jQuery Check if Date is Valid
Simple JavaScript function is used to check if the date is valid.
function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] + '/' + bits[1] + '/' + bits[0]); return !!(d && (d.getMonth() + 1) == bits[1] && d.getDate() == Number(bits[0])); } //测试 var currentDate = new Date('31/09/2011'); console.log(isValidDate(currentDate.toString())); console.log(isValidDate('30/09/2011'));
JavaScript function is used to get the number of days in a month.
function daysInMonth(month, year) { return new Date(year, month, 0).getDate(); } //测试 daysInMonth('09','2011'); //输出: 30
See also:- jQuery Get future date
- JavaScript date displays year error
- jQuery date and time function - complete list
JavaScript function is used to check if the date is valid and if it is invalid, it will be set to the last day of the month.
/* 检查日期是否有效,如果无效则恢复到当月最后一天 */ validateDateLastDayMonth: function() { /* 辅助函数 */ function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] + '/' + bits[1] + '/' + bits[0]); return !!(d && (d.getMonth() + 1) == bits[1] && d.getDate() == Number(bits[0])); } function daysInMonth(month, year) { return new Date(year, month, 0).getDate(); } /* 初始化 */ var currentDate = new Date(), currentMonth = currentDate.getMonth() + 1, lastDayOfMonth = new Date(currentDate.getFullYear(), (currentDate.getMonth() - 1), 0).getDate(), departureDate = FCL.DATETIME.futureDateDays(14), depDate = departureDate.split('/'), departureDateMonth = depDate[1]; if (departureDateMonth != currentMonth) { departureDate = FCL.DATETIME.leadingZero(lastDayOfMonth) +'/'+ FCL.DATETIME.leadingZero(currentMonth) +'/'+ depDate[2]; } /* 验证日期 */ if (!isValidDate(departureDate.toString())) { var bits = departureDate.split('/'); departureDate = FCL.DATETIME.leadingZero(daysInMonth(bits[1],bits[2])) +'/'+ FCL.DATETIME.leadingZero(currentMonth) +'/'+ depDate[2]; } $('input[name="depDate"]').val(departureDate); }
jQuery date verification FAQs (FAQs)
How to use jQuery to verify dates in a specific format?
jQuery provides a method called $.datepicker.parseDate
that can be used to verify dates in a specific format. This method takes two parameters: date format and date string. If the date string matches the format, the method returns a Date object. If it does not match, an error will be raised. Examples are as follows:
try { $.datepicker.parseDate('dd/mm/yy', '31/12/2020'); console.log('日期有效'); } catch (error) { console.log('日期无效'); }
What is a jQuery verification plugin and how do you use it for date verification?
jQuery Verification Plugin provides a powerful client form verification framework. It includes built-in verification methods for common needs including date verification. To use it, you need to include the plugin in your project and call the validate
method on the form. You can specify rules for each form field, including date fields. For example:
$('form').validate({ rules: { dateField: { date: true } } });
How to use jQuery to verify date range?
To verify the date range, you can use the $.datepicker.parseDate
method to convert the date string to a Date object and then compare. Examples are as follows:
var startDate = $.datepicker.parseDate('dd/mm/yy', '01/01/2020'); var endDate = $.datepicker.parseDate('dd/mm/yy', '31/12/2020'); if (startDate > endDate) { console.log('开始日期晚于结束日期'); } else { console.log('日期范围有效'); }
Can I use jQuery to verify the date without using the plugin?
Yes, you can use the built-in JavaScript Date object to verify dates without using plug-ins. However, this method is not as flexible and more complex as using plug-ins or $.datepicker.parseDate
. Examples are as follows:
var dateString = '31/12/2020'; var dateParts = dateString.split('/'); var dateObject = new Date(+dateParts[2], dateParts[1] - 1, +dateParts[0]); if (dateObject.getDate() == dateParts[0] && dateObject.getMonth() == dateParts[1] - 1 && dateObject.getFullYear() == dateParts[2]) { console.log('日期有效'); } else { console.log('日期无效'); }
The rest of the FAQ content is similar to the above, but the description of the problem and the code example have been slightly adjusted. In order to avoid duplication, I will not repeat it here. The core idea is to verify the validity of dates based on JavaScript's Date
object and jQuery's $.datepicker.parseDate
method. Different problems only differ in verification conditions, such as date format, date range, leap year, etc.
The above is the detailed content of jQuery Check if Date is Valid. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










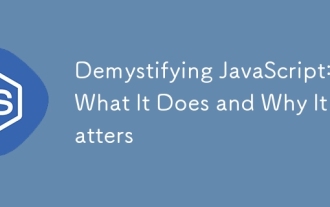
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
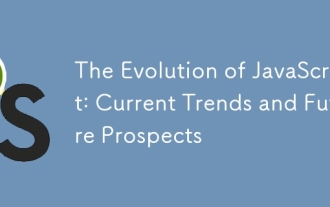
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
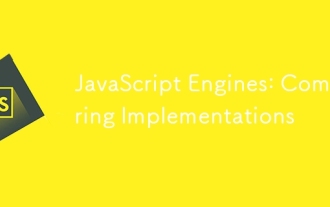
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
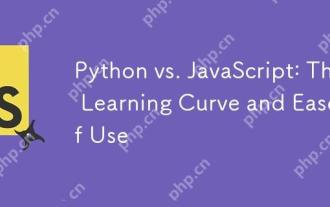
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
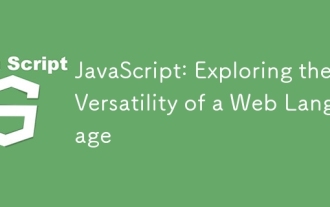
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
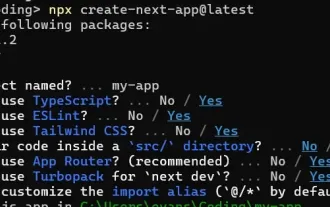
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
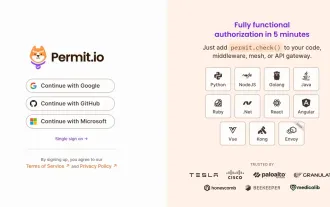
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
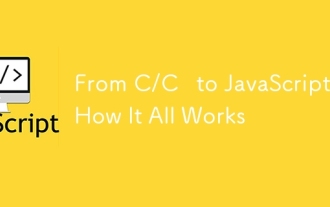
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
