How to Create a PHP/MySQL Powered Forum From Scratch
This tutorial guides you through building a PHP/MySQL-powered forum from the ground up. It's ideal for beginners learning PHP and database interaction.
Step 1: Database Table Creation
Effective application development begins with a robust data model. Our forum will have:
- Users: Individuals who interact with the forum.
- Topics: Discussion threads started by users.
- Categories: Sections organizing topics.
- Posts: Replies within topics.
Here's a visual representation of the database schema:
Each square represents a table, listing its columns and relationships. Let's examine the SQL for each table:
The CREATE TABLE
statement generates new tables. Field names are self-explanatory; we'll focus on data types.
-
user_id
(INT, PRIMARY KEY): Uniquely identifies each user. No two users share the sameuser_id
. -
user_name
(VARCHAR, UNIQUE): User's display name. Must be unique. -
user_pass
(VARCHAR): Stores the SHA1 hash of the user's password (for security). -
user_email
(VARCHAR): User's email address.
Similar primary keys exist in topics
, posts
, and categories
tables.
Foreign keys establish relationships between tables. A foreign key in one table references a primary key in another, ensuring data integrity. For example:
-
topic_by
intopics
referencesuser_id
inusers
. -
post_topic
inposts
referencestopic_id
intopics
. -
post_by
inposts
referencesuser_id
inusers
.
ON DELETE CASCADE
and ON UPDATE CASCADE
ensure data consistency across related tables. ON DELETE RESTRICT
prevents accidental data loss when deleting a user.
Step 2: Header/Footer System
Every forum page needs a consistent structure. header.php
includes:
- DOCTYPE declaration.
- Charset and meta tags.
- Link to the CSS stylesheet (
style.css
). - Basic forum title and navigation.
The footer.php
file (not shown) will provide a consistent closing structure for each page.
Step 3 - 7: User Authentication (signin.php, signout.php)
These steps detail user registration and login functionality. Key aspects include:
- Secure password hashing using
sha1()
. Never store passwords in plain text. - SQL injection prevention using parameterized queries (
mysqli_prepare()
andmysqli_stmt_bind_param()
). - Session management using
$_SESSION
variables to track logged-in users. - Error handling to provide feedback to the user.
Step 8: Displaying Categories (index.php)
The index.php
file retrieves and displays categories from the categories
table using a simple SQL query.
Step 9: Creating Topics (create_topic.php)
This section demonstrates creating new topics. It includes:
- Authentication check to ensure only logged-in users can create topics.
- A form for users to input topic details (subject, category, message).
- Database interaction using transactions (
mysqli_begin_transaction()
,mysqli_query()
,mysqli_commit()
,mysqli_rollback()
) to maintain data consistency.
Step 10: Viewing Topics (topic.php)
This step explains how to fetch and display individual topics and their associated posts. It uses LEFT JOIN
to combine data from the topics
and posts
tables, along with user information from the users
table.
Step 11: Displaying Topic Replies (topic.php)
This section builds upon the previous step, refining the display of replies within a topic, including user information.
Step 12: Adding Replies (reply.php)
This final step shows how to add replies to existing topics. It includes:
- Authentication check.
- A form for users to input their replies.
- Database interaction to insert new posts into the
posts
table.
This comprehensive tutorial provides a solid foundation for building a functional PHP/MySQL forum. Remember to always prioritize security and data integrity.
The above is the detailed content of How to Create a PHP/MySQL Powered Forum From Scratch. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




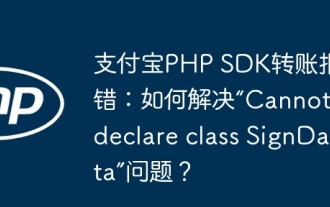
Alipay PHP...
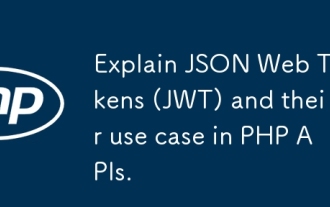
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
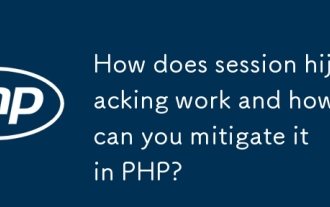
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
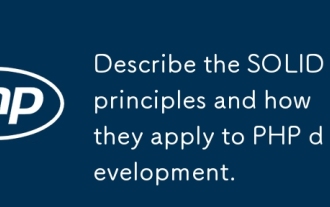
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
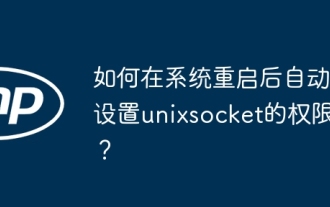
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
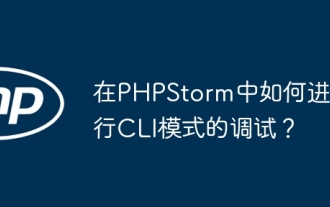
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
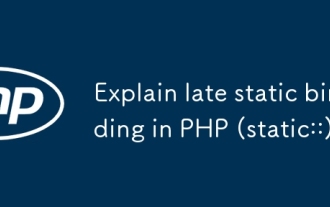
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
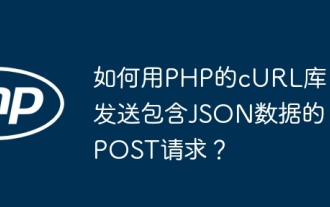
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
