP revolves around making HTTP GET and POST requests, which can be easily done using PHP built-in functions. There are two issues with relying on cURL to issue HTTP requests: 1) There are often many options to set up even for the simplest transactions, and 2) It is an extension that depending on your hosting and installation, it may also be available. May not be available; it is a common extension, but not enabled by default. For basic GET requests, you can use For requests that require specifying HTTP headers (whether it's GET or any other HTTP method), you can create a context by passing a special keyed array to The above example shows the letters required for uploading files via POST, context arrays use the keys "method", "header", and "content" to specify the transaction 口. When using Summary I hope you find the code snippet introduced in this article interesting. They showcase creative problem solving and using PHP's built-in capabilities for new effects. I hope you find them useful and inspiring. If you have your own inspiring code snippet, feel free to share it in the comments below. (Picture from Fotolia) (FAQ about PHP code snippets) (The FAQ part is omitted here because the content of the original FAQ part has little to do with the code snippet itself, and is a supplementary description. You can add or modify it yourself according to actual needs.) The above is the detailed content of PHP Master | 5 Inspiring (and Useful) PHP Snippets. For more information, please follow other related articles on the PHP Chinese website!<?php
$csv = "";
foreach ($data as $row) {
$csv .= join(",", $row) . "\n";
}
echo $csv;
?>
Template
class in the view, and you can choose to pass a directory name to find subsequent template files (allowing you to group relevant files). Then, the value that should be filled with the template is provided to the set()
method or as a naked attribute. Once all values are specified, you can call the out()
method to render the template. <?php
function toCSV(array $data, array $colHeaders = array(), $asString = false) {
$stream = ($asString)
? fopen("php://temp/maxmemory", "w+")
: fopen("php://output", "w");
if (!empty($colHeaders)) {
fputcsv($stream, $colHeaders);
}
foreach ($data as $record) {
fputcsv($stream, $record);
}
if ($asString) {
rewind($stream);
$returnVal = stream_get_contents($stream);
fclose($stream);
return $returnVal;
} else {
fclose($stream);
}
}
?>
mytemplate.php
file might look like this: <?php
spl_autoload_register(function ($classname) {
$classname = ltrim($classname, "\");
preg_match('/^(.+)?([^\]+)$/U', $classname, $match);
$classname = str_replace("\", "/", $match[1])
. str_replace(["\", "_"], "/", $match[2])
. ".php";
include_once $classname;
});
?>
out()
can specify that the template content is returned as a string instead of refreshing it directly to the browser, you can use it to replace the result of a previously filled template. Placeholder. file_get_contents()
and stream_context_create()
are two native PHP functions that have been available since version 4.3. In conjunction, they can perform many requests of the same type as the cURL usually performs. file_get_contents()
itself: <?php
// 解析NACHA报头记录
$row = fread($fp, 94);
$header = array();
$header["type"] = substr($row, 0, 1);
$header["priority"] = substr($row, 1, 2);
$header["immDest"] = substr($row, 3, 10);
$header["immOrigin"] = substr($row, 13, 10);
$header["date"] = substr($row, 23, 6);
$header["time"] = substr($row, 29, 4);
$header["sequence"] = substr($row, 33, 1);
$header["size"] = substr($row, 34, 3);
$header["blockFactor"] = substr($row, 37, 2);
$header["format"] = substr($row, 39, 1);
$header["destName"] = substr($row, 40, 23);
$header["originName"] = substr($row, 63, 23);
$header["reference"] = substr($row, 86, 8);
print_r($header);
?>
stream_context_create()
and then pass the context to file_get_contents()
. <?php
// 解析NACHA报头记录
$row = fread($fp, 94);
$header = unpack("A1type/A2priority/A10immDest/A10immOrigin/"
. "A6date/A4time/A1sequence/A3size/A2blockFactor/A1format/"
. "A23destName/A23originName/A8reference", $row);
print_r($header);
?>
file_get_contents()
for complex requests (such as file uploads), first create a mock web form and run it through Firefox or similar tools with firebug enabled, then check what is included in the request may be a bit help. From there you can infer the important header elements to include.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


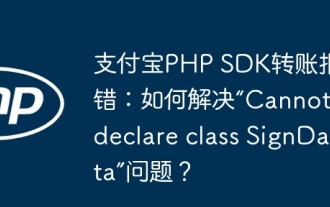
Alipay PHP...
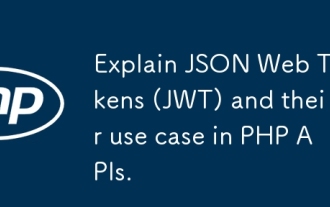
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
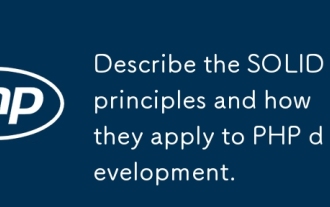
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
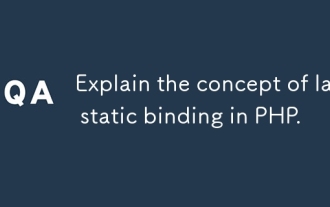
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
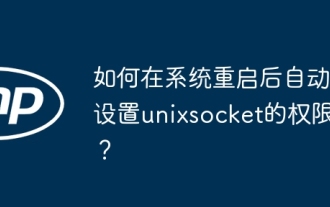
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
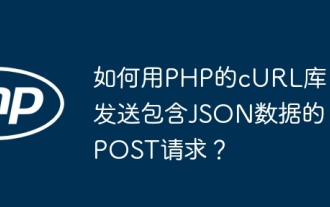
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
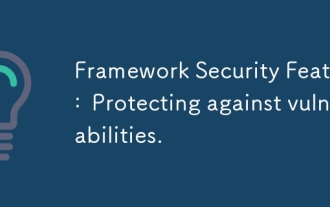
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
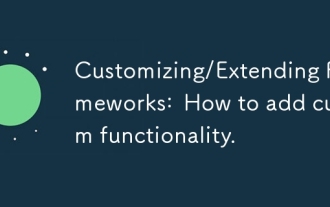
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
