jQuery Ajax Loading using ajaxStart/ajaxSetup
jQuery AJAX Loading with ajaxStart()
and ajaxStop()
This guide demonstrates using jQuery's ajaxStart()
and ajaxStop()
methods to manage loading indicators during AJAX requests. We'll cover several approaches, including using these methods directly, incorporating them into AJAX calls, and leveraging ajaxSetup()
.
Method 1: Direct Use of ajaxStart()
and ajaxStop()
This method displays a loading image and disables a form's submit button while an AJAX request is in progress. Once the request completes, the image is hidden, and the button is re-enabled.
var $form = $('#form'); $form.find('.loading') .hide() .ajaxStart(function() { $(this).show(); $form.find('.submit').attr('disabled', 'disabled'); }) .ajaxStop(function() { $(this).hide(); $form.find('.submit').removeAttr('disabled'); });
Method 2: Loading Indicator Before and complete
Handler
Alternatively, you can show the loading indicator before the AJAX call and use the complete
handler to hide it.
// Show loading image, disable submit button $form.find('.msg').remove(); $form.find('.loading').show(); $form.find('.submit').attr('disabled', 'disabled'); // AJAX call $.ajax({ // ... your AJAX settings ... complete: function() { $form.find('.loading').hide(); $form.find('.submit').removeAttr('disabled'); } });
Method 3: Using ajaxSetup()
for Global Handling
For a global solution affecting all AJAX requests, use ajaxSetup()
. This hides the loading image and enables the form after all AJAX requests complete.
$.ajaxSetup({ complete: function() { $form.find('.loading').hide(); $form.find('.submit').removeAttr('disabled'); } });
Frequently Asked Questions
The following Q&A section addresses common questions about using ajaxStart()
, ajaxStop()
, and related jQuery AJAX methods. Note that the original FAQ section has been condensed and rephrased for clarity and conciseness.
-
ajaxStart()
Purpose:ajaxStart()
executes a function when an AJAX request begins and no other requests are active. It's ideal for displaying loading indicators. -
Showing a Loading Spinner: Create a spinner element (e.g.,
<div id="spinner">). Use <code>$(document).ajaxStart(function(){ $("#spinner").show(); });
to display it. -
Hiding the Spinner: Use
$(document).ajaxStop(function(){ $("#spinner").hide(); });
to hide the spinner when all AJAX requests complete. -
ajaxStart()
with Specific Requests: BindajaxStart()
to the element initiating the AJAX request (e.g.,$("#myButton").ajaxStart(...)
). -
ajaxStart()
vs.ajaxSend()
:ajaxStart()
triggers only when no other AJAX requests are running;ajaxSend()
triggers for every request. -
Error Handling: Use
ajaxError()
alongsideajaxStart()
to handle errors. For example:$(document).ajaxStart(...).ajaxError(function(event, jqxhr, settings, thrownError){ ... });
-
Combining with Other AJAX Methods:
ajaxStart()
works with other jQuery AJAX methods (ajaxStop()
,ajaxComplete()
,ajaxError()
, etc.). -
Using with Shorthand Methods:
ajaxStart()
functions with shorthand methods like$.get()
and$.post()
.
This revised response provides a more concise and organized explanation of jQuery AJAX loading techniques, addressing the key aspects and common questions. The image remains in its original position.
The above is the detailed content of jQuery Ajax Loading using ajaxStart/ajaxSetup. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










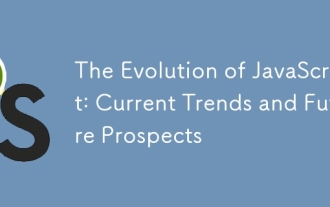
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
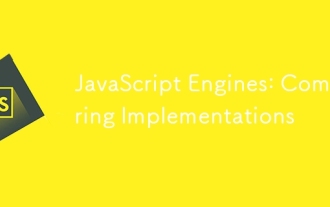
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
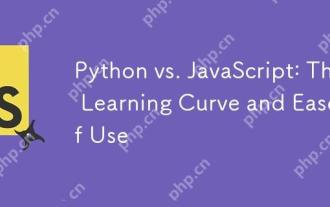
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
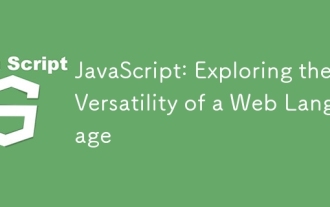
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
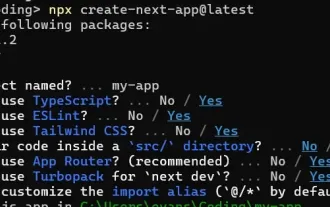
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
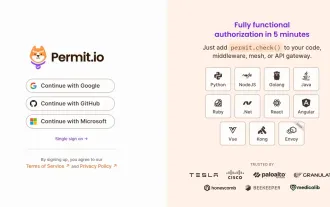
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
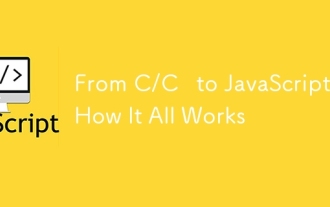
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
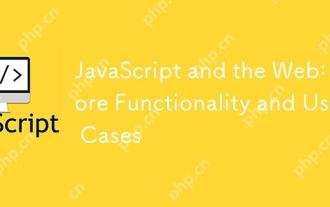
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
