Magic Methods and Predefined Constants in PHP
Core points
- PHP provides predefined constants and magic methods to enhance code functionality. Predefined constants provide read-only information about code and PHP, while magic methods are names reserved in the class to enable special PHP features.
- Predefined constants (all capital letters enclosed with double underscores) provide information about the code. Examples include
__LINE__
(returns the line number in the source file),__FILE__
(represents the file name, including its full path),__DIR__
(represents the file path only),__CLASS__
(returns the name of the current class),__FUNCTION__
(returns the name of the current function),__METHOD__
(represents the name of the current method), and__NAMESPACE__
(returns the name of the current namespace). - The magic method provides a mechanism to link to special PHP behaviors. They include
__construct()
(used to create an object instance of the class),__destruct()
(called when an object is destroyed by a garbage collector in PHP),__get()
(called if the property is undefined or inaccessible and is called in the getter context),__set()
(called for an undefined property in the setter context),__isset()
(check if the property is set),__unset()
(receive a parameter, namely the name of the property the program wants to unset), and__toString()
(help represent the object as a string).
PHP provides a special set of predefined constants and magic methods for the program. Unlike constants set with define()
, the values of these constants depend on where they are used in the code and are used to access read-only information about the code and PHP. Magic methods are reserved method names that you can use in your class for hooking to special PHP features. If you haven't learned about the magic methods and constants of PHP, this article is for you! I'll review some more useful methods and how to use them in my code.
Predefined constant
Predefined constants are used to access information about the code. The constants here are written in all capital letters enclosed with double underscores, such as __LINE__
and __FILE__
. Here are some useful constants provided by PHP:
-
__LINE__
Returns the line number of the constants appearing in the source file, as shown below:
<?php echo "line number: " . __LINE__; // line number: 2 echo "line number: " . __LINE__; // line number: 3 echo "line number: " . __LINE__; // line number: 4 ?>
__FILE__
Indicates the name of the file, including its full path, as shown below:
<?php echo "the name of this file is: " . __FILE__; // the directory and name of file is: C:wampwwwindex.php ?>
__DIR__
Only represent the path to the file:
<?php echo "the directory of this file is: " . __DIR__; // the directory of this file is: C:wampwww ?>
__CLASS__
Returns the name of the current class:
<?php class Sample { public function __construct() { echo __CLASS__; } } $obj = new Sample(); // Sample ?>
__FUNCTION__
Returns the name of the current function:
<?php function mySampleFunc() { echo "the name the function is: " . __FUNCTION__; } mySampleFunc(); //the name of function is: mySampleFunc ?>
__METHOD__
Represents the name of the current method:
<?php class Sample { public static function myMethod() { echo "the name of method is: " . __METHOD__; } } Sample::myMethod(); // the name of the method is: myMethod ?>
__NAMESPACE__
Returns the name of the current namespace:
<?php namespace MySampleNS; echo "the namespace is: " . __NAMESPACE__; // the name space is: MySampleNS ?>
Magic Method
The magic method provides a mechanism to link to special PHP behaviors. Unlike previous constants, their names are written in lowercase/camel letters using two leading underscores, such as __construct()
and __destruct()
. __construct()
is a magic method called by PHP to create instances of class objects. It can accept any number of parameters.
<?php class MySample { public function __construct($foo) { echo __CLASS__ . " constructor called with $foo."; } } $obj = new MySample(42); // MySample constructor called with 42 ?>
As the name implies, the __destruct()
method is called when an object is destroyed by a PHP garbage collector. It does not accept any parameters and is usually used to perform any cleaning operations that may be required, such as closing a database connection.
<?php class MySample { public function __destruct() { echo __CLASS__ . " destructor called."; } } $obj = new MySample; // MySample destructor called ?>
Our next magic method handles property overloading and provides a way to let PHP handle undefined (or unaccessible) properties and method calls. If the property is undefined (or inaccessible) and is called in the getter context, PHP calls the __get()
method. This method accepts a parameter, namely the name of the property. It should return a value that is considered the value of the property. The __set()
method is called for undefined properties in the setter context. It accepts two parameters, attribute name and value.
<?php echo "line number: " . __LINE__; // line number: 2 echo "line number: " . __LINE__; // line number: 3 echo "line number: " . __LINE__; // line number: 4 ?>
In the above example code, the property name is not defined in the class. I tried assigning the value "mysample" to it, and PHP calls the magic method __set()
. It takes "name" as the $prop
parameter and "Alireza" as the $value
, and I store the value in a private $myArray
array. The __get()
method works similarly; when I output $obj->name
, the __get()
method is called and "name" is passed in as the $prop
parameter. There are other magic methods that help us retrieve and check inaccessible member variables, which also appear in the sample code: __isset()
, __unset()
, and __toString()
. __isset()
and __unset()
are both triggered by functions with the same name (without underscore) in PHP. __isset()
Check if the property is set and accepts a parameter, which is the property we want to test. __unset()
Receives a parameter, namely the name of the property the program wants to unset. In many cases, it is useful to represent objects as strings, such as output to users or other processes. Typically, PHP represents them as IDs in memory, which is not good for us. The __toString()
method helps us represent objects as strings. This method will be triggered in any case where an object is used as a string, for example: echo "Hello $obj"
. It can also be called directly like any other ordinary public method, which is preferable to tricks like appending empty strings to cast.
