Set Up Caching in PHP With the Symfony Cache Component
This tutorial introduces the Symfony Cache component, a straightforward method for integrating caching into your PHP applications. Caching significantly enhances application performance by reducing page load times.
The Symfony Cache Component: A Deep Dive
The Symfony Cache component simplifies caching in PHP applications. Its ease of installation and configuration allows for rapid implementation. It offers a range of adapters, including:
- Database adapter
- Filesystem adapter
- Memcached adapter
- Redis adapter
- APCu adapter
- And more
Understanding the Symfony Cache component involves familiarity with two key approaches:
PSR-6 Caching: A Key-Value Approach
This generic caching system utilizes cache pools and cache items. A cache item represents the stored content (a key-value pair). The cache pool logically groups these items and manages them. The cache adapter handles the underlying storage in the chosen back-end.
Cache Contracts: Callback-Based Caching
This approach, while simpler, offers more power through recomputation callbacks and built-in stampede prevention. It's the recommended method due to its concise code.
This tutorial covers both approaches, starting with installation and configuration, then demonstrating practical examples.
Installation and Configuration: Getting Started
Assuming you have Composer installed, use this command to install the Cache component:
composer require symfony/cache
This generates a composer.json
file (or updates it):
{ "require": { "symfony/cache": "^4.1" } }
Finally, include the Composer-generated autoload.php
in your application:
<?php require_once './vendor/autoload.php'; // Application code ?>
PSR-6 Caching: A Practical Example
This example illustrates PSR-6 caching:
<?php require_once './vendor/autoload.php'; use Symfony\Component\Cache\Adapter\FilesystemAdapter; $cachePool = new FilesystemAdapter(); // Store string values $demoString = $cachePool->getItem('demo_string'); if (!$demoString->isHit()) { $demoString->set('Hello World!'); $cachePool->save($demoString); } if ($cachePool->hasItem('demo_string')) { $demoString = $cachePool->getItem('demo_string'); echo $demoString->get(); echo "<br>"; } // ... (rest of the code remains the same)
This code demonstrates creating a cache pool, storing and retrieving string and array values, deleting items, and setting expiration times. The comments within the original code provide detailed explanations of each section.
Cache Contracts: A Concise Alternative (Details omitted for brevity)
The original article details using Cache Contracts; however, due to space constraints, a detailed explanation is omitted here. The core concept involves using callbacks for value generation, minimizing code compared to the PSR-6 approach.
Conclusion
The Symfony Cache component offers a flexible and efficient way to implement caching in PHP applications. Its diverse adapter support and straightforward API make it a valuable tool for performance optimization. The choice between PSR-6 and Cache Contracts depends on project needs and coding style.
(Post thumbnail generated by OpenAI DALL-E)
The above is the detailed content of Set Up Caching in PHP With the Symfony Cache Component. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


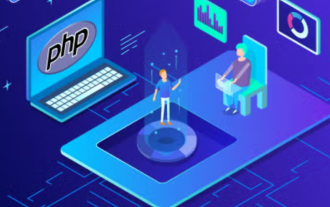
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
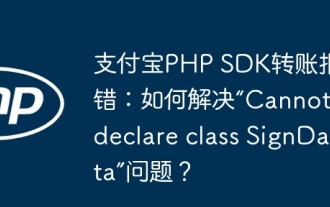
Alipay PHP...
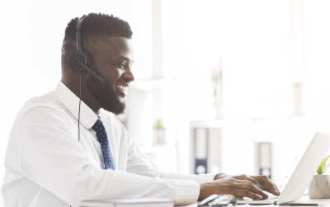
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
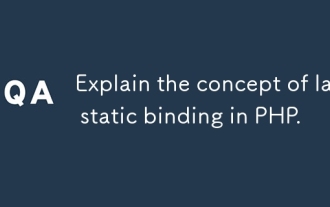
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
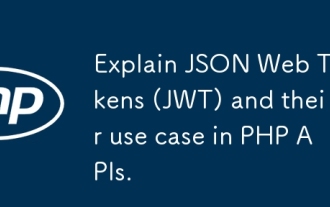
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
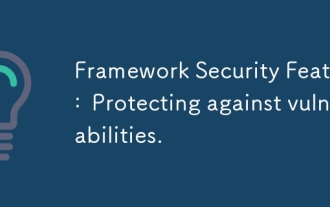
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
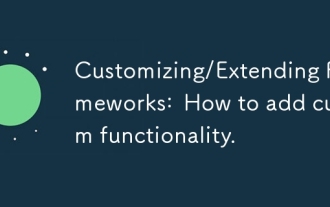
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
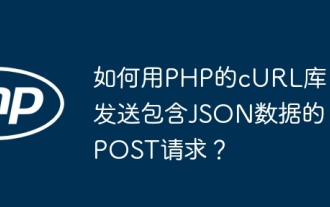
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
