phpmaster | Using PHP Regular Expressions
Core points
- Regular expressions (also known as regex) are patterns used to match text in strings. They are especially useful when you need to find text for different instances in a string.
- Regular expression notation uses special characters and symbols to define patterns. The "^" symbol specifies that the match must start at the beginning of the line, while " " is a quantifier that means that "at least one" of the previous character or collection must be matched.
- PHP uses functions such as
preg_match()
,preg_replace()
andpreg_match_all()
to apply regular expressions. These functions can verify form fields, format text, and extract information arrays from strings, respectively. - Metacharacters, quantifiers and separators play a crucial role in regular expression patterns. Understanding their capabilities can help you create more complex and precise search patterns.
^[A-Za-z0-9-_.+%]+@[A-Za-z0-9-.]+.[A-Za-z]{2,4}$
This code looks as hard to understand as ancient Egyptian hieroglyphs, but it is actually a regular expression pattern for matching email addresses such as oleomarg32@hotmail.com
, Fiery.Rebel@veneuser.info
, robustlamp selfmag@gmail.ca
, etc. This article will introduce the basics of regular expressions and their application in PHP.
Beginner of regular expression notation
Let's break down the above example one by one: ^[A-Za-z0-9-_. %] @[A-Za-z0-9-.] .[A-Za-z]{2,4}$
-
^
: Match the beginning of the string. -
[A-Za-z0-9-_. %]
: Match one or more letters, numbers, or special characters (-_. %). Square brackets[]
define character sets. -
@
: Literally match the "@" symbol. -
[A-Za-z0-9-.]
: Match one or more letters, numbers, or dots (.). -
.
: Literal match "." symbol (escaped). -
[A-Za-z]{2,4}
: Match 2 to 4 letters. Braces{}
Specifies the number of repetitions. -
$
: Match the end of the string.
If you replace the first
quantifier with *
, for example:
^[A-Za-z0-9-_.+%]*@[A-Za-z0-9-.]+.[A-Za-z]{2,4}
can match strings like @SodaCanDrive.com
because the *
quantifier means "zero or more".
Regular expressions in PHP
After understanding regular expression notation, let's see how to use it in PHP. PHP provides several functions to handle regular expressions: preg_match()
, preg_replace()
, and preg_match_all()
.
preg_match()
preg_match()
Used to check if there is a matching pattern in the string. Returns 1 if a match is found, otherwise returns 0.
<?php if (preg_match('/^[A-Za-z0-9-_.+%]+@[A-Za-z0-9-.]+.[A-Za-z]{2,4}$/', $_POST["emailAddy"])) { echo "Email address accepted"; } else { echo "Email address is all broke."; } ?>
Note that regular expressions are wrapped with slash /
as delimiter.
preg_replace()
preg_replace()
Used to find and replace matching patterns.
^[A-Za-z0-9-_.+%]+@[A-Za-z0-9-.]+.[A-Za-z]{2,4}$
U
modifier makes regular expressions match non-greedy. The brackets ()
are used to capture the matching text, and 1
is a backreference that refers to the first captured group.
preg_match_all()
preg_match_all()
Used to find all matching patterns in a string and store the results into an array.
^[A-Za-z0-9-_.+%]*@[A-Za-z0-9-.]+.[A-Za-z]{2,4}
i
modifier means case insensitive.
This article only introduces the basics of regular expressions, more advanced usages, such as prospects, backsights and more complex backreferences, please refer to the official PHP documentation.
Pictures from Boris Mrdja / Shutterstock
The above is the detailed content of phpmaster | Using PHP Regular Expressions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


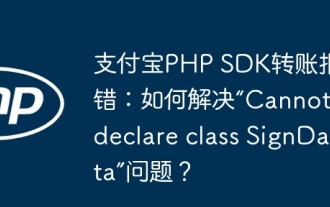
Alipay PHP...
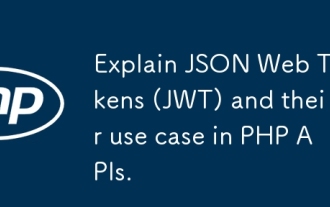
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
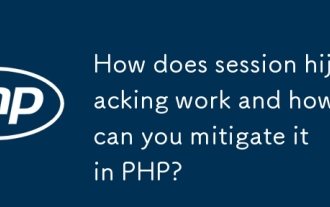
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
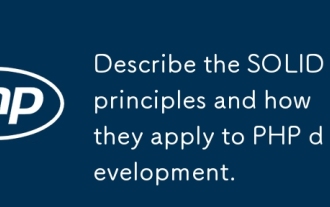
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
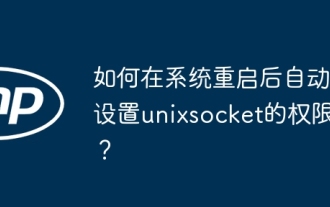
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
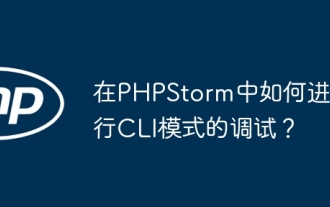
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
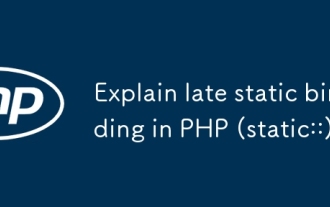
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
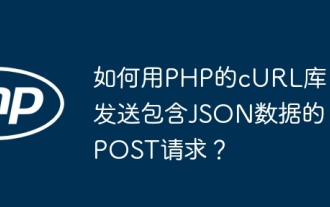
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
