


Is Django paging query inefficient? How to avoid full-table scanning of databases?
Django Pagination Query Efficiency is Low? How to Avoid Full Table Scans?
Django's built-in pagination, while convenient, can lead to performance issues with large datasets if not implemented carefully. The primary culprit is the potential for full table scans. When you use Paginator
with a queryset that hasn't been optimized, Django might fetch all rows from the database before slicing them into pages. This is inefficient and drastically slows down the response time, especially with millions of records. To avoid full table scans, you must ensure that your database query only retrieves the necessary rows for the requested page. This involves using database-level pagination features, which means leveraging LIMIT
and OFFSET
clauses in your SQL query. Django's ORM provides ways to do this, most effectively through QuerySet.offset()
and QuerySet.limit()
, or by directly using raw SQL queries with appropriate LIMIT
and OFFSET
clauses if needed for complex scenarios. Properly indexed database columns are also crucial; without them, even limited queries can still be slow. Ensure you have indexes on columns frequently used in WHERE
clauses of your pagination queries.
What are the common causes of slow pagination in Django?
Several factors contribute to slow pagination in Django applications:
- Lack of Database Indexing: Without proper indexes on relevant columns, the database must perform a full table scan to locate the desired rows for each page. This is extremely slow for large datasets.
-
Inefficient QuerySets: Using
QuerySet
methods that force the evaluation of the entire queryset before pagination (e.g., iterating through the entire queryset before applying pagination) defeats the purpose of pagination and leads to performance bottlenecks. - N 1 Problem: If your pagination involves related models and you're not using prefetching or select_related, you'll end up making numerous additional database queries for each object on a page (one query per object to retrieve related data).
- Unoptimized Database Queries: Complex or poorly written queries that don't leverage database indexes effectively can significantly impact performance.
-
Improper Use of
Paginator
: UsingPaginator
without considering the underlying database query can lead to fetching the entire dataset before applying pagination, which is highly inefficient. -
Lack of Database-Level Pagination: Relying solely on Python-side pagination without using
LIMIT
andOFFSET
in the database query will result in fetching all data from the database before slicing it, negating the performance benefits of pagination. - Heavy Data Transfer: Transferring large amounts of data from the database to the application server even after pagination can still cause delays if not handled efficiently.
How can I optimize my Django models and queries for efficient pagination?
Optimizing Django models and queries for efficient pagination involves a multi-pronged approach:
-
Database Indexing: Create indexes on columns frequently used in
WHERE
clauses of your pagination queries, especially those involved in ordering. -
Efficient QuerySets: Use
QuerySet.order_by()
to define the sorting order for your data. UtilizeQuerySet.select_related()
andQuerySet.prefetch_related()
to reduce database queries when dealing with related models. Avoid unnecessaryQuerySet
operations that force early evaluation of the queryset. -
Database-Level Pagination: Employ
QuerySet.offset()
andQuerySet.limit()
methods to leverage the database's built-in pagination capabilities usingLIMIT
andOFFSET
clauses in the generated SQL. This ensures only the necessary data is retrieved. -
Raw SQL (if necessary): For complex pagination scenarios, consider using raw SQL queries with
LIMIT
andOFFSET
for fine-grained control over the database interaction. - Model Optimization: Ensure your models are appropriately designed and normalized to avoid redundancy and improve query efficiency.
- Caching: Implement caching strategies (e.g., using Django's caching framework or a dedicated caching solution like Redis) to store frequently accessed paginated data.
What are the best practices for implementing efficient pagination in Django with large datasets?
For efficient pagination with large datasets in Django, follow these best practices:
-
Always use database-level pagination: Never fetch the entire dataset into memory before paginating. Always use
LIMIT
andOFFSET
to retrieve only the data needed for the current page. - Optimize database queries: Ensure your queries are efficient and use appropriate indexes. Profile your queries to identify bottlenecks.
- Use appropriate data structures: Avoid unnecessary data transformation or manipulation after retrieving data from the database.
- Implement caching: Cache frequently accessed pages to reduce database load.
-
Consider alternative pagination strategies: For extremely large datasets, explore alternative pagination techniques like cursor-based pagination, which avoids the issues associated with
OFFSET
for very large offsets. Cursor-based pagination uses a unique identifier to fetch the next page, making it more efficient for very large datasets. - Use asynchronous tasks: For complex pagination logic or computationally intensive operations, offload the work to asynchronous tasks (e.g., using Celery) to prevent blocking the main thread.
- Monitor performance: Regularly monitor your application's performance to identify and address any emerging pagination issues. Use profiling tools to pinpoint slow queries and optimize them accordingly.
The above is the detailed content of Is Django paging query inefficient? How to avoid full-table scanning of databases?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


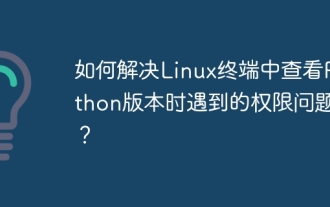
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
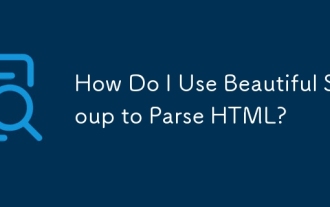
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
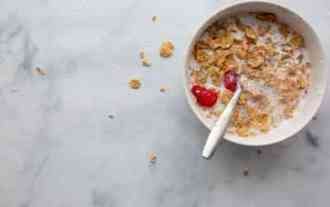
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
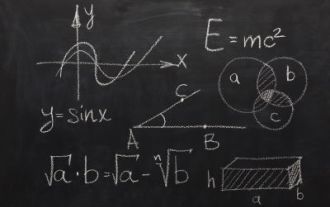
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
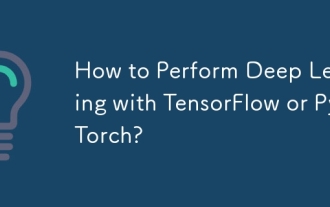
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap

This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
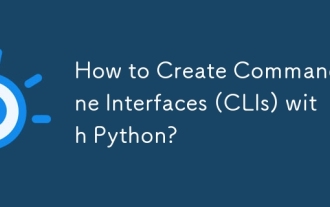
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
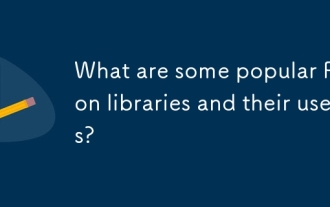
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
