jQuery AJAX Utility Helper Function
Core points
- This jQuery AJAX utility helper function can be used to store data locally on JavaScript objects, or to run JavaScript callback functions dynamically when ajax succeeds. This utility function reduces the need to write ajax functions in multiple files and keeps ajax definition calls in one place.
- This AJAX utility helper function is flexible and powerful, allowing developers to specify various settings for AJAX requests in a single function call. It can be used with other JavaScript libraries, but care should be taken to avoid potential conflicts.
- This AJAX utility helper function can handle errors using the error callback option. It can also send data to the server, load JSON data, cancel AJAX requests, send files to the server, and make synchronous AJAX requests, although the latter is not usually recommended due to the possibility of browser blocking and slowing web applications response speed.
Good morning for all jQuery lovers! Today, I will share with you a short ajax helper function I wrote that can receive some basic ajax settings and store data on JavaScript objects, or run JavaScript callbacks dynamically when ajax succeeds. Using ajax utility functions will save you time writing ajax functions in multiple files. It can also keep your ajax definition call in one place if you need specific requirements for ajax (such as adding loading images or specific error handlers). Related articles: - 6 real-time examples of jQuery Ajax - The difference between GET and POST in jQuery AJAX
AJAX Utility Helper Function
This ajax helper function can be included in your JavaScript utility object.
/** * JQUERY4U.COM * * 为其他JavaScript对象提供实用程序函数。 * * @author Sam Deering * @copyright Copyright (c) 2012 JQUERY4U * @license http://jquery4u.com/license/ * @since Version 1.0 * @filesource js/jquery4u.util.js * */ (function($,W,D) { W.JQUERY4U = W.JQUERY4U || {}; W.JQUERY4U.UTIL = { /** * AJAX辅助函数,可用于动态存储数据或在成功后运行函数。 * @param callback - 'store' 用于本地存储数据,'run' 用于运行回调函数。 * @param callbackAction - 数据存储位置。 * @param subnamespace - 数据存储/函数运行的命名空间。 */ ajax: function(type, url, query, async, returnType, data, callback, callbackAction, subnamespace) { $.ajax( { type: type, url: url + query, async: async, dataType: returnType, data: data, success: function(data) { switch(callback) { case 'store': JQUERY4U[subnamespace]["data"][callbackAction] = data; //存储数据 break; case 'run': JQUERY4U[subnamespace][callbackAction](data); //使用数据运行函数 break; default: return true; } }, error: function(xhr, textStatus, errorThrown) { alert('ajax加载错误...'); return false; } }); } } })(jQuery,window,document);
How to use AJAX utility function
The following is an example of how to use ajax utility function: 1) Get the data with ajax and store it on your JS object 2) Get the data with ajax and run a callback function that passes the data
/** * JQUERY4U.COM * * 使用AJAX实用程序函数的示例JavsScript对象。 * * @author Sam Deering * @copyright Copyright (c) 2012 JQUERY4U * @license http://jquery4u.com/license/ * @since Version 1.0 * @filesource js/jquery4u.module.js * */ (function($,W,D) { W.JQUERY4U = W.JQUERY4U || {}; W.JQUERY4U.MODULE = { data: { ajaxData: '' //用于存储ajax数据 }, init: function() { this.getData(); //存储数据测试 this.runFunc(); //运行函数测试 }, //调用ajax并在ajax成功后保存数据的示例函数 getData: function() { JQUERY4U.UTIL.ajax('GET', 'jquery4u.com/data.php', '?param=value¶m2=value2', false, 'HTML', '', 'store', 'ajaxData', 'MODULE'); //ajax数据在ajax成功后存储在JQUERY4U.MODULE.data.ajaxData中 }, //调用ajax并在ajax成功后运行函数的示例函数 runFunc: function() { var data = ['传递给服务器端脚本的一些数据']; JQUERY4U.UTIL.ajax('POST', 'jquery4u.com/data.php', '', true, 'HTML', data, 'run', 'ajaxCallbackFunction', 'MODULE'); //JQUERY4U.MODULE.ajaxCallbackFunction在ajax成功后被调用 }, //ajax成功后调用的函数 ajaxCallbackFunction: function(data) { //对返回的数据执行某些操作 } } $(D).ready(function() { JQUERY4U.MODULE.init(); }); })(jQuery,window,document);
This ajax function works perfectly, but it's still in development and I'm tweaking it so I'll try to keep the code updated.
Frequently Asked Questions about jQuery AJAX Utility Helper Functions (FAQ)
What is jQuery AJAX utility helper function and how does it work?
jQuery AJAX utility helper function is a powerful tool that allows developers to create asynchronous web applications. It works by sending HTTP requests to the server and receiving responses without having to reload the entire page. This function is particularly useful in enhancing the user experience, as it allows creating faster and more interactive web applications.
