How to Cache Using Redis in Django Applications
This tutorial demonstrates how to leverage Redis caching to boost the performance of Python applications, specifically within a Django framework. We'll cover Redis installation, Django configuration, and performance comparisons to highlight the benefits of caching.
Introduction to Redis and Caching
Caching significantly improves application speed by storing frequently accessed data in a readily available location (the cache) rather than repeatedly querying slower data sources like databases. Redis, an open-source, in-memory data structure store, excels as a database, cache, and message broker. It dramatically reduces database load by serving data directly from its cache.
Installing Redis
For Ubuntu users, the simplest installation involves these commands:
sudo apt-get update sudo apt install redis
Verify the installation with:
redis-cli --version
Windows users can utilize the Windows Subsystem for Linux (WSL2). First, enable WSL2 (run as administrator):
Enable-WindowsOptionalFeature -Online -FeatureName Microsoft-Windows-Subsystem-Linux
Install Ubuntu from the Microsoft Store, then launch the Ubuntu terminal and execute:
sudo apt-add-repository ppa:redislabs/redis sudo apt-get update sudo apt-get upgrade sudo apt-get install redis-server sudo service redis-server restart
Django API Example: Caching Product Data
This example demonstrates caching product data in a Django application. We'll use django-redis
to interact with Redis.
Prerequisites:
- Django
django-redis
- Redis
-
loadtest
(for performance testing)
Project Setup:
- Create a project directory and virtual environment.
- Activate the virtual environment and install dependencies:
pip install django==1.9 django-redis djangorestframework
- Create a Django project and app:
django-admin startproject django_cache cd django_cache python manage.py startapp store
-
Add
store
andrest_framework
toINSTALLED_APPS
insettings.py
. -
Create the
Product
model instore/models.py
:
from django.db import models class Product(models.Model): name = models.CharField(max_length=255) description = models.TextField(null=True, blank=True) price = models.IntegerField(null=True, blank=True) date_created = models.DateTimeField(auto_now_add=True, blank=True) date_modified = models.DateTimeField(auto_now=True, blank=True) def __str__(self): return self.name def to_json(self): return { 'id': self.id, 'name': self.name, 'desc': self.description, 'price': self.price, 'date_created': self.date_created, 'date_modified': self.date_modified }
- Run migrations:
python manage.py makemigrations store python manage.py migrate
- Create a superuser and populate the database with sample data.
Configuring Redis in Django:
Add the following to settings.py
:
CACHES = { 'default': { 'BACKEND': 'django_redis.cache.RedisCache', 'LOCATION': 'redis://127.0.0.1:6379/', 'OPTIONS': { 'CLIENT_CLASS': 'django_redis.client.DefaultClient', } } }
Creating Views and URLs:
Create store/views.py
with endpoints for retrieving products (with and without caching):
from rest_framework.decorators import api_view from rest_framework.response import Response from django.core.cache import cache from .models import Product @api_view(['GET']) def view_products(request): products = Product.objects.all() results = [p.to_json() for p in products] return Response(results) @api_view(['GET']) def view_cached_products(request): products = cache.get('products') if products: return Response(products) else: products = Product.objects.all() results = [p.to_json() for p in products] cache.set('products', results) return Response(results)
Create store/urls.py
:
from django.urls import path from . import views urlpatterns = [ path('', views.view_products), path('cached/', views.view_cached_products), ]
Include store/urls
in your main urls.py
.
Performance Testing with loadtest
:
Install loadtest
: sudo npm install -g loadtest
Run tests for both endpoints to compare performance. The cached endpoint should show significantly improved requests per second after the initial cache population.
Conclusion:
This tutorial illustrates the straightforward integration of Redis caching into a Django application, resulting in substantial performance gains. Redis's in-memory nature and ease of use make it an excellent choice for improving application speed and reducing server load. Remember to consider caching strategies to optimize your application's performance and resource utilization.
(Post thumbnail image generated by OpenAI DALL-E.)
The above is the detailed content of How to Cache Using Redis in Django Applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










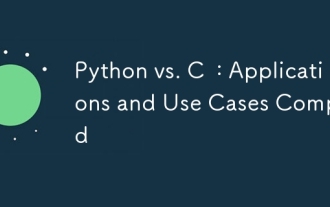
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
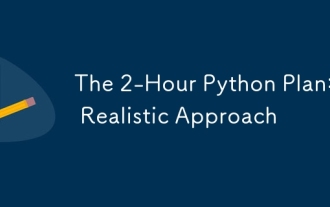
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
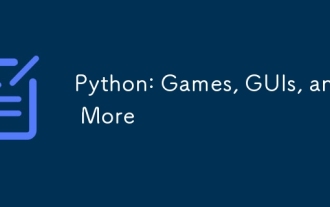
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
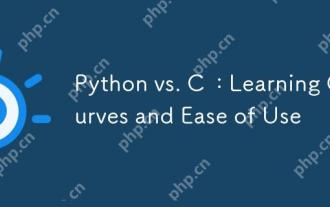
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
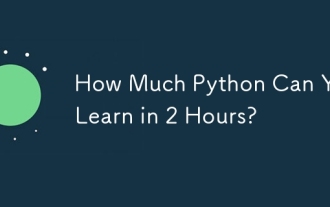
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
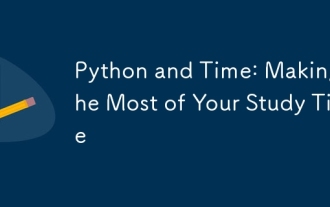
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
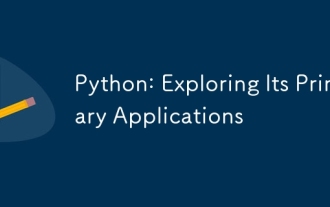
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
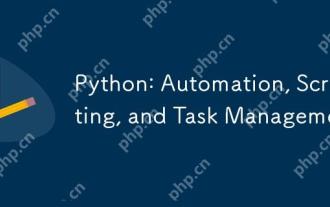
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
