jQuery get element padding/margin
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky.
// 设置 $("div.header").css("margin","10px"); $("div.header").css("padding","10px");
You might think this code is simple, but it doesn't work.
// 获取 $("div.header").css("margin"); $("div.header").css("padding");
Try it yourself (paste the following code into Firebug). You will find that the returned margin and padding values are empty.
(function($){ function logMarginPadding(elem) { // 获取 var margin = elem.css("margin"), padding = elem.css("padding"); console.log("margin="+margin+" padding="+padding); } var elem = $("div.header"); // 设置要检查的元素 logMarginPadding(elem); // 设置 elem.css("margin","10px"); elem.css("padding","10px"); logMarginPadding(elem); })(jQuery);
This code seems to work.
// 获取元素的顶部外边距 alert($("div.header").css("margin-top")); // 获取元素的顶部外边距(整数) alert($("a").css("margin-top").replace("px", "")); // 如果与计算一起使用,则必须将其转换为整数 parseInt($("a").css('padding-left').replace("px", ""));
Anyway, this is how I get the margins inside the element. This approach is not ideal and only works if the inner margins are even (the same on each side). Just get the width and outer width and divide by 2.
var item = $('div.header'); width = item.width(), padding = (item.outerWidth()-width)/2;
A popular plugin called jsizes might be a good solution to get/set the inner/outside margin values of the element. JSizes is a small plugin for the jQuery JavaScript library that adds support for querying and setting CSS min-width
, min-height
, max-width
, max-height
, border-*-width
, margin
, padding
,
, , and properties.
outerWidth
Further readingouterHeight
jQuery has and functions, which by default contain borders and inner margins, and if the first parameter of the function is true, it also contains outer margins.
https://www.php.cn/link/a47ad724599e11d59272b02d08d0dbd7
jQuery Element Inner/Margin FAQ (FAQ)css()
var padding = $("div").css("padding");
You can use the
method of jQuery to get the inner margin of an element. This method returns the calculated style attribute value. For example, to get the inner margin of a div element, you can use the following code:This will return the inner margin value of the div element in pixels. css()
$("div").css("margin", "20px");
You can use the
method of jQuery to set the margin of an element. This method sets one or more style properties of the selected element. Here is an example of how to set the margins of a div element:
outerWidth()
outerHeight()
This will set the margin of the div element to 20 pixels.
What is the difference between and outerWidth()
in outerHeight()
?
How to use jQuery to get the size of an element?
jQuery provides several ways to get the size of an element. These methods include width()
, height()
, innerWidth()
, innerHeight()
, outerWidth()
, outerHeight()
and
How to change the size of an element using jQuery?
width()
You can change the size of an element in jQuery using the height()
, innerWidth()
, innerHeight()
, outerWidth()
, outerHeight()
,
methods. These methods not only return the corresponding dimensions of the selected element, but also set a new dimension when passing the value as a parameter. innerWidth()
What is the difference between outerWidth()
and
in ? innerWidth()
outerWidth()
The
method returns the width of the element, including the inner margin and border. If the optional parameter is set to true, margins are also included.
How to use jQuery to get the border width of an element? css()
// 设置 $("div.header").css("margin","10px"); $("div.header").css("padding","10px");
This will return the border width of the div element in pixels.
How to set the inner and outer margins of an element using jQuery at the same time? css()
// 获取 $("div.header").css("margin"); $("div.header").css("padding");
This will set the inner and outer margins of the div element to 10 pixels and 20 pixels respectively.
How to use jQuery to get the margin of an element? css()
(function($){ function logMarginPadding(elem) { // 获取 var margin = elem.css("margin"), padding = elem.css("padding"); console.log("margin="+margin+" padding="+padding); } var elem = $("div.header"); // 设置要检查的元素 logMarginPadding(elem); // 设置 elem.css("margin","10px"); elem.css("padding","10px"); logMarginPadding(elem); })(jQuery);
This will return the margin value of the div element in pixels.
How to set the border width of an element using jQuery? css()
// 获取元素的顶部外边距 alert($("div.header").css("margin-top")); // 获取元素的顶部外边距(整数) alert($("a").css("margin-top").replace("px", "")); // 如果与计算一起使用,则必须将其转换为整数 parseInt($("a").css('padding-left').replace("px", ""));
This will set the border width of the div element to 2 pixels.
The above is the detailed content of jQuery get element padding/margin. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










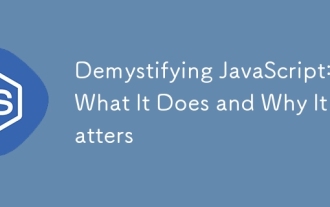
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
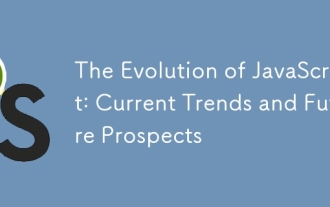
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
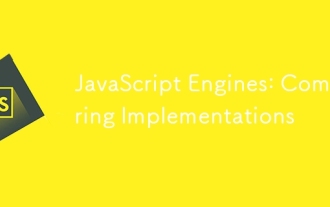
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
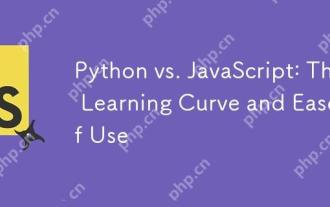
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
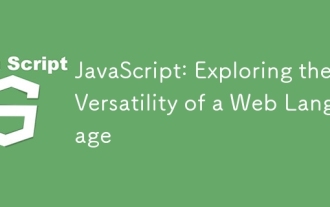
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
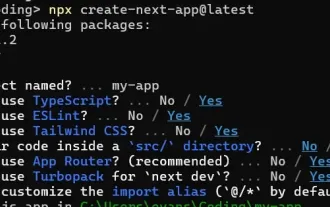
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
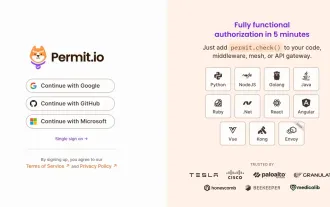
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
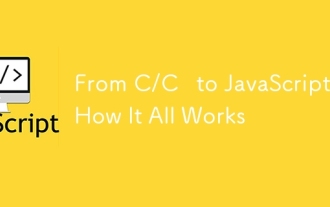
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
