An Introduction to Python's Flask Framework
This tutorial demonstrates building a simple two-page website using Flask, a lightweight Python web framework. It focuses on static content to establish a foundational workflow, easily expandable for more complex applications.
Flask Installation
Before starting, install Flask. If you encounter issues, consult online resources or leave a comment detailing the problem.
Virtualenv Setup
We'll use virtualenv to create an isolated Python environment for this project. This prevents conflicts with other system libraries.
Check if virtualenv is already installed:
$ virtualenv --version
If not, install it:
$ pip install virtualenv
Create and activate a virtual environment:
$ virtualenv flaskapp $ cd flaskapp $ . bin/activate
Now install Flask:
pip install Flask
Project Structure
Organize your project as follows within the flaskapp
directory:
<code>flaskapp/ ├── app/ │ ├── static/ │ │ ├── css/ │ │ ├── img/ │ │ └── js/ │ ├── templates/ │ ├── routes.py │ └── README.md └── ...</code>
The diagram below illustrates the application flow:
- A user request (e.g.,
/
) reaches theroutes.py
file. routes.py
locates the corresponding template in thetemplates
folder.- The template accesses static assets (images, CSS, JavaScript) from the
static
folder. - The rendered HTML is returned to the browser via
routes.py
.
Creating the Home Page
To avoid repetitive HTML boilerplate, we'll use web templates. Flask utilizes the Jinja2 template engine.
First, create a base layout template:
app/templates/layout.html
<!DOCTYPE html> <html> <head> <title>Flask App</title> <link href="{{ url_for('static', filename='css/main.css') }}" rel="stylesheet"> </head> <body> <div class="container"> <h1 id="Flask-App">Flask App</h1> </div> <div class="container"> {% block content %}{% endblock %} </div> </body> </html>
Next, create the home page template:
app/templates/home.html
{% extends "layout.html" %} {% block content %} <div class="jumbo"> <h2 id="Welcome">Welcome!</h2> <h3 id="This-is-the-home-page">This is the home page.</h3> </div> {% endblock %}
Now, map the URL to the template in routes.py
:
app/routes.py
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def home(): return render_template('home.html') if __name__ == '__main__': app.run(debug=True)
Add CSS styling to static/css/main.css
: (Content of main.css remains the same)
Running the app and visiting http://localhost:5000/
will display the home page.
Adding an About Page and Navigation
Let's create an "About" page and add navigation links.
Create the "About" template:
app/templates/about.html
{% extends "layout.html" %} {% block content %} <h2 id="About">About</h2> <p>This is the About page.</p> {% endblock %}
Update routes.py
to include the about page route:
app/routes.py
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def home(): return render_template('home.html') @app.route('/about') def about(): return render_template('about.html') if __name__ == '__main__': app.run(debug=True)
Add navigation links to layout.html
: (Content remains the same)
Add navigation styles to main.css
: (Content remains the same)
Now, you can access the about page at http://localhost:5000/about
.
Conclusion
This tutorial demonstrates a basic Flask application, illustrating a scalable workflow for building more complex web applications. Flask's simplicity and power make it an excellent choice for various web development projects.
The above is the detailed content of An Introduction to Python's Flask Framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


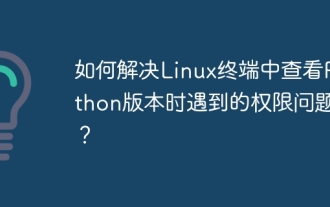
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
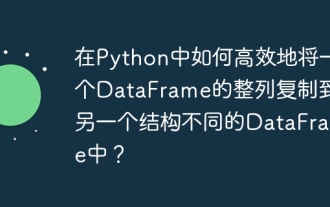
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
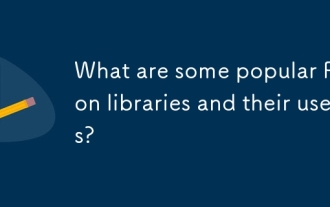
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
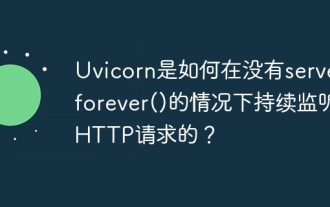
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
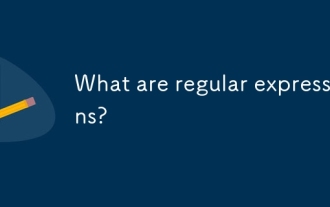
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
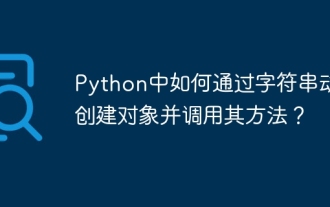
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
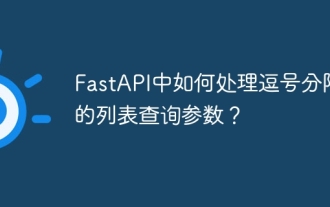
Fastapi ...
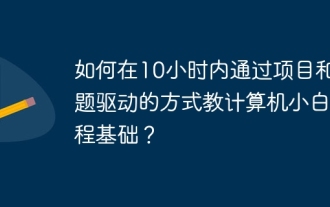
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
