


What are the types of values returned by c language functions? What determines the return value?
What are the different return types available for functions in C?
C offers a wide variety of return types for functions, allowing for flexibility in how functions communicate results. These types broadly fall into several categories:
-
Basic Types: These are the fundamental data types in C. They include:
-
void
: Indicates that the function does not return any value. -
int
: Represents an integer value. -
char
: Represents a single character. -
float
: Represents a single-precision floating-point number. -
double
: Represents a double-precision floating-point number. -
short
,long
,long long
: Variations of integer types with different sizes. -
unsigned int
,unsigned char
, etc.: Unsigned versions of integer types, allowing for only non-negative values.
-
-
Derived Types: These types are built upon the basic types:
-
arrays
: Functions can return arrays (although this is often achieved by returning a pointer to the first element of the array). Note that returning a local array directly is generally unsafe, leading to undefined behavior. -
pointers
: Pointers can point to any data type, allowing functions to return addresses of variables or data structures. -
structures
: Functions can return structures, enabling the return of multiple related data items as a single unit. -
unions
: Similar to structures, but all members share the same memory location. Returning a union is possible but requires careful consideration of the size and alignment of members. -
enums
: Functions can return enumerated types, representing a set of named integer constants.
-
-
Void Pointers:
void *
is a generic pointer type that can point to any data type. While flexible, it requires explicit casting when dereferenced, increasing the risk of errors.
The choice of return type depends heavily on the function's purpose and the nature of the data it needs to convey back to the calling function.
How does the compiler determine the return type of a C function?
The compiler determines the return type of a C function based on the return
statement within the function's body and the function's declaration.
- Function Declaration: The function declaration explicitly states the return type. This declaration is crucial because it informs the compiler how to interpret the function's return value. For example:
int add(int a, int b); // Declaration: Tells the compiler the function returns an int
- Return Statement: The
return
statement specifies the value returned by the function. The type of the expression within thereturn
statement must be compatible with the declared return type. If a function is declared to returnint
, thereturn
statement must return an integer value. If there's a mismatch, the compiler will issue an error. For example:
int add(int a, int b) { return a + b; // Returns an integer, matching the declaration }
-
Void Functions: If a function is declared with a
void
return type, it shouldn't have areturn
statement with a value. Areturn;
statement (without a value) is permitted in avoid
function to explicitly indicate the function's end.
What are the implications of choosing a specific return type for a C function?
The choice of return type has significant implications for:
- Data Integrity: An incorrect return type can lead to data corruption or unexpected behavior. If a function is expected to return an integer but returns a floating-point number, the integer portion might be truncated, leading to inaccurate results.
- Memory Management: Returning large data structures or arrays can impact memory usage and performance. Returning pointers to dynamically allocated memory requires careful handling to prevent memory leaks. The caller is responsible for freeing the memory allocated by the function.
- Error Handling: The return type can be used to indicate success or failure. For example, a function might return 0 for success and a non-zero value to represent different error codes.
- Code Readability: Choosing clear and descriptive return types improves code readability and maintainability. A well-chosen return type makes the function's purpose and output more obvious.
- Function Interface: The return type is a critical part of the function's interface, defining how the function interacts with other parts of the program. Changes to the return type require updating all parts of the code that use the function.
C language function return value types? Return value is determined by what?
The C language supports various return value types as detailed in the first answer. These include basic types (like int
, float
, char
), derived types (like pointers, arrays, structures), and void
(for functions that don't return a value).
The return value of a C function is determined by two key factors:
- The function's declaration: The function declaration explicitly specifies the return type. This declaration acts as a contract, defining what kind of value the function is expected to produce.
-
The
return
statement within the function body: Thereturn
statement determines the actual value returned by the function. The type and value of the expression within thereturn
statement must be compatible with the declared return type. The compiler will check for this compatibility during compilation. If a function is declared to returnint
but thereturn
statement tries to return adouble
, the compiler will report an error.
The above is the detailed content of What are the types of values returned by c language functions? What determines the return value?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










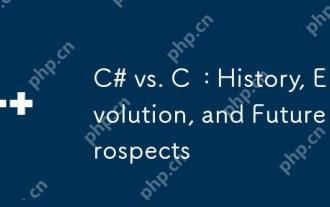
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
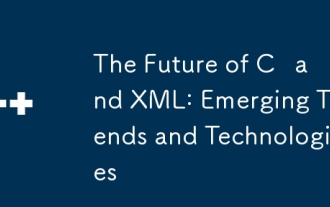
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
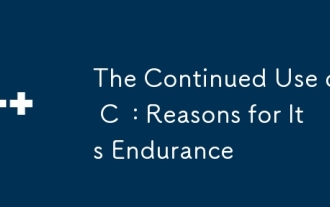
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
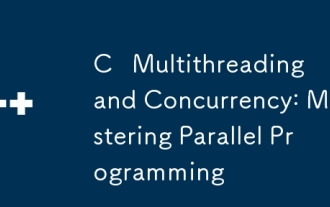
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
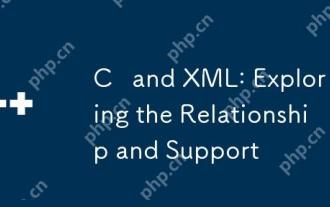
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
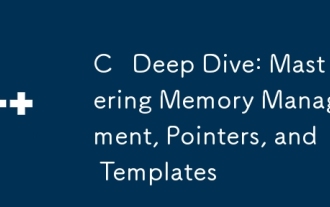
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
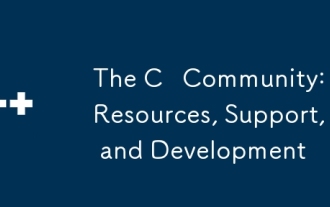
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
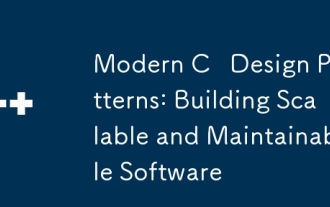
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
