jquery add image to browser cache
This guide demonstrates how to preload images into the browser cache using jQuery, improving website performance by reducing load times. The images are added to a hidden div element within the DOM.
(function($,D,W) { var JQUERY4U = {}; JQUERY4U.UTIL = { images: { loadingImage: '<img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/174076317693190.gif" class="lazy" alt="jquery add image to browser cache " /></img>', ajaxImage: '<img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/174076317789628.gif" class="lazy" alt="jquery add image to browser cache " /></img>', savingImage: '<img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/174076317761887.gif" class="lazy" alt="jquery add image to browser cache " /></img>' }, preloadImages: function() { $('body').append('<div id="img-cache" style="display:none"></div>'); $.each(JQUERY4U.UTIL.images, function(i,v) { $('#img-cache').append(v); }); } } $(D).ready(function($) { JQUERY4U.UTIL.preloadImages(); }); })(jQuery,document,window);
This code creates a hidden div
with the ID "img-cache" and appends image elements to it. The $.each
loop iterates through the images
object, adding each image to the cache.
Alternative Approach:
A simpler method for preloading images without a hidden div:
// Array of image URLs var imageArray = ['image1.jpeg', 'image2.png', 'image3.gif']; // Preload images $.each(imageArray, function(index, src) { new Image().src = src; });
This approach directly creates Image
objects, assigning the src
attribute. The browser will then fetch and cache these images without needing to add them to the DOM.
Frequently Asked Questions
This section answers common questions about adding images to a webpage using jQuery.
Adding Images to a Div with jQuery
Use the append()
method to add an image to a div:
$('#myDiv').append('<img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/174076317883720.png" class="lazy" alt="jquery add image to browser cache " />');
Loading and Appending Images with JavaScript
JavaScript's createElement()
and appendChild()
methods achieve the same result:
var img = document.createElement('img'); img.src = 'image.png'; document.getElementById('myDiv').appendChild(img);
Dynamically Appending Images with jQuery's append()
Dynamically add images using variables:
var imagePath = 'image.png'; var altText = 'My Image'; $('#myDiv').append('<img src="' + imagePath + '" alt="' + altText + '">');
Appending Images with Variable IDs via AJAX and jQuery
AJAX allows for dynamic image loading and appending:
$.ajax({ url: '/get-image-path', // Endpoint to fetch image path success: function(data) { $('#myDiv').append('<img src="' + data.imagePath + '" id="dynamicImg' + data.imageId + '" alt="Dynamic Image">'); } });
Appending Images via AJAX in Laravel
In Laravel, use the asset()
helper within the AJAX success callback:
$.ajax({ url: '/get-image', success: function(data) { $('#myDiv').append('<img src="' + "{{ asset('" + data.image + "') }}" + '" alt="Laravel Image">'); } }); ``` Remember to adjust paths and endpoints to match your specific project setup.
The above is the detailed content of jquery add image to browser cache. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










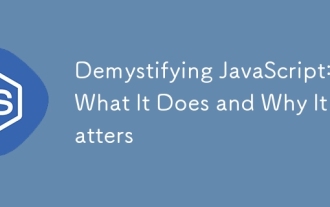
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
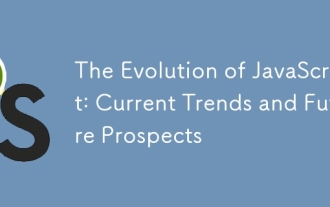
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
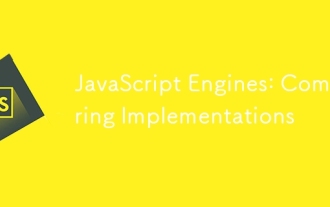
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
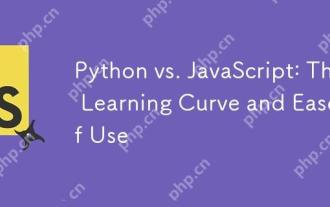
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
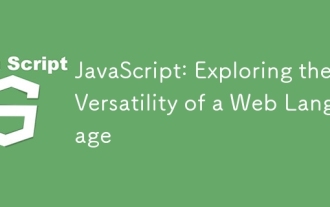
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
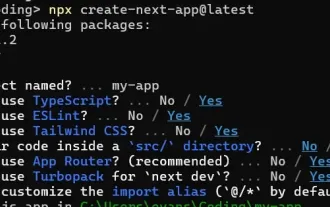
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
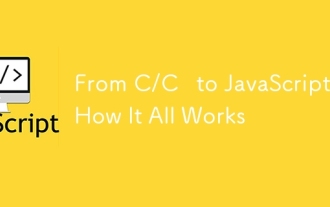
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
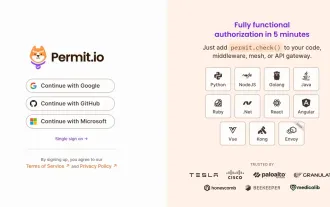
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