How to use jQuery AJAX utility helper function in my code?
To use jQuery AJAX utility helper function, you first need to include the jQuery library in the HTML file. You can then use the $.ajax() method to send an asynchronous request to the server. This method takes an option object as a parameter where you can specify details, such as the URL to send the request, the type of request (GET, POST, etc.), the data type of the response, and the callback function to process the response.
jQuery What is the main difference between AJAX utility helper functions and other AJAX methods?
The jQuery AJAX utility helper functions are more flexible and powerful than other AJAX methods. It allows you to specify various settings for AJAX requests in a single function call. Other AJAX methods such as $.get() and $.post() are simpler and easier to use, but have poor flexibility and weaker control.
Can I use jQuery AJAX utility helper functions with other JavaScript libraries?
Yes, you can use jQuery AJAX utility helper functions with other JavaScript libraries. However, you need to be aware of possible conflicts between jQuery and other libraries. To avoid conflicts, you can use jQuery's noConflict() method, which allows you to create a new alias for jQuery and free the $ symbol for use by other libraries.
How to handle errors in jQuery AJAX utility helper function?
You can use the error callback option to handle errors in jQuery AJAX utility helper function. If the AJAX request fails, this function is called. It accepts three parameters: jqXHR object, a string describing the error type, and (if it happens) an optional exception object.
How to use jQuery AJAX utility helper function to send data to server?
You can use the data option in the jQuery AJAX utility helper function to send data to the server. This option allows you to specify the data to be sent to the server as a string, a normal object, or a JavaScript array.
Can I load JSON data using jQuery AJAX utility helper function?
Yes, you can load JSON data using jQuery AJAX utility helper function. You can specify the response's data type as "json" in the options object and jQuery will automatically parse the JSON data for you.
How to cancel AJAX request in jQuery?
You can cancel the AJAX request in jQuery by calling the abort() method of the jqXHR object returned by the $.ajax() method. This will immediately terminate the request and trigger an error callback.
Can I send files to the server using jQuery AJAX utility helper function?
Yes, you can use the jQuery AJAX utility helper function to send files to the server. You need to set the processData option to false to prevent jQuery from converting data to query strings and the contentType option to false to prevent jQuery from setting the default content type for the request.
How to use jQuery to synchronize AJAX requests?
Although it is generally recommended to use asynchronous AJAX requests for a better user experience, you can synchronize AJAX requests in jQuery by setting the async option to false in the option object. However, be aware that synchronous requests can block the browser and slow down the response of your web application.
The above is the detailed content of jQuery AJAX Utility Helper Function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










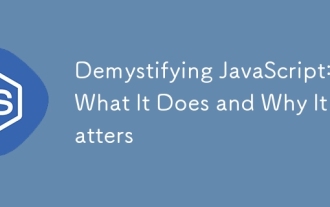
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
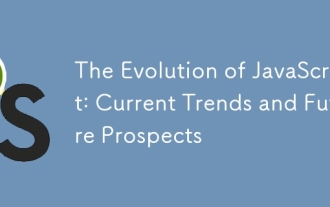
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
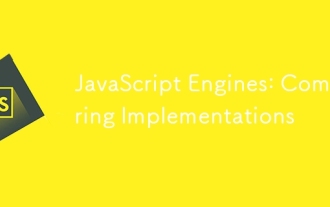
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
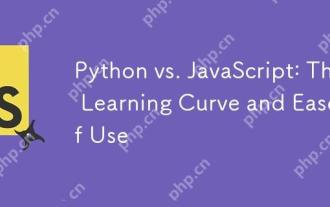
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
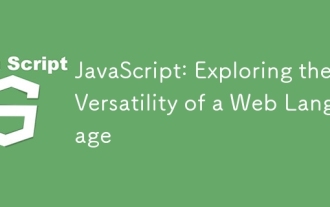
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
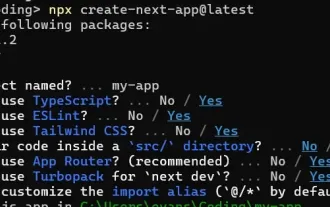
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
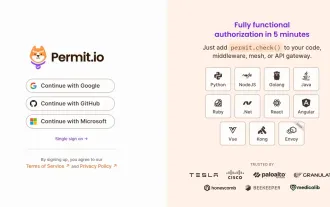
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
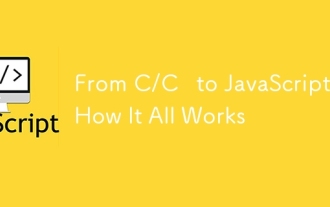
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
