PHP Master | Multi-Factor Authentication with PHP and Twilio
Core points
- Multifactor authentication (MFA) requires at least two different verification methods, which are much safer than traditional single-factor authentication. A practical MFA method is to ask the user to provide a password and a confirmation token sent to their phone via SMS or voice calls.
- Twilio provides infrastructure and APIs that developers can use to write interactive phone applications, including multi-factor authentication systems. Developers can use TwiML (Twilio Markup Language) and its REST API to make and receive calls and send and receive text messages through Twilio.
- Using Twilio to implement multi-factor authentication involves creating a new instance of the Services_Twilio class, accessing its API through the account property, and sending SMS messages or initiating voice calls through the create() method of the SMS message instance resource or the Calls instance resource.
- The multi-factor authentication process involves showing the user a login form to start the process, verifying their credentials, sending their code to their phone, and authorizing the user only after she submits the correct code. This process can be customized and enhances features such as resending a confirmation code link, locking an account if an incorrect code is provided, and logging of authentication events.
There are many ways to confirm the identity of a user: refer to some aspects of the user themselves (such as biometrics), ask what the user knows (such as passwords), or ask what the user actually has (such as an RFID card). Traditional websites implement single-factor authentication, which only requires the user's password. But on the other hand, multifactor authentication requires at least two different methods of verification and is much safer. Using biometrics to verify someone’s access to your website may still be a long way to go, with many technical barriers and civil liberties issues to overcome. A more practical multi-factor authentication method is to implement options from two other categories, such as requiring a password and a confirmation token sent to the user via SMS or voice calls (the user knows the password and has a cell phone). This is what you will learn how to achieve in this article. It can be frustrating if you do phone integration from scratch, and in most cases it is not practical to build your own infrastructure (although it can be done with programs like commodity hardware and Asterisk). Fortunately, Twilio provides infrastructure and APIs that developers can easily write interactive phone applications. I will use their services in this article. You can make and receive calls and send and receive text messages through Twilio using TwiML (Twilio markup language) and its REST API. You can use the API directly or use one of the available auxiliary libraries. The library I will use here is twilio-php, which is a library published and officially supported by Twilio. So, are you ready to learn how to implement multi-factor authentication? Continue reading!
Connect with PHP
Connecting to a Twilio service is as simple as including the twilio-php library and creating a new instance of the Services_Twilio class. The constructor of this object accepts the SID and authentication tokens of your account that are listed on the dashboard page of your Twilio account after you register their services. With the available Services_Twilio instances, you can access its API through the account property. account exposes an instance of Services_Twilio_Rest_Account that represents your Twilio account. I only use one account here, but can have multiple sub-accounts. This may be useful for breaking down your interactions based on your needs. You can read the Twilio documentation to learn more about sub-accounts.
<?php require "Services/Twilio.php"; define("TWILIO_SID", "…"); define("TWILIO_AUTH_TOKEN", "…"); $twilio = new Services_Twilio(TWILIO_SID, TWILIO_AUTH_TOKEN); $acct = $twilio->account;
account instance exposes several other properties, including calls and sms_messages. These are instances of objects such as Services_Twilio_Rest_Calls and Services_Twilio_Rest_SmsMessages that encapsulate REST resources used to issue your calls and messages, respectively. However, you rarely use these objects outside the create() method, and the documentation calls their properties exposing them "instance resources". I will do the same thing to help avoid any confusion.
Send text message
Send SMS messages is done through the create() method of the SMS message instance resource ($acct->sms_messages). This method accepts three parameters: your account's Twilio number (similar to the "Sender Address" of an email), the recipient's number ("Recipient Address"), and your message text (up to 160 character).
<?php $code = "1234"; // some random auth code $msg = $acct->sms_messages->create( "+19585550199", // "from" number "+19588675309", // "to" number "Your access code is " . $code );
Behind the scenes, the twilio-php library makes some POST requests for Twilio API on your behalf. An instance of Services_Twilio_Rest_SmsMessage is returned by a call that encapsulates information about the message. You can view a complete list of what information is provided in the documentation, but perhaps more important values are exposed by the status and price attributes. status Displays the status of the text message (queued, sent, sent or failed), while price displays the amount of the message charged for your account.
