10 Insanely Useful Django Tips
Unlocking Django's Potential: 10 Tips for Faster Development and Fewer Headaches
Django, a robust Python framework, simplifies web development through automation and clean coding principles. However, even experienced Django developers can benefit from these tips and tricks to streamline their workflow and avoid common pitfalls. This guide covers techniques ranging from basic best practices to more advanced optimization strategies.
1. Embrace Relative Paths in Configuration
Hardcoding absolute file paths in your Django settings is a recipe for disaster when moving projects or deploying to servers. Instead, use relative paths based on the current working directory. This ensures portability and minimizes configuration changes across different environments. A well-structured settings file should be independent of the project's filesystem location.
2. Leverage the {% url %} Tag for URL Management
Avoid hardcoding URLs directly in your templates. The {% url %} template tag provides a cleaner, more maintainable approach. It performs reverse lookups based on view names, preventing broken links when your urls.py
file changes. This simple technique significantly improves code robustness.
Image: Illustrative example of using the {% url %} tag
3. Optimize Database Performance with prefetch_related
Minimize database queries by utilizing prefetch_related
for efficient fetching of related objects in many-to-many or reverse foreign key relationships. This dramatically improves database performance, especially with complex data models. The following example illustrates its use:
class Author(models.Model): full_name = models.CharField(max_length=100) def __str__(self): return self.full_name class Course(models.Model): title = models.CharField(max_length=100) description = models.TextField() author = models.ForeignKey(Author) def __str__(self): return self.title # Efficient query using prefetch_related tutorials = Course.objects.prefetch_related('author').all()
4. Employ a Dedicated Media Server
Serving static files (images, CSS, JavaScript) from your Django application server is inefficient and impacts performance. Use a separate server like Nginx, a cloud storage service (Amazon S3, Google Cloud Storage, Azure), or a CDN for optimal delivery of static assets. This significantly enhances website speed and scalability.
5. Harness the Power of the Debug Toolbar
The Django debug toolbar is an invaluable debugging tool. It provides insights into database queries, template rendering times, and other performance metrics, assisting in identifying bottlenecks and optimizing your application.
Image: Screenshot of the Django debug toolbar in action
6. Implement Comprehensive Unit Testing
Write unit tests to ensure code correctness and maintain backward compatibility. Django's built-in testing framework simplifies this process, allowing you to use doctest
or unittest
for robust testing. Regular testing prevents regressions and improves code quality.
Image: Example of a Django unit test
7. Manage Dependencies with Virtual Environments
Python's dependency management can be challenging. Use virtual environments to isolate project dependencies, preventing conflicts between different projects and system-wide Python installations. Employ requirements.txt
to track project dependencies for reproducibility.
Image: Visual representation of a virtual environment
8. Utilize Class-Based Views for Reusability
Class-based views offer a more structured and reusable approach compared to function-based views. They simplify common tasks and improve code organization, making it easier to add logic and maintain consistency.
Image: Comparison of function-based and class-based views
9. Supercharge Performance with Memcached
For performance-critical applications, implement caching using Memcached. It significantly reduces database load and improves response times. While other caching options exist in Django, Memcached generally offers superior performance.
Image: Illustration of Memcached in a Django application
10. Leverage Django's Built-in Capabilities
Avoid reinventing the wheel. Django provides a comprehensive set of tools and features. Utilize its capabilities to avoid unnecessary custom scripting and ensure a clean, maintainable architecture. This reduces development time and complexity.
Image: Conceptual representation of Django's capabilities
By incorporating these tips into your Django development workflow, you'll significantly enhance efficiency, reduce debugging time, and build more robust and scalable applications. This updated post incorporates valuable contributions from Esther Vaati.
The above is the detailed content of 10 Insanely Useful Django Tips. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


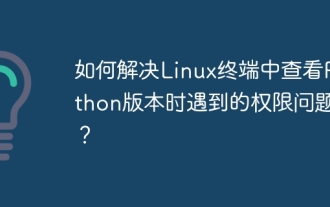
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
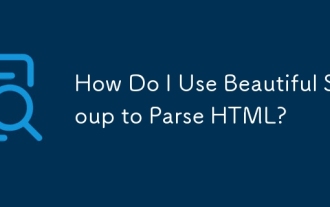
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
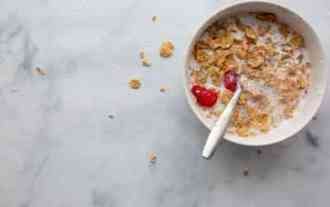
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
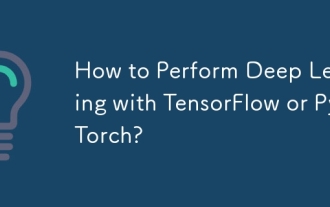
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
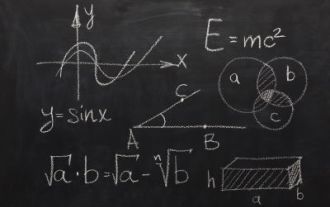
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti

This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
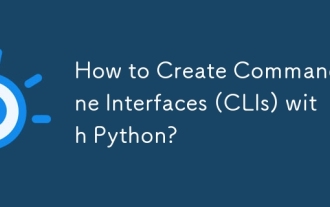
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
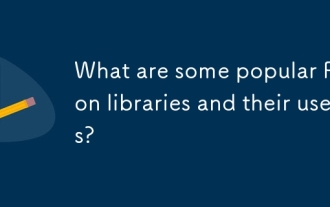
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
