


Let's Talk: Efficient Communication for PHP and Android, Part 1
Efficient Communication Between PHP and Android: A Two-Part Guide (Part 1)
Key Concepts
This two-part series demonstrates building a high-performance PHP REST web service for an Android app. We'll focus on efficient data serialization and compression techniques. This approach is adaptable to other mobile platforms. Basic PHP and Android development knowledge is assumed.
- RESTful Web Services: Leverage a PHP-based REST API as the communication bridge between your Android app and backend.
- Data Serialization & Compression: Optimize bandwidth usage by employing efficient data formats (like JSON and MessagePack) and compression algorithms (bzip2, gzip, deflate). Negotiate the best format based on client capabilities.
-
Asynchronous Operations: Use Android's
AsyncTask
to handle network requests on a background thread, preventing UI freezes. - Network Connectivity Check: Implement a check for network availability before initiating data requests.
A Typical Request/Response Cycle
-
Request: The Android app sends an HTTP request to the PHP REST service, specifying supported data serialization (e.g.,
application/json
,application/x-msgpack
) and compression formats (e.g.,bzip2
,gzip
,deflate
) via request headers. - Response: The server analyzes the request headers, selects compatible formats, applies them to the data, and sends a response including headers indicating the chosen formats and the processed data.
- Data Processing: The Android app uses the response headers to decompress and deserialize the received data.
Android App: Requesting Data
To make HTTP requests, your Android app needs the INTERNET
permission in AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET" />
The DataModel
class uses AndroidHttpClient
(Android 2.2 and above) to handle HTTP requests:
import android.net.http.AndroidHttpClient; import java.io.IOException; import org.apache.http.HttpResponse; import org.apache.http.client.methods.HttpGet; public class DataModel { // ... (rest of the class remains the same) }
The getData()
method sends a HttpGet
request, including headers specifying supported serialization (Accept
) and compression (Accept-Encoding
) formats. The 10.0.2.2
IP address is used for requests from the emulator.
Android App: Background Task Implementation
Data retrieval is asynchronous using AsyncTask
:
<uses-permission android:name="android.permission.INTERNET" />
GetDataTask
extends AsyncTask
, handling pre-execution (showing a progress dialog), background data retrieval using DataModel
, and post-execution (dismissing the dialog, handling potential errors, and processing the DataValueObject
).
Android App: Executing the Background Task
Before executing GetDataTask
, check for network connectivity:
import android.net.http.AndroidHttpClient; import java.io.IOException; import org.apache.http.HttpResponse; import org.apache.http.client.methods.HttpGet; public class DataModel { // ... (rest of the class remains the same) }
The networkIsAvailable()
method checks network status. onCreate()
executes GetDataTask
only if a network connection is available; otherwise, it displays an error message.
Conclusion (Part 1)
This part focused on the Android app's request setup. Part 2 will cover data serialization and compression on both the Android and PHP sides.
Frequently Asked Questions (FAQs) about Converting PHP Projects to Android Apps
The FAQs section remains largely unchanged, providing helpful information about the conversion process, challenges, and optimization strategies.
The above is the detailed content of Let's Talk: Efficient Communication for PHP and Android, Part 1. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


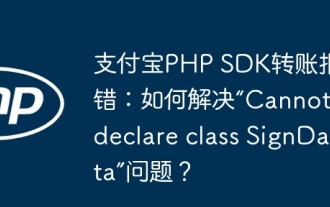
Alipay PHP...
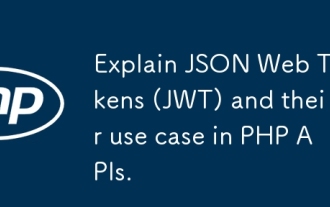
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
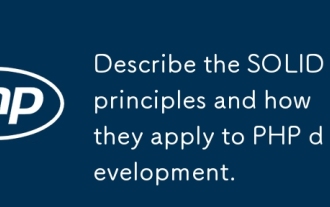
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
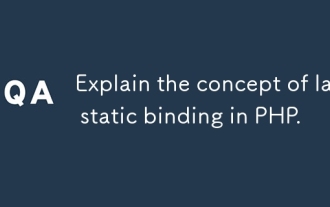
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
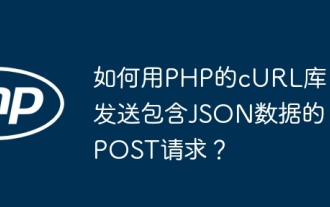
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
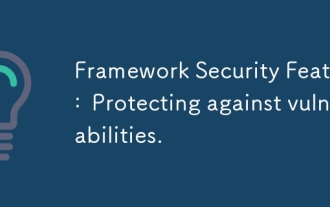
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
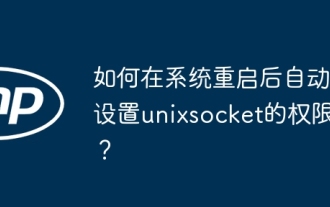
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
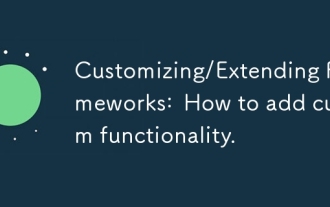
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