Send voice call
Starting a voice call is done through the create() method of the Calls instance resource ($acct->calls). As with sending text messages, you need to provide your account number, recipient number, and message. However, in this case, the message is the URL of the TwiML document describing the nature of the call.
<?php // Note spaces between each letter in auth code. This forces // the speech synthesizer to enunciate each digit. $code = "1 2 3 4"; $msg = $acct->calls->create( "+19585550199", // "from" number "+19588675309", // "to" number "http://example.com/voiceCall.php?code=" . urlencode($code) );
library again makes a POST request on your behalf and makes a voice call. When the called person answers the call, Twilio's process will retrieve and execute the commands provided in the XML of the callback URL. In the example of starting a voice call above, I have passed the confirmation code as a GET parameter to the URL of the callback script. When Twilio retrieves the URL, PHP uses this parameter when rendering the response. Building a call flow requires only a small number of TwiML tags, but you can use them to define rather complex processes (such as phone tree menus, etc.). However, the basic call flow for such scenarios can be generated by PHP, as shown below:
<?php require "Services/Twilio.php"; define("TWILIO_SID", "…"); define("TWILIO_AUTH_TOKEN", "…"); $twilio = new Services_Twilio(TWILIO_SID, TWILIO_AUTH_TOKEN); $acct = $twilio->account;
The TwiML tags used here are Response (root element), Say (providing the text Twilio will say), and Gather (collecting user input). When uttering text indicated by the Say child element, Twilio will also listen for user input due to Gather and pause afterwards for five seconds so that the user can enter their response. If Gather timed out without input, it exits and executes subsequent Say text and terminates the call. Otherwise, the input will be sent back to the action URL via POST for further processing. Gather's Twilio documentation is very good at explaining behaviors and the element properties you can use to modify behaviors, and even lists several examples. I suggest you read it quickly. Before proceeding, there is one more thing to note; I have added spaces between each number in the auth code in the startup script. This forces the speech synthesizer to pronounce one by one, saying "one two three four" instead of "one thousand two hundred thirty four". For speech synthesis, sometimes what we see in the text is not always what we get. If it brings better clarity and understanding to your callee, it is OK to modify or misspel voice conversations.
Implement multi-factor authentication
Now that you have learned the basic workflow for SMS and voice interaction with Twilio, it’s time to see how it fits into the login process. What you see here is very simple and straightforward, and for clarity I've reduced the accompanying code, as the details of your own login process are bound to vary. A login form for starting the process should be displayed to the user. Form submission causes you to verify their credentials and reject invalid credentials, but valid credentials should not immediately authenticate users to your application. Instead, treat the user as "partially verified", which is a state that allows her to view the second form of the code requested has been sent to her phone. The user should be authorized only after she submits the correct code.
<?php $code = "1234"; // some random auth code $msg = $acct->sms_messages->create( "+19585550199", // "from" number "+19588675309", // "to" number "Your access code is " . $code );
The above code is for illustration only. In your actual application, you may want to consider adding the following:
- Add a link to resend the confirmation code to the user's phone.
- If the user decides not to continue the process, add an unlink to the code request form. Regarding the above code, such a link needs to be unset
$_SESSION["username"]
because the value acts as a "partial authentication" flag for$_SESSION["isAuthenticated"]
in addition to storing the username. - If the wrong code is provided, add throttling or account lock.
- Login the authentication event somewhere depending on your level of paranoia or the compliance requirements you face. (Apps that require multi-factor authentication are usually sensitive enough to require audit trail!)
In addition, you may want to consider creating a sufficiently complex authentication code for your purposes. 4-digit codes are very common, but you are not limited to that. If you choose to use a mix of letters or alphanumeric values, I recommend avoiding confusing values (such as number 0 with letter O, number 1 with letter I, etc.).
Summary
The popularity of affordable mobile devices and IP phones has added other channels to interact with users, and in this article you learn a way to leverage these channels by using the “cloud communication” service Twilio, a “cloud communication” service, to leverage these channels by enabling multi-factor authentication, . I hope you find this article helpful in understanding multifactor authentication and Twilio. Feel free to comment below to share how you can use Twilio in your own app or implement multi-factor authentication. I would love to hear what you think!
(Picture from Fotolia)
FAQs (FAQs) on Multi-factor Authentication with PHP and Twilio
- How secure is it to use PHP and Twilio for multi-factor authentication?
