


What is the function of underscore of import statements in Go language?
Go Language Import Statement Underscore _: What is its purpose?
The underscore _
in a Go import statement serves a crucial purpose: it allows you to import a package without actually using any of its exported identifiers within your current file. This is primarily used to avoid unused import warnings from the Go compiler. While the package is still loaded and potentially used by other parts of your program (e.g., if it has side effects like initializing global variables), your current file won't be flagged for having an unused import. This improves code cleanliness and readability by suppressing warnings that might otherwise be considered noise, particularly in larger projects. Essentially, it's a way to tell the Go compiler, "Yes, I know I'm importing this package, and it's necessary, even if I don't explicitly use any of its names in this specific file."
What does the underscore _
do when importing packages in Go?
When you use _
in a Go import statement (e.g., import _ "path/filepath"
), the Go compiler loads the specified package (path/filepath
in this example) but ignores any exported names it contains. This means you cannot directly access functions, constants, variables, or types defined within that package in the current file. However, the package's init()
function (if present) will still be executed. This is important because some packages perform crucial initialization tasks or register themselves with other parts of the system. For instance, some packages might register themselves with a global registry or set up necessary runtime configurations. Using _
allows you to leverage these initialization side effects without incurring the penalty of unused import warnings.
How can I avoid unused import warnings in Go using underscores?
The underscore _
provides a clean and straightforward way to suppress unused import warnings. If you have a package that you need to import for its side effects (initialization, for example), but you don't actually use any of its exported entities in the current file, simply prefix the package name with an underscore in your import statement.
For example:
import ( "fmt" _ "net/http/pprof" // Import for pprof side effects, not directly used in this file ) func main() { fmt.Println("Hello, world!") }
In this example, net/http/pprof
is imported solely for its side effects (it sets up profiling capabilities), and the underscore prevents an unused import warning.
Can I use the underscore _
to selectively import parts of a Go package?
No, the underscore _
in Go imports does not allow selective importing of parts of a package. It either imports the entire package (including its init()
function) or it doesn't import it at all. There's no mechanism to import only specific parts of a package while ignoring others using the underscore. If you only need specific parts of a package, you should import only those specific parts and avoid using the underscore. Using the underscore is specifically for situations where you need the package's initialization but don't directly use its exported identifiers within the current file. Selective importing is generally handled by importing only the necessary elements, potentially through renaming with the as
keyword.
The above is the detailed content of What is the function of underscore of import statements in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


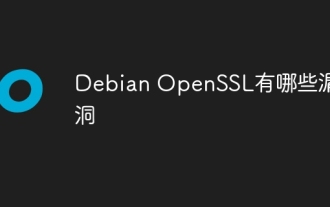
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
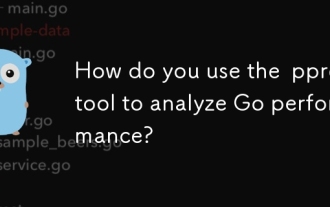
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
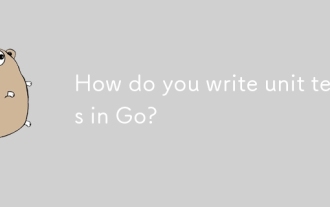
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
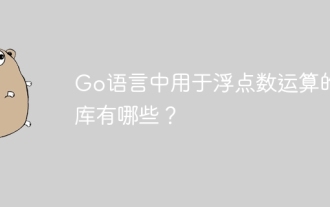
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
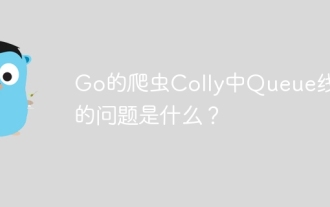
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
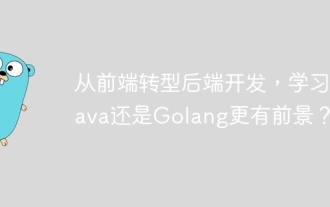
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
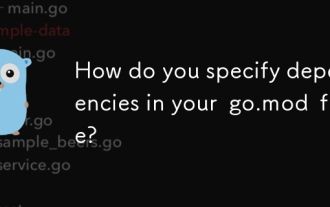
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
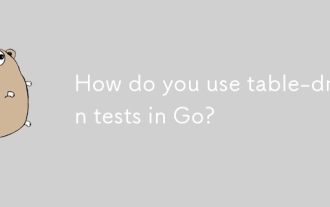
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
