Implement Two-Way SMS with PHP
This article explores the intricacies of building two-way SMS applications using PHP, focusing on the complexities beyond simpler one-way systems. We'll cover the lifecycle, implementation details, and crucial considerations for choosing an SMS gateway.
Key Differences and Lifecycle:
Unlike one-way SMS (e.g., Gmail notifications), two-way SMS allows users to initiate conversations and receive application-generated responses. This involves a four-step lifecycle:
- User to Gateway: The user sends a message to a designated shortcode or long number (provided by the SMS gateway).
- Gateway to Server: The gateway forwards the message to your application server via HTTP GET or POST requests (often XML or SOAP).
- Server to Gateway: Your PHP application processes the message, generates a response, and sends it back to the gateway using the gateway's API.
- Gateway to User: The gateway delivers the response to the user.
Implementation with PHP and Clickatell (Example):
Choosing an SMS gateway (like Clickatell, Twilio, Nexmo, or Plivo) is crucial. Consider factors such as cost, reliability, delivery rates, API support, and customer service.
This example uses Clickatell to illustrate handling incoming messages via HTTP GET:
<?php $fromNo = $_GET["from"]; $toNo = $_GET["to"]; $message = $_GET["text"]; $msgID = $_GET["moMsgId"]; // Process the user command and generate output... ?>
For XML POST requests, the process involves parsing the XML data:
<?php $data = $_POST["data"]; $xmlDoc = new DOMDocument(); $xmlDoc->loadXML($data); // Extract data from XML... ?>
Handling Long Messages (UDH):
Long messages are split into segments by the gateway. The User Data Header (UDH) contains information to reassemble these segments. The PHP code needs to check for UDH and reconstruct the complete message before processing. An example of handling UDH is shown below:
<?php // ... (previous code) ... $udh = $_GET["udh"]; $total = 1; $count = 1; if ($udh) { $tmp = str_split($udh, 2); $total = hexdec($tmp[4]); $count = hexdec($tmp[5]); } if ($count != $total) { // Save message part in database } else if ($total != 1) { // Retrieve and combine previous parts from database } // ... (process the complete message) ... ?>
Sending Responses:
Sending responses involves using the gateway's API. Again, using Clickatell as an example:
<?php $message = array( "to" => "+15551234567", // Recipient number "from" => "+15559876543", // Your registered number "text" => "Your response message", "api_id" => API_KEY, // Your API key // ... other parameters ... ); // ... (cURL request to send the message) ... ?>
Conclusion:
Building a two-way SMS application with PHP requires careful consideration of the message lifecycle, handling of long messages using UDH, and the selection of a suitable SMS gateway. This guide provides a foundational understanding of the process and essential code examples to get you started. Remember to consult your chosen gateway's API documentation for specific details and parameters.
The above is the detailed content of Implement Two-Way SMS with PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


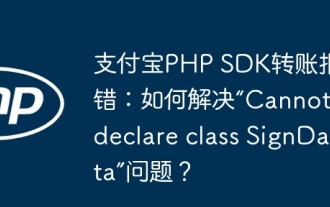
Alipay PHP...
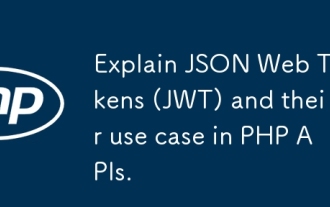
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
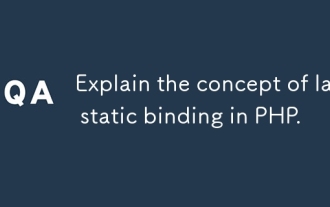
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
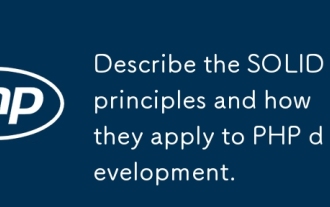
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
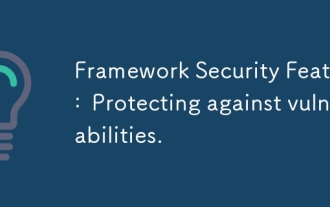
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
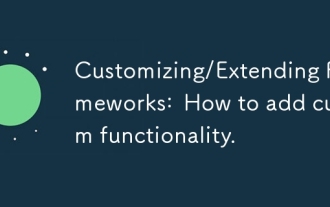
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
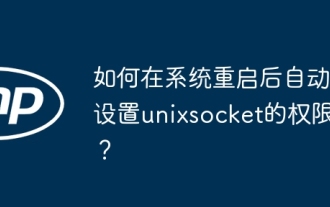
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
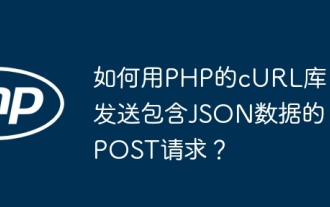
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
