How to deal with empty nodes when modifying XML content
XML Modification: How to Handle Empty Nodes?
Handling empty nodes in XML depends heavily on your definition of "empty." An empty node can refer to several scenarios:
-
A node with no children: This is a node with only attributes, or a node with no attributes and no children. This is generally not considered problematic and often represents valid XML structure. For instance,
<tag attribute="value"/>
is perfectly valid. - A node with only whitespace: A node containing only whitespace characters (spaces, tabs, newlines) is often considered empty. These nodes might be unintentionally introduced during XML creation or editing.
-
A node with empty text content: A node containing
<tag></tag>
or<tag> </tag>
(note the spaces). This is similar to the whitespace case but explicitly indicates an empty text content within the tags.
The approach to handling empty nodes depends on which of these definitions applies and your desired outcome. Ignoring them might be acceptable in some cases, while in others, you might need to remove them or replace them with a default value. The strategy should be determined by the specific requirements of your XML processing task.
How Can I Efficiently Remove Empty XML Nodes?
Efficiently removing empty XML nodes requires careful consideration of your data and chosen tools. Directly manipulating the XML document using string manipulation is generally inefficient and error-prone. Instead, leverage XML processing libraries that provide robust and optimized methods.
Here's a general approach, assuming "empty" means nodes with only whitespace or no content:
-
Use an XML parsing library: Libraries like
xml.etree.ElementTree
(Python),libxml2
(C), orlxml
(Python) offer DOM (Document Object Model) manipulation capabilities. These allow you to traverse the XML tree, identify empty nodes, and remove them efficiently. - XPath or XSLT (for more complex scenarios): For complex XML structures or large files, XPath expressions can help locate empty nodes precisely. XSLT (Extensible Stylesheet Language Transformations) allows you to transform the XML document, removing empty nodes as part of the transformation.
-
Iterative approach: Traverse the XML tree. For each node, check if its text content is only whitespace (using
strip()
in Python, for instance). If it is, remove the node using the library's provided functions (e.g.,node.remove()
inxml.etree.ElementTree
). Remember to handle potential exceptions during file processing.
Example (Python with xml.etree.ElementTree
):
import xml.etree.ElementTree as ET tree = ET.parse('input.xml') root = tree.getroot() for element in root.findall('.//*'): # Find all elements recursively if element.text is None or element.text.strip() == '': element.remove() tree.write('output.xml')
What Are the Best Practices for Handling Empty Nodes During XML Updates?
Best practices for handling empty nodes during XML updates focus on clarity, efficiency, and data integrity:
- Define "empty" explicitly: Clearly define what constitutes an "empty" node in your context. This avoids ambiguity and ensures consistent handling.
- Use appropriate tools: Employ XML processing libraries designed for efficient DOM manipulation, rather than manual string manipulation.
- Validate XML: Before and after updates, validate the XML against its schema (if available) to ensure well-formedness and validity. This helps prevent errors caused by incorrect node removal or modification.
- Backup your data: Always back up your XML data before performing any updates. This allows for easy recovery in case of errors.
- Error handling: Implement robust error handling to gracefully handle unexpected situations, such as malformed XML or missing nodes.
- Logging: Log significant events during XML processing, including the removal or modification of empty nodes. This helps in debugging and monitoring.
- Consider alternatives to removal: Instead of removing empty nodes, consider replacing them with default values or placeholder nodes, depending on the context and requirements. This can improve data consistency and prevent downstream processing issues.
What XML Tools or Libraries Are Best Suited for Managing Empty Nodes in XML Files?
Several tools and libraries excel at managing empty nodes in XML files. The best choice depends on your programming language and the complexity of your task:
-
Python:
xml.etree.ElementTree
(built-in, suitable for simpler tasks),lxml
(faster and more feature-rich, excellent for larger files and complex manipulations). -
Java:
javax.xml.parsers
(built-in),dom4j
,JDOM
. -
C :
libxml2
(a very powerful and widely used library). - JavaScript: Various libraries exist, including those that work with DOM manipulation directly in the browser.
- XSLT Processors: For complex transformations, XSLT processors (available in many languages) are ideal for selectively removing or modifying empty nodes based on rules defined in an XSLT stylesheet.
Choosing the right tool depends on your specific needs. For simple tasks, built-in libraries are sufficient. For large files, complex manipulations, or high-performance requirements, dedicated XML processing libraries are recommended. Consider factors like speed, ease of use, and the availability of features such as XPath support when making your selection.
The above is the detailed content of How to deal with empty nodes when modifying XML content. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


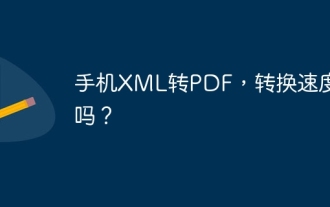
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
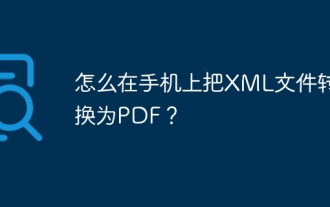
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
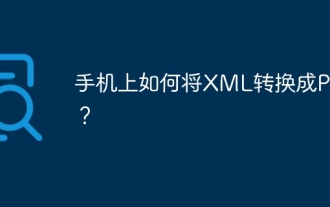
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.

To open a web.xml file, you can use the following methods: Use a text editor (such as Notepad or TextEdit) to edit commands using an integrated development environment (such as Eclipse or NetBeans) (Windows: notepad web.xml; Mac/Linux: open -a TextEdit web.xml)
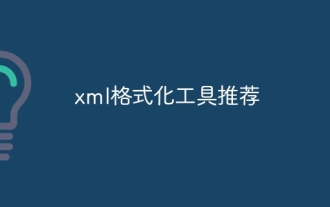
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
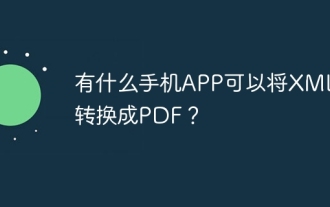
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
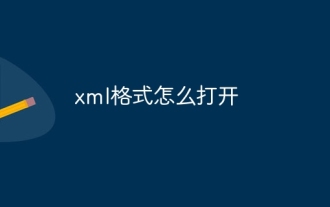
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
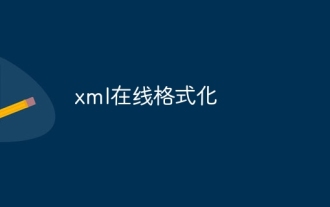
XML Online Format Tools automatically organizes messy XML code into easy-to-read and maintain formats. By parsing the syntax tree of XML and applying formatting rules, these tools optimize the structure of the code, enhancing its maintainability and teamwork efficiency.