Summary
Object-oriented programming can produce code that is easier to maintain and test. It helps us create better, more standard PHP code. In addition, it can take advantage of magic methods and constants provided by PHP.
Pictures from Stepan Kapl / Shutterstock
FAQs on PHP Magic Methods and Predefined Constants
What are the different types of magic methods in PHP?
The magic method in PHP is a special function that will automatically trigger when certain conditions are met. They always start with a double underscore (). Different types of magic methods in PHP include `construct()、
destruct()、
call()、
callStatic()、
get()、
set()、
isset()、
unset ()、
sleep()、
wakeup()、
toString()、
invoke()、
set_state()、
clone()和
debugInfo()`. Each of these methods is triggered by a specific event, such as when creating an object, accessing a property, or calling a method.
How to use predefined constants in PHP?
Predefined constants in PHP are always built-in constants available. They include core constants such as PHP_VERSION
and PHP_OS
, as well as many other constants defined by various extensions. To use a predefined constant, just write its name without precluding the dollar sign ($) in it. For example, to get the current version of PHP, you can use the PHP_VERSION
constant, as shown below: echo PHP_VERSION;
What is the purpose of the magic method in PHP? __construct()
The magic method in PHP is automatically called every time a new object is created from a class. It is usually used to initialize the properties of an object or to perform any settings required by the object before use. __construct()
Yes, you can define your own constants in PHP using the
function or the define()
keyword. Once a constant is defined, it cannot be changed or undefined. const
The main difference between magic methods and conventional methods in PHP is that magic methods are automatically triggered by certain events, while conventional methods need to be called explicitly. Also, the magic method always starts with a double underscore (__), while the conventional method is not.
How to check whether constants are defined in PHP?
You can use the
function to check whether constants are defined in PHP. This function takes the name of the constant as a string, and returns true if the constant is defined, otherwise returns false. defined()
__destruct()
Magic method in PHP is automatically called when the object is destroyed or the script ends. It is often used to perform cleanup tasks, such as closing a database connection or freeing resources.
__destruct()
Can I use magic methods with static methods in PHP?
Yes, you can use magic methods with static methods in PHP.
Magic methods are automatically fired when calling static methods that are inaccessible or do not exist in the class.
__callStatic()
What are some common uses of predefined constants in PHP?
Predefined constants in PHP are usually used to obtain information about the PHP environment or to control the behavior of certain functions. For example, the
constant can be used to check the PHP version, and the constant can be used to check the operating system. PHP_VERSION
PHP_OS
Can I rewrite the magic method in PHP?
Yes, you can rewrite magic methods in PHP. When creating a subclass, you can provide your own magic method implementation. However, if you want to call the implementation of the parent class, you can use the
keyword.The above is the detailed content of Magic Methods and Predefined Constants in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


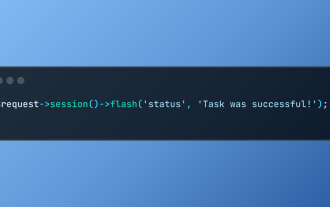
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
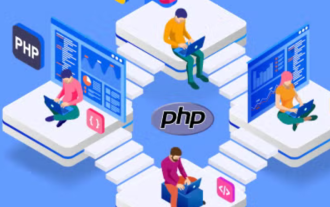
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
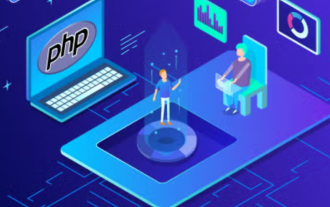
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
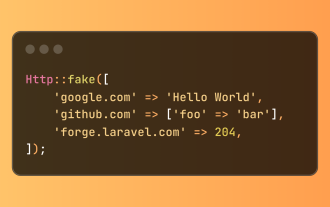
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
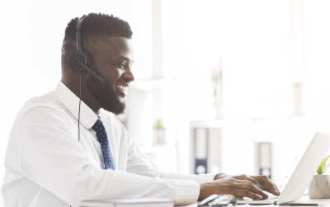
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
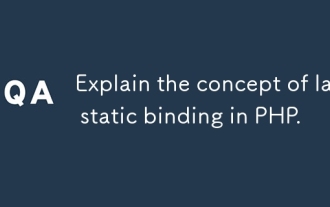
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
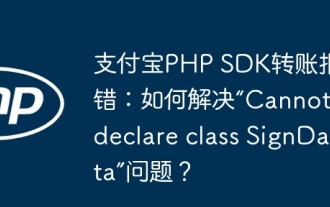
Alipay PHP...
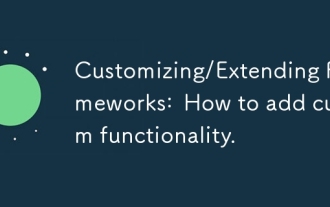
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
