How to dynamically add methods to objects in Python?
Adding Methods to Python Objects: A Comprehensive Guide
This article answers four key questions regarding the dynamic addition of methods to Python objects. We'll explore various approaches and best practices.
How to Dynamically Add Methods to a Python Object?
In Python, you can add methods to an object at runtime without modifying its class definition. This is achieved primarily through using the setattr()
function. setattr()
allows you to dynamically add attributes to an object, including methods. A method is simply a function that's attached to an object as an attribute.
Here's an example:
class MyClass: def __init__(self, name): self.name = name obj = MyClass("Example") def new_method(self): print(f"Hello from the dynamically added method! My name is {self.name}") setattr(obj, 'dynamic_method', new_method) obj.dynamic_method() # Output: Hello from the dynamically added method! My name is Example
In this code, we define a function new_method
. setattr(obj, 'dynamic_method', new_method)
binds this function to the obj
instance under the name dynamic_method
. Now obj
behaves as if it had a method named dynamic_method
. Crucially, this doesn't alter the MyClass
class itself; the method is only added to the specific instance obj
.
How Can I Add a Method to a Python Class Instance at Runtime?
The answer is the same as the previous question. Using setattr()
is the primary way to achieve this. The key is understanding that adding a method to an instance doesn't change the class definition; the method is added only to that specific instance.
Let's illustrate with a slightly different example:
class Dog: def __init__(self, name): self.name = name my_dog = Dog("Buddy") def fetch(self, item): print(f"{self.name} fetched the {item}!") setattr(my_dog, "fetch", fetch) my_dog.fetch("ball") # Output: Buddy fetched the ball!
Here, the fetch
method is added only to my_dog
, not to all instances of the Dog
class. Another Dog
instance created later wouldn't have the fetch
method.
What Are the Best Practices for Dynamically Adding Methods to Python Objects?
While dynamic method addition is powerful, it should be used judiciously. Overusing it can lead to code that's harder to understand and maintain. Here are some best practices:
- Minimize its use: Prefer defining methods within the class definition whenever possible. This improves readability and maintainability. Dynamic addition should be reserved for situations where the need for a method arises only at runtime and is not known beforehand.
- Clear naming conventions: Use descriptive names for dynamically added methods to avoid confusion.
- Careful consideration of side effects: Ensure that dynamically added methods don't unexpectedly interact with existing methods or attributes.
- Documentation: Clearly document why and how dynamic method addition is used in your code.
- Testing: Thoroughly test any code that uses dynamic method addition to prevent unexpected behavior.
Is It Possible to Add Methods to a Python Object Without Modifying Its Class Definition?
Yes, absolutely. As demonstrated throughout this article, setattr()
allows you to add methods to an instance of a class without altering the class's definition. This is a key feature of Python's dynamic nature. The added method is specific to the instance and doesn't become part of the class's blueprint. This approach preserves the original class definition, keeping it clean and predictable.
The above is the detailed content of How to dynamically add methods to objects in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










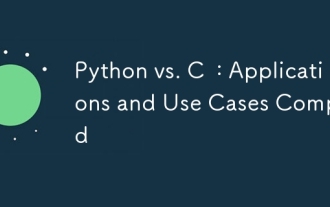
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
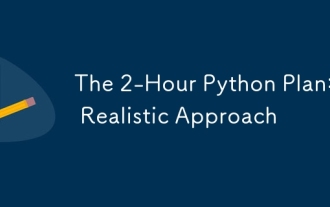
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
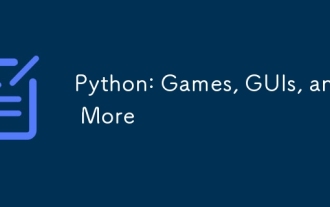
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
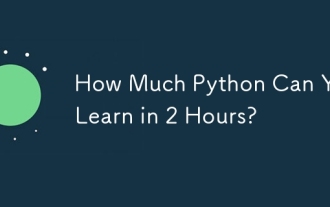
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
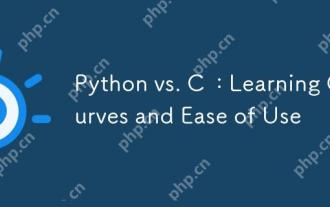
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
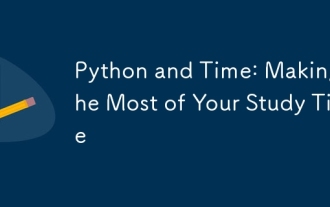
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
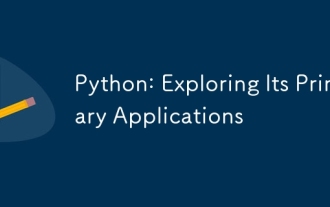
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
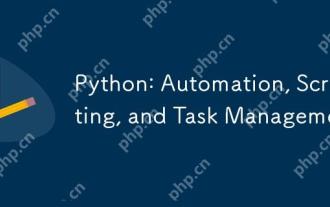
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
