Using Faker to Generate Filler Data for Automated Testing
The development of many websites and applications requires various types of data to simulate how real life works. During the testing and development stages of a project, we often use fake data to fill databases, UI elements, and so on.
Writing your own code to generate fake data for your project can be very cumbersome. In this tutorial, you will learn how to generate fake data using the proven Faker library in PHP.
Beginner
I want to clarify a few points before continuing.
The original fake library was fzaninotto/Faker. However, it was archived by the owner on December 11, 2020. Now, the library branch called FakerPHP/Faker is continuing its development work. If you are trying to decide which one you should use in your project, select FakerPHP.
Faker from FakerPHP requires that your PHP version be greater than or equal to 7.4. This is in contrast to PHP >= 5.3.3 supported by the original library.
From now on, all references to Faker in this tutorial will refer to newer branch versions.
You can install Faker using composer by running the following command:
<code>composer require fakerphp/faker</code>
This will create a composer.json file for you and install all the required packages in the vendor directory.
After the installation is complete, you can start using the library in your project by adding these two lines to your code:
require 'vendor/autoload.php'; $faker = Faker\Factory::create();
The first line includes the faker library in our project.
In the second line, we initialize a faker generator using a call to the static Factory class of the Generator instance, which we can use to generate various fake data.
Generate false names, addresses and phone numbers
Provider object is used to generate random data. Calls to the Generator instance that we bundled with the default provider.
The default provider generates name, address, phone number, company details, etc. based on the United States. These calls add providers in the background:
$faker = new Faker\Generator(); $faker->addProvider(new Faker\Provider\en_US\Person($faker)); $faker->addProvider(new Faker\Provider\en_US\Address($faker)); $faker->addProvider(new Faker\Provider\en_US\PhoneNumber($faker)); $faker->addProvider(new Faker\Provider\en_US\Company($faker)); $faker->addProvider(new Faker\Provider\Lorem($faker)); $faker->addProvider(new Faker\Provider\Internet($faker));
You can generate fake names in the faker by simply calling the name attribute. Each call will output a different random name. Here is an example:
require 'vendor/autoload.php'; $faker = Faker\Factory::create(); $fake_names = []; for($i = 0; $i < 10; $i++){ $fake_names[] = $faker->name; } /* Array ( [0] => Gabe Mann Jr. [1] => Lazaro Leuschke [2] => Angie Welch Sr. [3] => Prof. Kirk Krajcik III [4] => Sadye Mosciski [5] => Danyka Braun [6] => Jacinthe Dickinson [7] => Clifton Beahan [8] => Dr. Jan Casper MD [9] => Adelia Schimmel ) */ print_r($fake_names);
If you are not interested in your full name, you can also request only your first or last name.
require 'vendor/autoload.php'; $faker = Faker\Factory::create(); $first_names = []; for($i = 0; $i < 10; $i++){ $first_names[] = $faker->firstName; } // Outputs: Dylan, Ariane, Doris, Reilly, Jamar, Merl, Maverick, Frederik, Lucius, Madyson echo implode(', ', $first_names);
Suppose you only need male or female names. In this case, you can simply call the firstNameMale() and address() methods or access phoneNumber(), phoneNumberWithExtension().
require 'vendor/autoload.php'; $faker = Faker\Factory::create(); /* Phone Number: +1-724-494-3101 Phone Number (Extension): (475) 499-3999 x9969 Phone Number (Toll Free): 855.618.0155 Phone Number: 1-660-934-8668 Phone Number (Extension): 351-533-3301 x1602 Phone Number (Toll Free): 844-530-4671 */ for($i = 0; $i < 2; $i++){ echo "Phone Number: ".$faker->phoneNumber()."\n"; echo "Phone Number (Extension): ".$faker->phoneNumberWithExtension()."\n"; echo "Phone Number (Toll Free): ".$faker->tollFreePhoneNumber()."\n\n"; }
Generate fake details specific to locale settings
Suppose you are developing an application that is targeted at a specific region. In this case, you may want to use data that reflects the target market. For example, an application for Nigerians will benefit from owning a Nigerian name. Similarly, applications for Indian users may wish to use Indian addresses.
The trick to generate locale-specific data is to pass the locale to the randomNumber() method, which by default generates a random number with a specified number of digits. You can pass the unixTime() method to get the Unix timestamp value between 0 and the current time or the specified time. Random dateTime() methods can also be generated. Again, the default maximum is the current date and time.
If you want to generate the dateTimeAD() method, it returns the date between January 1, 1, and the given maximum value.
You can use the time() method to generate random dates and times in a specific format.
Suppose you need to get the dateTimeBetween() method.
<code>composer require fakerphp/faker</code>
Generate tags using fake data
Now that we know how to use Faker to generate different types of numeric values, we can combine their results to create XML or HTML documents, fill the database with numeric values, and so on. For example, consider the following code, which generates random configuration files for different people.
require 'vendor/autoload.php'; $faker = Faker\Factory::create();
After generating random values, you can do anything to them. In this case, we output them in the Base class to generate the data type we want. If you are looking for a library that generates random data, Faker is definitely worth a try.
The above is the detailed content of Using Faker to Generate Filler Data for Automated Testing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


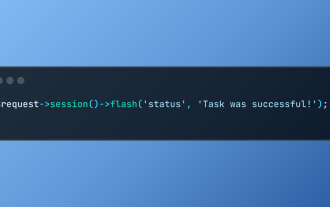
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
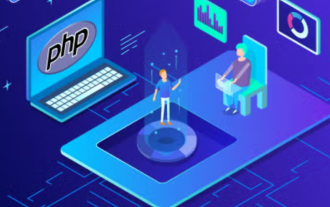
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
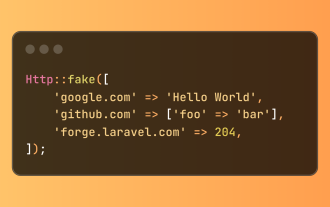
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
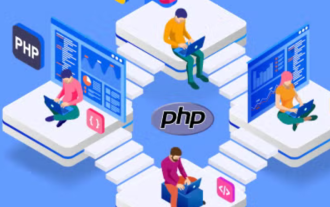
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
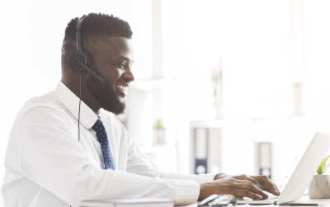
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
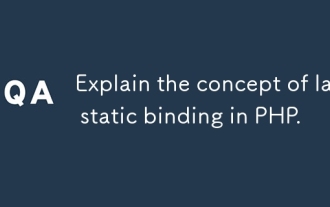
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
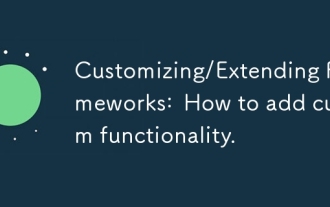
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
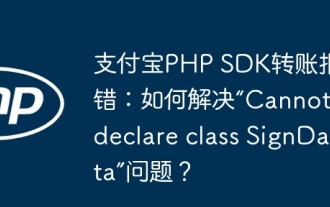
Alipay PHP...
