How to modify content using PHP in XML
Modifying XML Content with PHP
This article addresses common questions regarding using PHP to modify XML content, covering efficient techniques, suitable libraries, and crucial security considerations.
How to Use PHP to Modify XML Content
PHP offers several ways to modify XML content, primarily leveraging the DOMDocument
class. This class allows for a robust and flexible approach to parsing and manipulating XML structures. The process typically involves loading the XML file, finding the specific nodes you want to modify, making the changes, and then saving the updated XML.
Here's a basic example demonstrating how to change the value of a specific node:
<?php $xml = new DOMDocument(); $xml->load('data.xml'); // Load your XML file // Find the node you want to modify (e.g., using XPath) $xpath = new DOMXPath($xml); $node = $xpath->query('//item[@id="123"]/name')->item(0); // Selects the 'name' node within an 'item' node with id="123" //Check if the node exists if ($node !== null) { $node->nodeValue = 'New Name'; // Change the node value $xml->save('data.xml'); // Save the updated XML file } else { echo "Node not found"; } ?>
This code first loads the XML file using DOMDocument::load()
. Then, it utilizes DOMXPath
to locate the target node using an XPath expression. The nodeValue
property is then updated, and finally, DOMDocument::save()
writes the modified XML back to the file. Error handling (like checking if the node exists) is crucial to prevent unexpected behavior. Remember to replace 'data.xml'
and the XPath expression with your actual file path and target node selection.
How Can I Efficiently Update Specific XML Nodes Using PHP?
Efficiency in updating XML nodes hinges on several factors:
- XPath: Using XPath expressions allows for precise targeting of nodes without needing to traverse the entire XML tree. Well-crafted XPath queries significantly reduce processing time, especially with large XML files.
-
DOMDocument vs. SimpleXML: While
SimpleXML
is easier to use for simple modifications,DOMDocument
offers better performance and control for complex manipulations of large XML documents. For large-scale updates,DOMDocument
is generally preferred. - Caching: If you're performing repeated modifications on the same XML file, caching parts of the parsed XML structure can improve performance. This avoids redundant parsing.
-
Streaming: For extremely large XML files that don't fit in memory, consider using streaming techniques to process the XML incrementally. Libraries like
XMLReader
can be helpful in this scenario. - Database alternative: If you frequently update the same XML data, consider using a database instead. Databases are optimized for data manipulation and retrieval.
What PHP Libraries or Functions Are Best Suited for Parsing and Modifying XML Data?
The most commonly used and recommended PHP libraries for handling XML are:
-
DOMDocument
: This is the most powerful and flexible option. It provides full control over the XML structure and allows for complex manipulations. It's ideal for scenarios requiring precise node selection and modification. -
SimpleXML
: This offers a simpler, more intuitive interface for basic XML parsing and modification. It's suitable for smaller XML files and less complex operations. However, it lacks the fine-grained control ofDOMDocument
. -
XMLReader
: This is best suited for processing very large XML files that might not fit into memory. It allows for streaming XML data, processing it piece by piece.
Are There Any Security Considerations When Using PHP to Modify XML Files, Especially With User-Supplied Data?
Yes, security is paramount when handling user-supplied data within XML modifications. Failing to properly sanitize and validate input can lead to serious vulnerabilities like:
-
XML External Entity (XXE) Injection: Maliciously crafted XML input can exploit XXE vulnerabilities, allowing attackers to access local files or network resources. Disabling external entity processing in
DOMDocument
using$xml->resolveExternals = false;
is crucial. - Cross-Site Scripting (XSS): If user-supplied data is directly incorporated into the XML without proper escaping, it could lead to XSS vulnerabilities. Always sanitize user input before including it in the XML.
- File Path Manipulation: If users can influence the file path of the XML being modified, they might attempt to access or modify unintended files. Always validate and sanitize any file paths derived from user input.
- Denial of Service (DoS): Large or malformed XML input could lead to resource exhaustion and a denial-of-service attack. Implement input validation and size limits to prevent this.
In summary, while PHP provides excellent tools for XML manipulation, always prioritize security by thoroughly validating and sanitizing user input and implementing safeguards against potential vulnerabilities. Remember to choose the appropriate library (DOMDocument
, SimpleXML
, or XMLReader
) based on the complexity and size of your XML data.
The above is the detailed content of How to modify content using PHP in XML. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


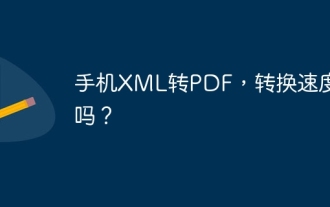
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
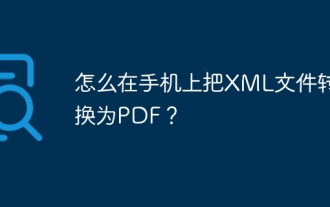
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
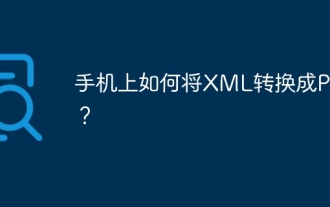
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.

To open a web.xml file, you can use the following methods: Use a text editor (such as Notepad or TextEdit) to edit commands using an integrated development environment (such as Eclipse or NetBeans) (Windows: notepad web.xml; Mac/Linux: open -a TextEdit web.xml)
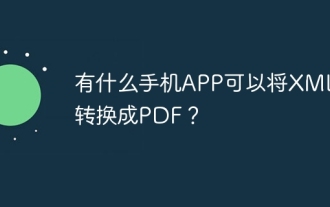
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
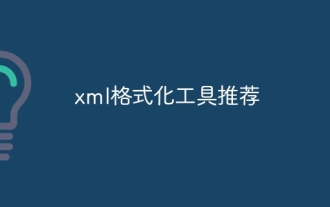
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
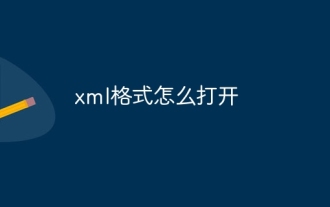
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
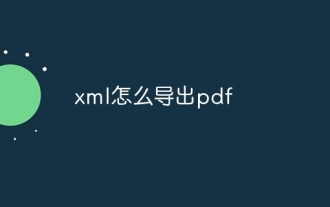
There are two ways to export XML to PDF: using XSLT and using XML data binding libraries. XSLT: Create an XSLT stylesheet, specify the PDF format to convert XML data using the XSLT processor. XML Data binding library: Import XML Data binding library Create PDF Document object loading XML data export PDF files. Which method is better for PDF files depends on the requirements. XSLT provides flexibility, while the data binding library is simple to implement; for simple conversions, the data binding library is better, and for complex conversions, XSLT is more suitable.
