Sanitize and Validate Data With PHP Filters
Effective data validation is crucial for secure and robust web forms. Invalid data can create security vulnerabilities and website malfunctions. This tutorial demonstrates how PHP's filter_var
function efficiently sanitizes and validates user inputs, preventing these issues.
Why Data Sanitization is Often Overlooked
Many developers find data validation tedious, often involving:
- Exhaustive comparisons against every conceivable input variation.
- Crafting complex regular expressions to handle all possibilities.
- Or a combination of both, leading to time-consuming work and a high error rate.
Fortunately, PHP offers a streamlined solution.
Leveraging PHP's filter_var
Function
PHP's filter_var
function simplifies the process. Its syntax is:
filter_var( mixed $value, int $filter = FILTER_DEFAULT, array|int $options = 0 ): mixed
$value
: The data to be filtered.$filter
: The filter ID (e.g.,FILTER_SANITIZE_EMAIL
,FILTER_VALIDATE_INT
).$options
: Optional parameters for filter customization. ReturnsFALSE
on filter failure.
Sanitizing Data with filter_var
Email Sanitization:
The FILTER_SANITIZE_EMAIL
filter removes illegal characters from email addresses. For example:
$email = "test\"';DROP TABLE users;--@example.com"; $sanitizedEmail = filter_var($email, FILTER_SANITIZE_EMAIL); echo $sanitizedEmail; // Outputs: test@example.com (malicious script removed)
URL Sanitization:
Similarly, FILTER_SANITIZE_URL
cleans URLs of harmful characters:
$url = "http://example.com/?param=<🎜>"; $sanitizedUrl = filter_var($url, FILTER_SANITIZE_URL); echo $sanitizedUrl; // Outputs: http://example.com/?param= (script removed)
Validating Data with filter_var
IP Address Validation:
$ip = "127.0.0.1"; if (filter_var($ip, FILTER_VALIDATE_IP)) { // Valid IP address } else { // Invalid IP address }
Integer Validation:
$foo = "123"; if (filter_var($foo, FILTER_VALIDATE_INT)) { // Valid integer } else { // Invalid integer }
Practical Application: An Email Submission Form
Let's build a simple email submission form to illustrate data sanitization and validation. The form collects: name, email, homepage, and message. Only valid data triggers email submission.
Step 1: Creating the Form (form.html):
<form method="post" action="form-email.php"> Name: <input type="text" name="name"><br><br> Email Address: <input type="email" name="email"><br><br> Home Page: <input type="url" name="homepage"><br><br> Message: <textarea name="message"></textarea><br><br> <input type="submit" name="Submit" value="Send"> </form>
Step 2: Handling Form Submission (form-email.php):
<?php $errors = ""; if (isset($_POST['Submit'])) { // ... (Validation and sanitization logic as shown in original example) ... if (empty($errors)) { // Send email using mail() function with sanitized data echo "Thank you for your message!"; } else { echo "Errors: <br>" . $errors; } } ?>
(Note: The complete validation and sanitization logic from the original example should be inserted into the if (isset($_POST['Submit']))
block in form-email.php
.)
Conclusion
This tutorial provides a foundation for using PHP's data filtering capabilities. While not exhaustive, it showcases the efficiency of filter_var
for secure and reliable data handling. Refer to the PHP manual's Data Filtering section for more advanced techniques. The image was generated using OpenAI's DALL-E 2.
The above is the detailed content of Sanitize and Validate Data With PHP Filters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










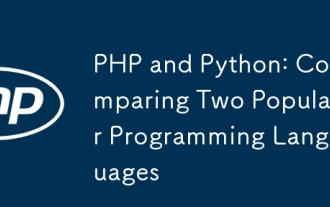
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
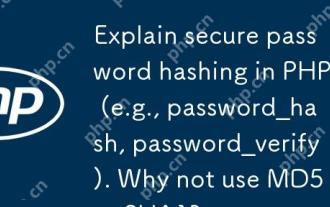
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
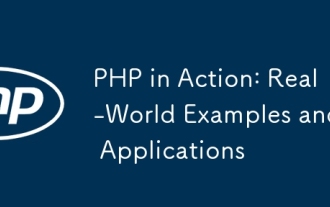
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
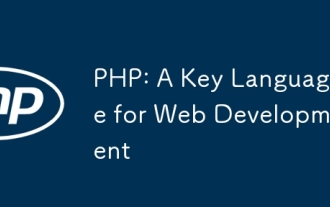
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
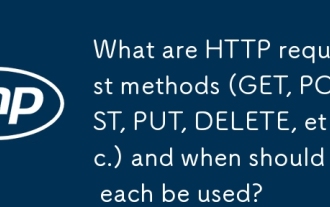
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
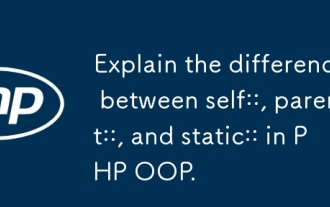
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
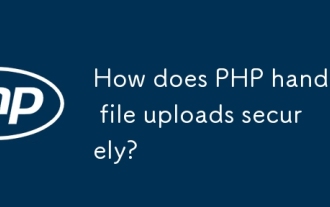
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
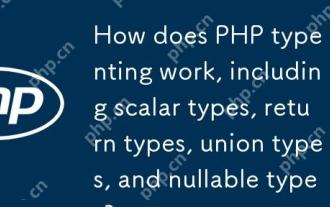
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