Multifactor authentication (MFA) is very secure with PHP and Twilio. It adds an additional layer of security by requiring users to provide at least two ways of authentication before accessing their account. The first form is usually a password, and the second form can be a code sent to the user's phone via text messages. Even if they have a password, this makes it harder for unauthorized users to access their accounts.
- Can I customize the authentication process?
Yes, you can customize the authentication process to suit your needs. Twilio's API is very flexible and allows you to adjust the authentication process to your specific requirements. You can choose the type of second factor (such as text messages, voice calls or emails), message content, and more.
- How reliable is Twilio to send an authentication code?
Twilio is a reliable platform for sending authentication codes. It has a strong infrastructure and provides high delivery rates. It also provides real-time delivery reports so you can track the status of messages.
- What if the user loses his phone?
If a user loses his phone, they can still access their account using the alternate code. These codes are generated when setting up MFA and should be saved in a safe place. Users can enter these codes instead of the codes sent to their phones.
- Can I use MFA with PHP and Twilio for large applications?
Yes, you can use MFA with PHP and Twilio for large applications. Twilio’s infrastructure is designed to handle a large amount of messages, making it suitable for applications with a large number of users.
- How to test MFA implementation?
You can test your MFA implementation by creating a test account and completing the authentication process. Twilio also provides a sandbox environment where you can test your code without sending actual messages.
- Is it possible to implement MFA without encoding?
While some MFA solutions can be used for non-coding, implementing MFA using PHP and Twilio requires some coding knowledge. However, the process is simple and there are a lot of resources to help you.
- How much does it cost to use Twilio for MFA?
The cost of using Twilio for MFA depends on the number of messages you send. Twilio charges by message, so the more messages you send, the higher the fee. However, Twilio offers competitive prices and batch discounts.
- Can I use MFA with PHP and Twilio for non-web applications?
Yes, you can use MFA with PHP and Twilio for non-web applications. You can implement MFA in any application that can send HTTP requests and process HTTP responses.
- What other services does Twilio offer?
In addition to MFA, Twilio also offers a range of communication services including voice, video, chat and email. These services can be used to build a variety of applications, from customer service systems to marketing automation tools.
The above is the detailed content of PHP Master | Multi-Factor Authentication with PHP and Twilio. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


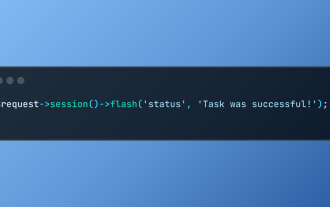
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
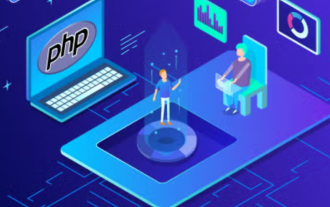
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
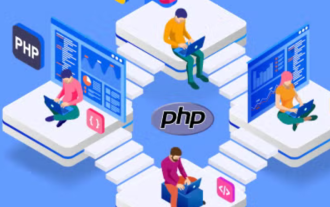
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
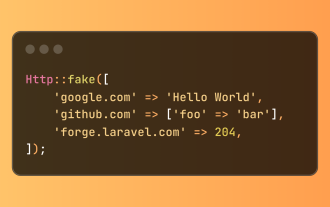
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
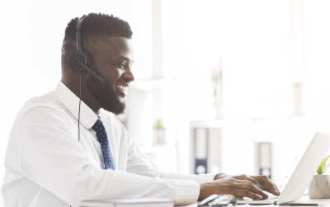
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
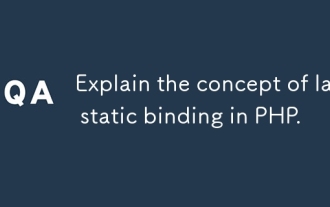
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
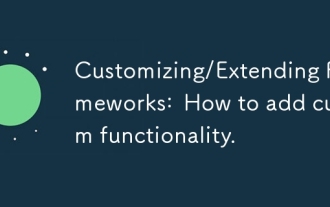
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
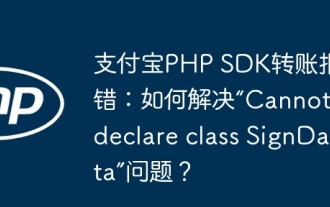
Alipay PHP...
